Browse the Full Catalog
Cybrary has the fastest growing, fastest moving catalog - We publish new content weekly! Learn at your own pace - with flexible learning, you can build your skills whenever, wherever.
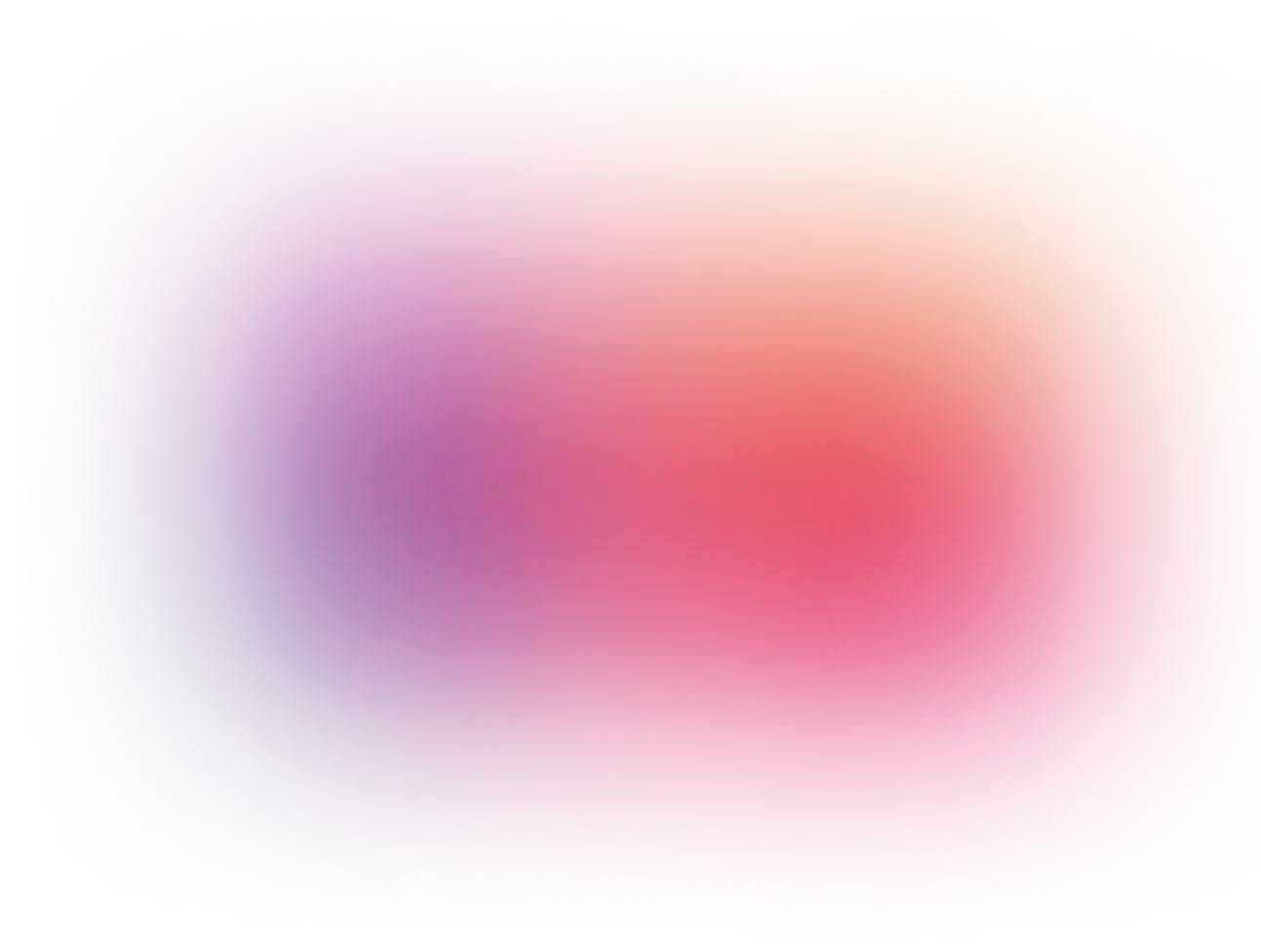
The content and tools you need to build real-world skills
Rapidly develop your skills via an integrated, and engaging learning
experience on the Cybrary platform.
On-Demand Video Training
Expert instructed from industry leaders
Virtual Labs
Hands on experience to apply and reinforce newly learned skills
Practice Exams
Prepare for industry certifications
Assessments
Gauge your skill development and see real-time progress
Thank you! Your submission has been received!
Oops! Something went wrong while submitting the form.
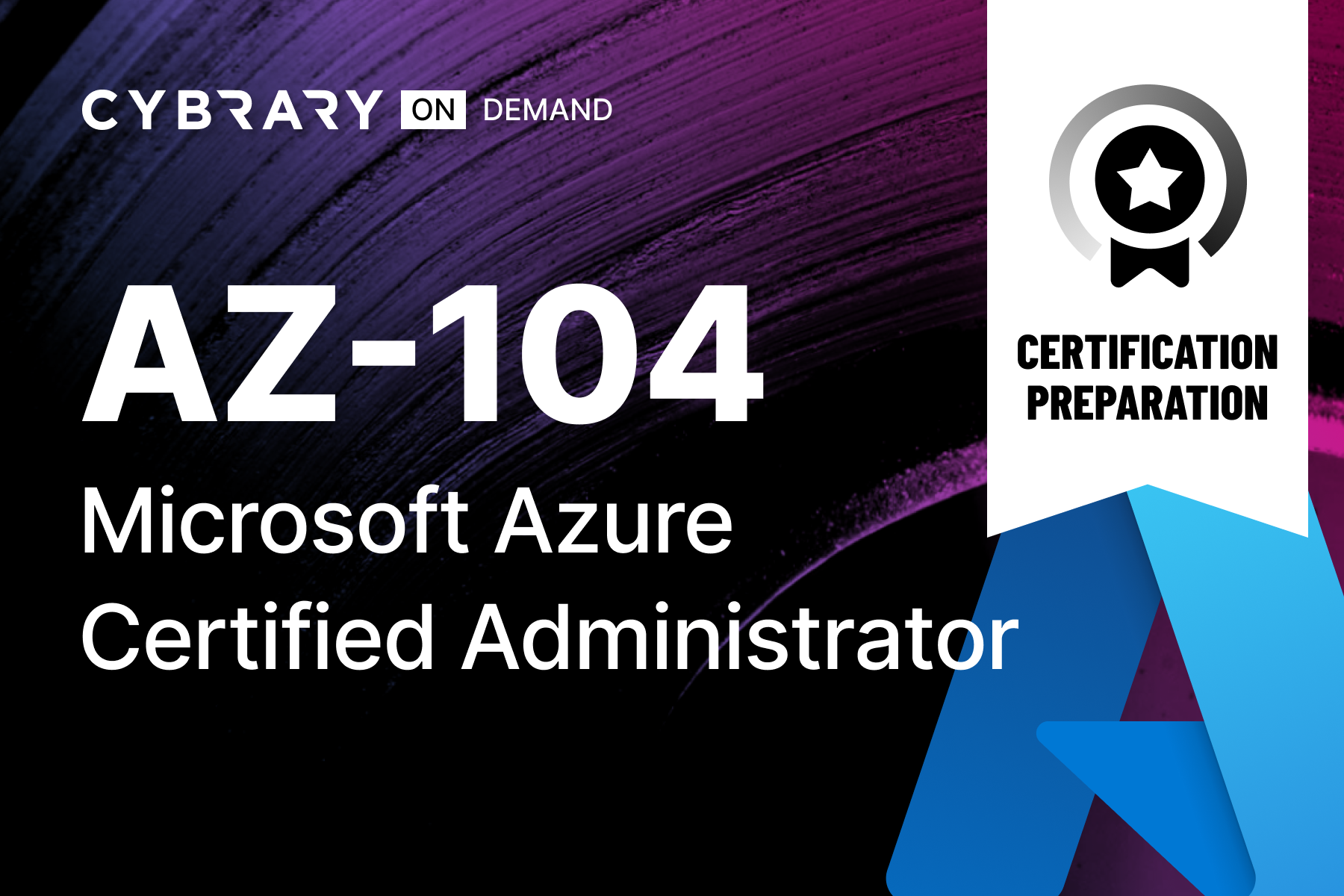
AZ-104 Microsoft Azure Administrator
Looking to enhance your experience with Azure administrative cloud technologies and solutions? This Microsoft Azure AZ-104 Certification training course will help you pass the exam and confidently administer basic Azure solutions such as web servers, networking solutions, storage solutions and cloud environment monitoring.
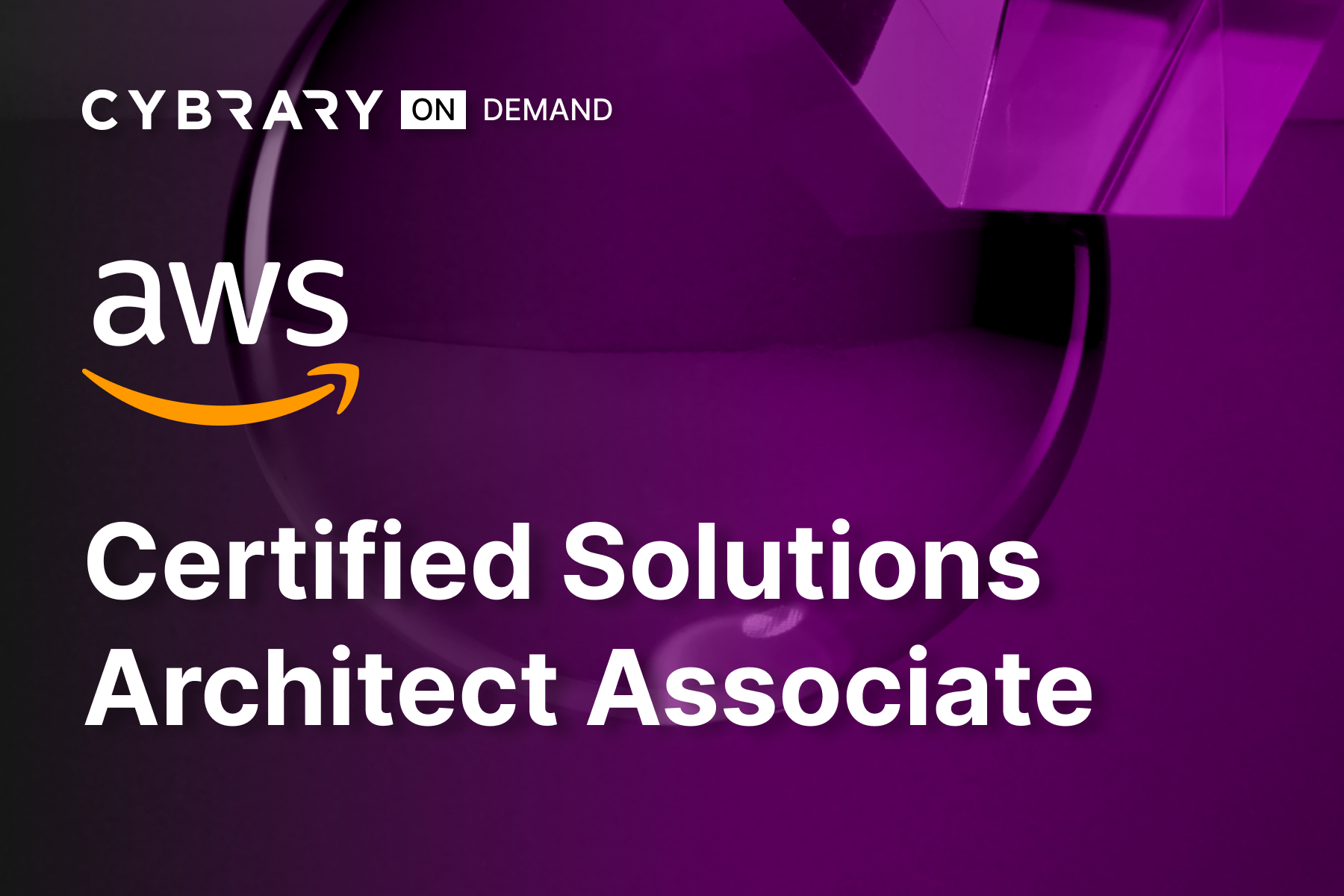
AWS Certified Solutions Architect Associate (SAA-C03)
Ready to design and implement distributed systems on AWS? This AWS Solutions Architect Associate training course will prepare you to pass the associate-level certification exam. Gain hands-on experience with configuring AWS secure architecture, creating a custom VPC, and more.
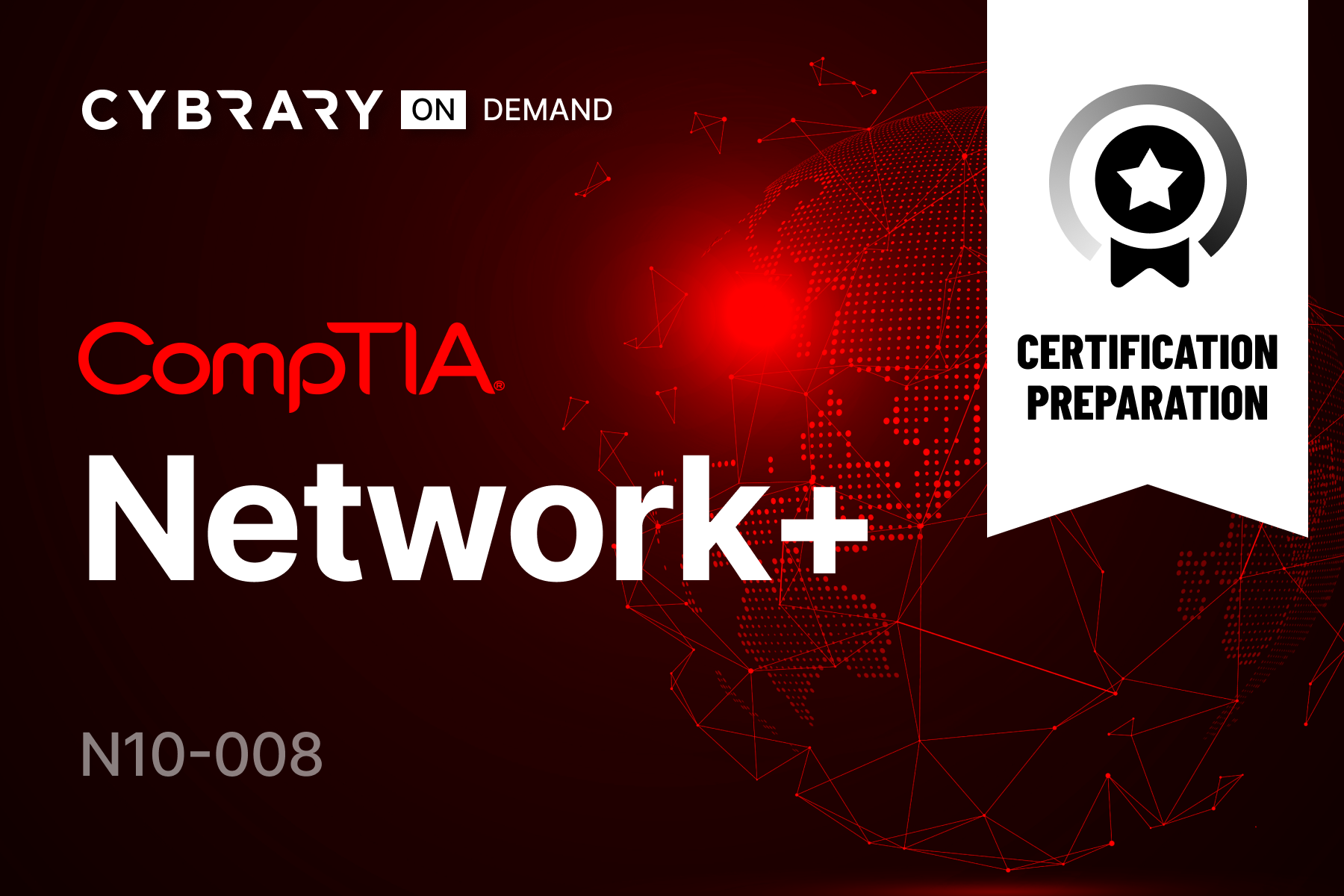
CompTIA Network+ (N10-008)
Eager to learn basic networking concepts and pass the CompTIA Network+ (N10-008) exam? Cybrary’s Network+ training course provides you with the knowledge to prepare for the exam and perform entry-level networking tasks such as troubleshooting, routing, and intrusion detection.

AWS Certified Cloud Practitioner
Learn the foundational principles of Amazon Web Services (AWS), the world’s most comprehensive and broadly adopted cloud platform. This AWS Cloud Practitioner training not only gives you a solid understanding of how to implement AWS cloud solutions but also prepares you to pass the current related certification exam.
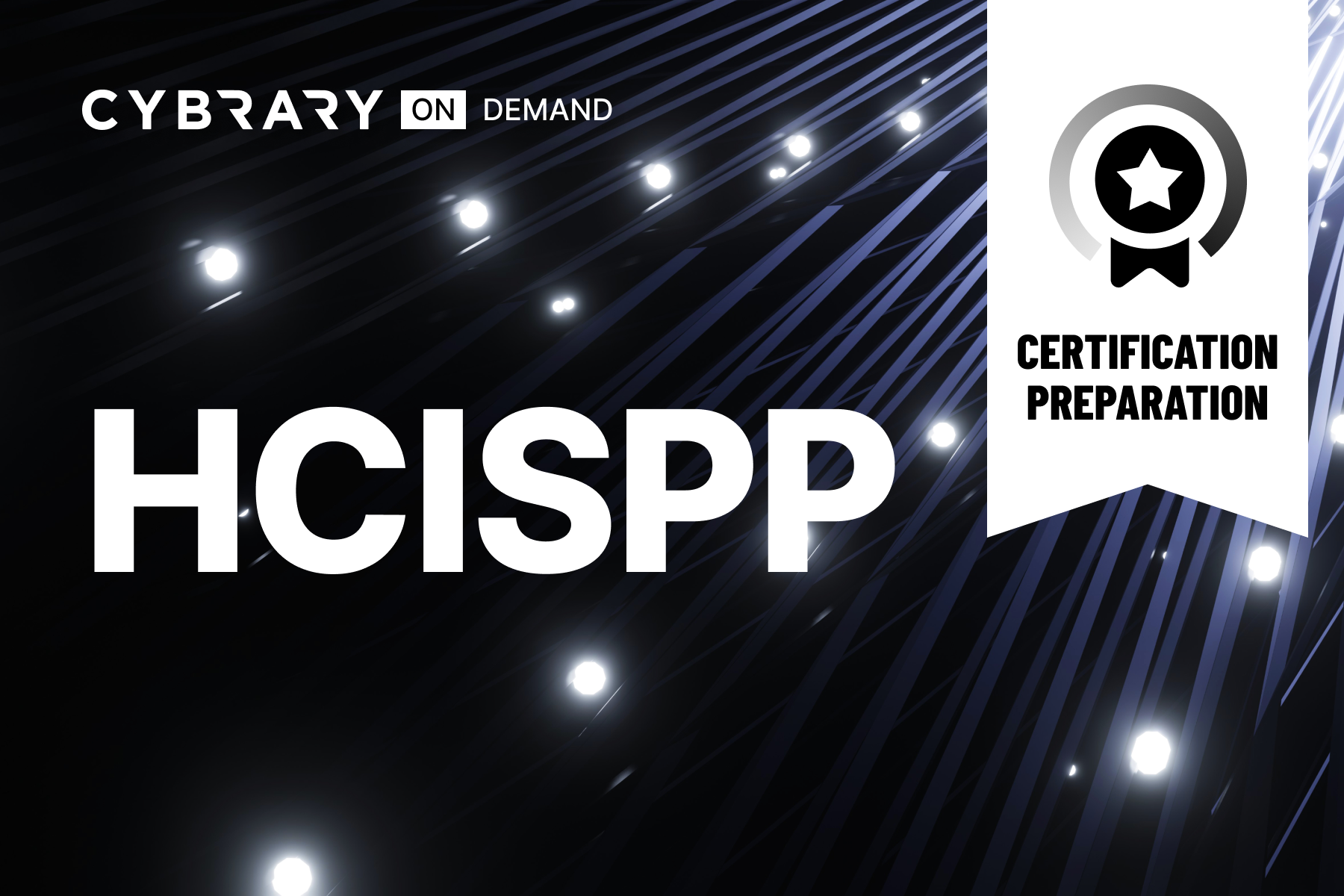
HCISPP
As our healthcare industry grows, so do the risks associated with keeping health information secure. The HCISPP certification is ideal for security professionals responsible for safeguarding protected health information (PHI). Take this HCISPP training course to prepare to manage and implement security controls for healthcare information.
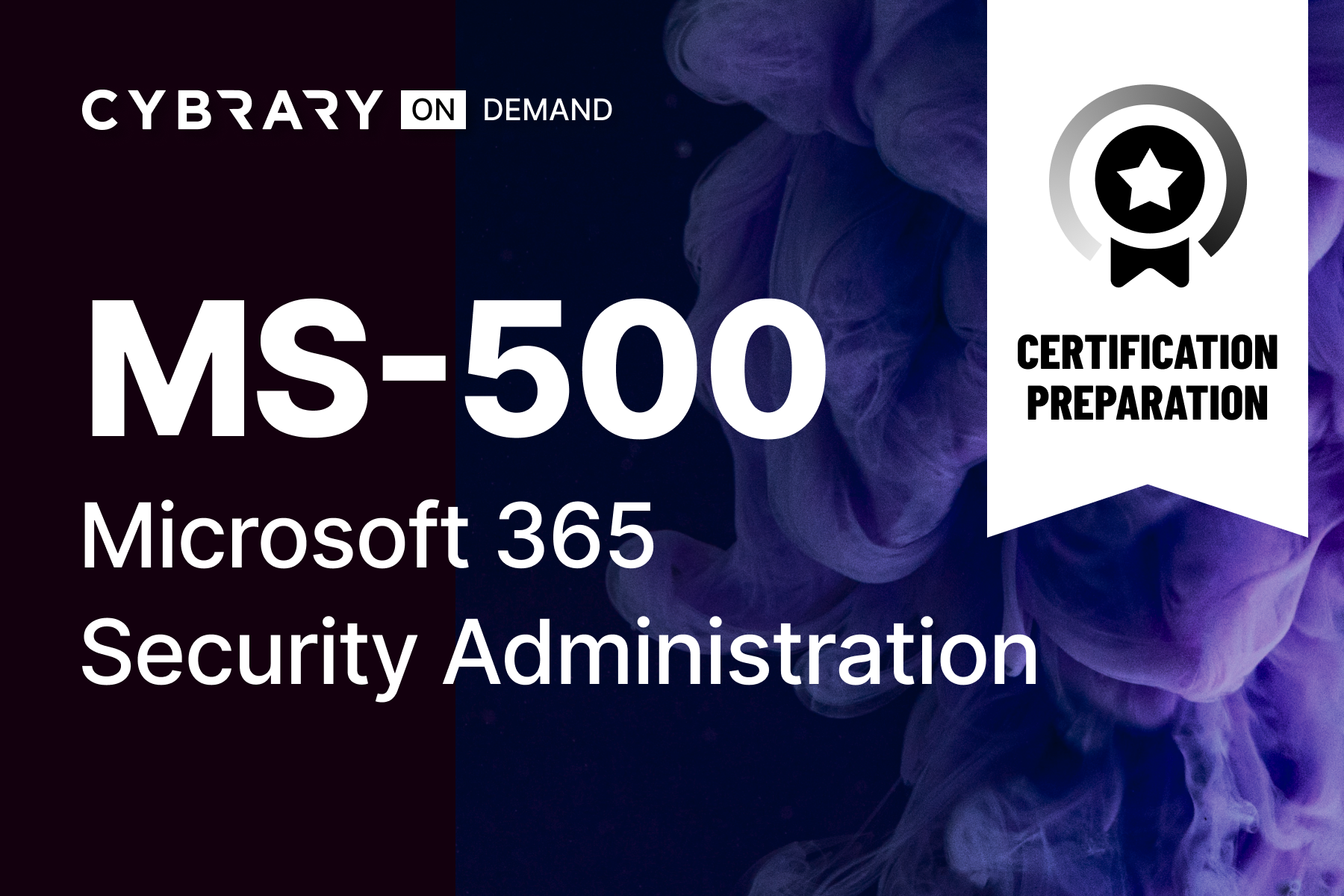
Microsoft 365 Security Administration (MS-500)
Are you a system administrator who wants to get a certification that is globally recognized and shows your commitment to staying current with Microsoft technologies and security best practices? Take this MS-500 training course and prepare to slay the exam with the knowledge and skills employers are looking for.
Thank you! Your submission has been received!
Oops! Something went wrong while submitting the form.
Popular
Foundations
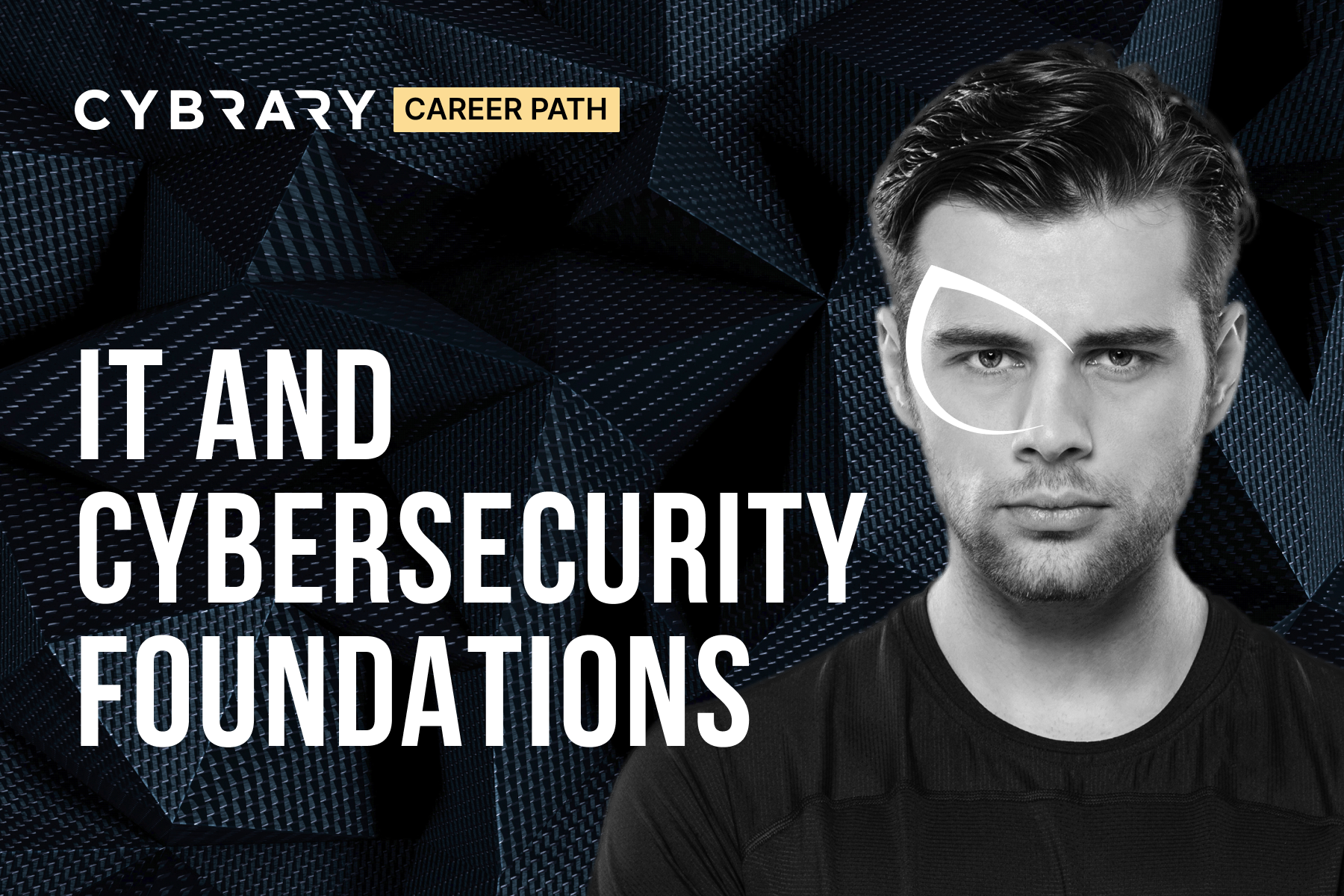
IT and Cybersecurity Foundations
Get everything you need to start your cybersecurity career journey, and stop wasting your time (and money) sorting through unreliable information from questionable sources.
While this industry offers very lucrative career opportunities, finding accurate, relevant information to break into the field can be incredibly frustrating. That’s why we’ve crafted this IT and Cybersecurity Foundations path, featuring thoughtful, bite-sized content from expert instructors covering core concepts found across all cybersecurity roles.
These instructors have helped thousands of other beginners grasp essential IT and cybersecurity topics. Get ready to take your first steps into cybersecurity by diving into core concepts needed for any cybersecurity role. Build practical skills and gain confidence as you begin your cybersecurity journey.
Risk for Managers
Cyber Defense Forensics Analyst
Cyber Defense Incident Responder
Technical Support Specialist
Defensive Security
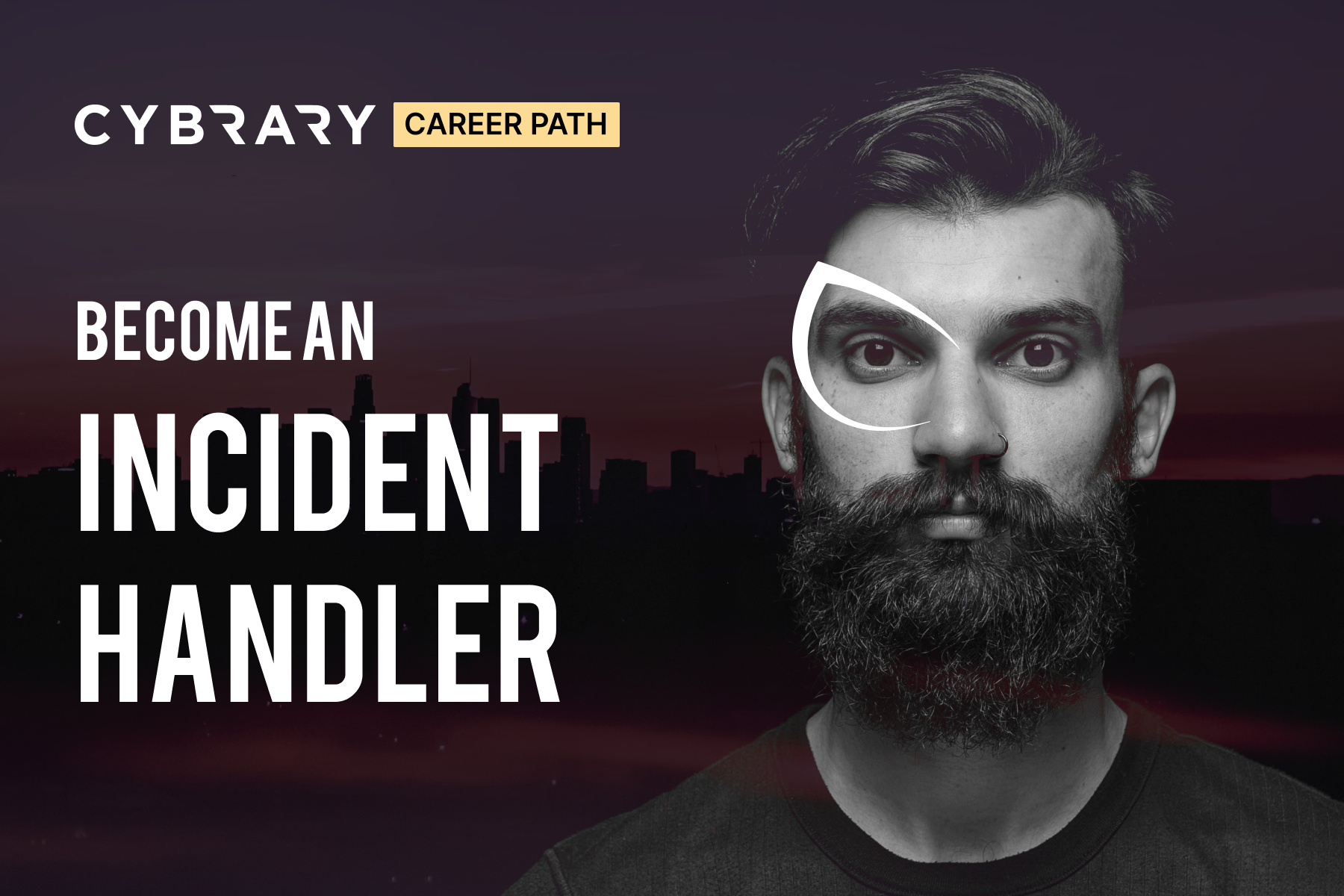
Become an Incident Handler
In this Career Path, you will learn the incident response process, from building an incident response kit and developing an incident response team, to identifying, containing, and recovering from incidents. We then steer away from a traditional “defensive-only” approach to introduce you to the attacker’s world.
Exploitation Analyst
Vulnerability Assessment Analyst
Offensive Security
Career Path
Practitioner Popular
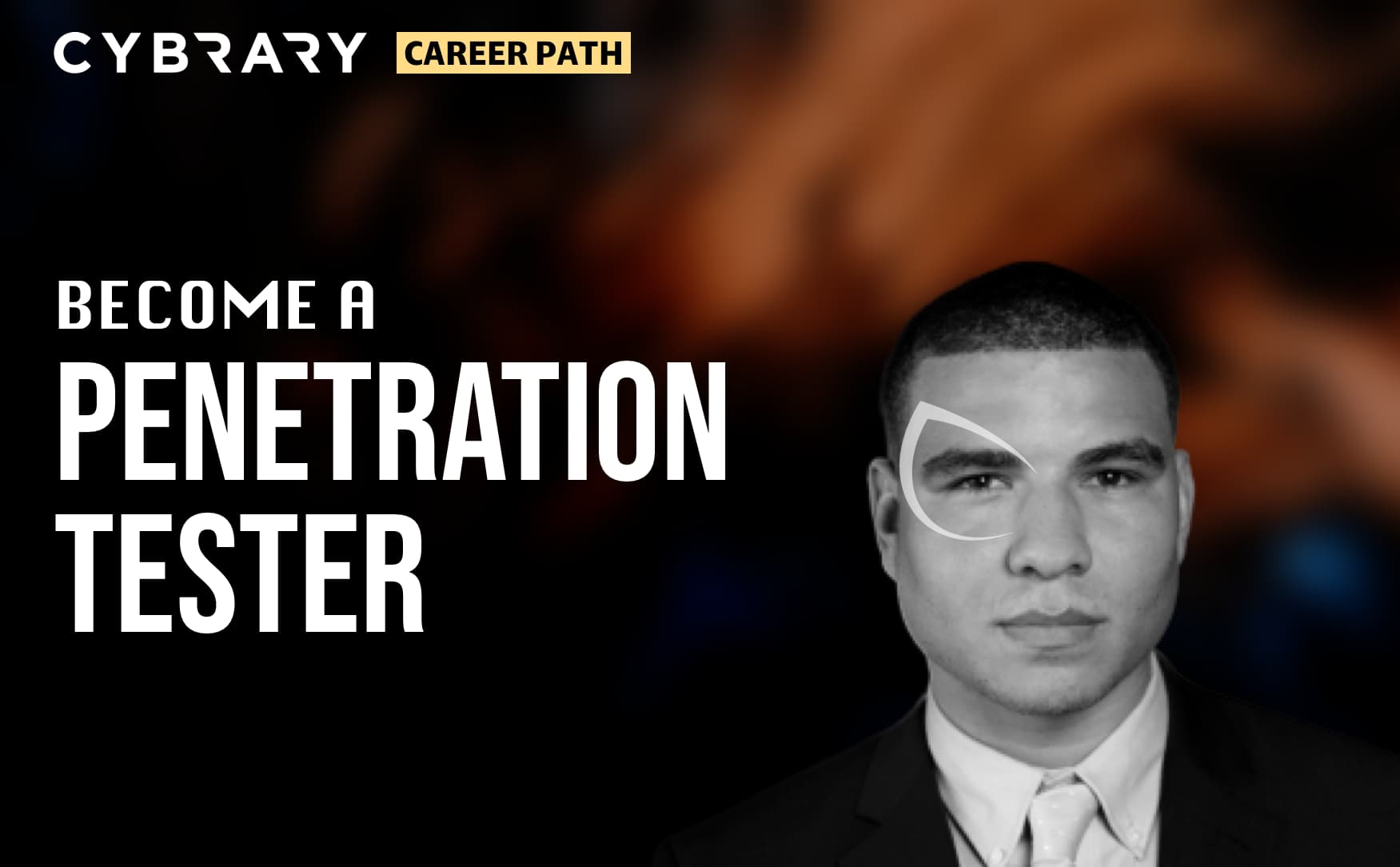
Become a Penetration Tester
Last year, penetration testers ranked as one of the three most in-demand jobs in the growing cybersecurity job market. To become a penetration tester, a college degree is not necessary since it’s a skills-based profession. Employers want professionals who can demonstrate the required skills, regardless of education. Cybrary offers realistic, virtual lab environments where you will gain real-world, hands-on skills you will use as a penetration tester.
CompTIA
MITRE Engenuity
Cyber Defense Analyst
Cyber Defense Incident Responder
Executive Cyber Leadership
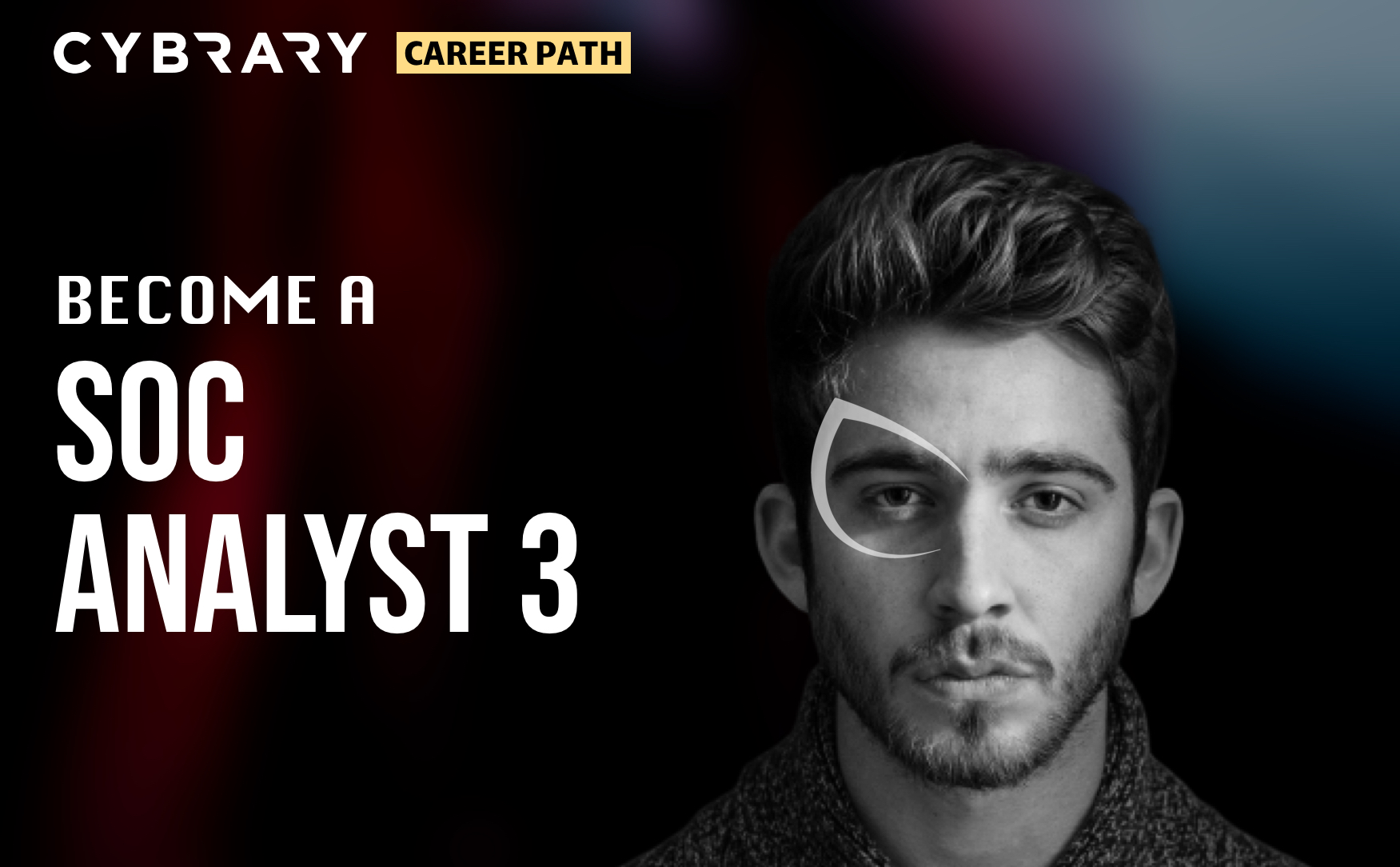
Become a SOC Analyst - Level 3
This Career Path is for a Security Operations Center Analyst (SOC Analyst). This particular Career Path covers a more advanced-level SOC role. As a SOC Analyst, your primary duty is to ensure that the organization’s digital assets are secure and protected from unauthorized access. That means that you are responsible for protecting both online and on-premise infrastructures, monitoring data to identify suspicious activity, and identifying and mitigating risks before there is a breach. In the event that a breach does occur, a SOC analyst will be on the front line, working to counter the attack.
Cyber Defense Analyst
Cyber Defense Incident Responder
Defensive Security
Career Path
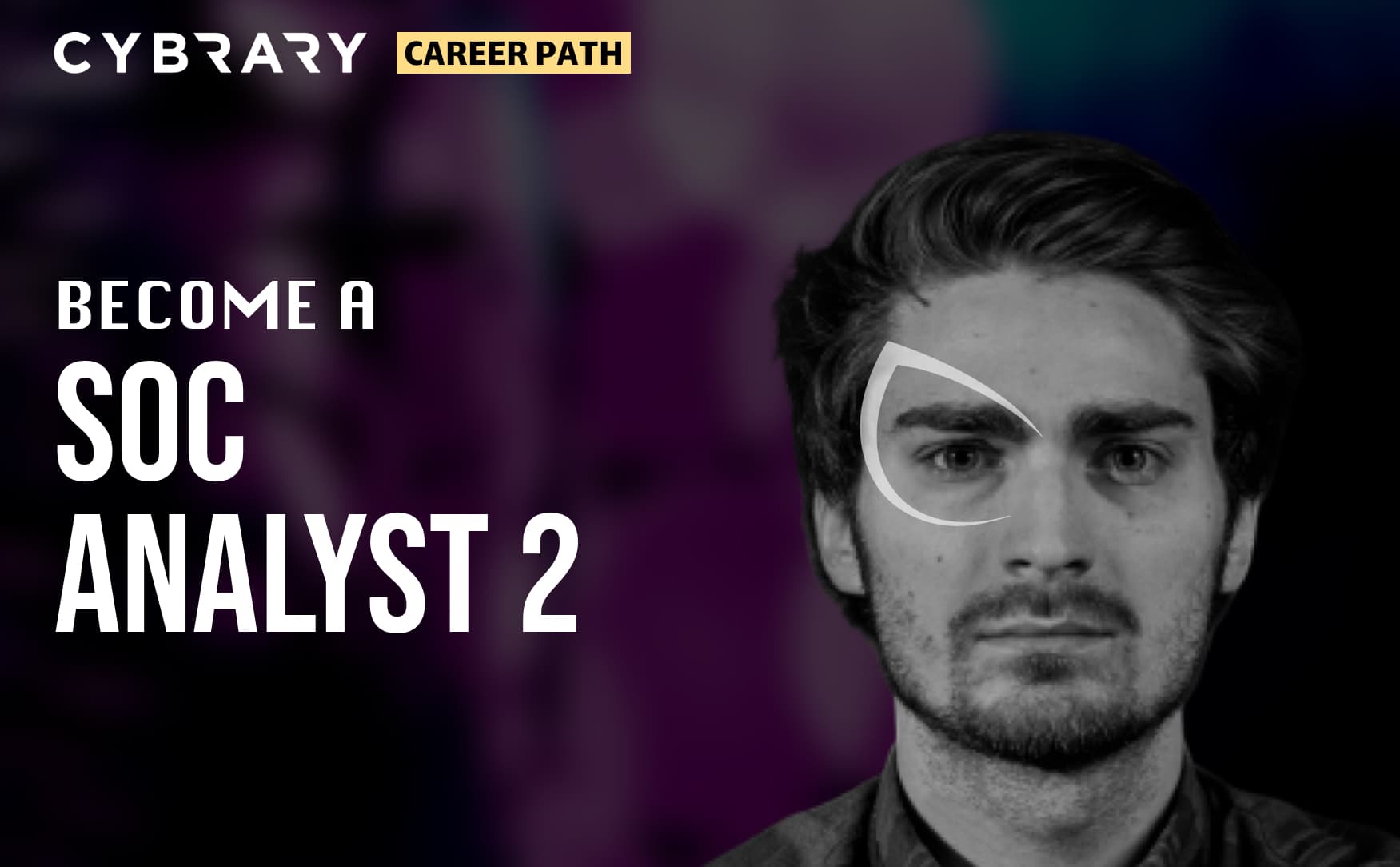
Become a SOC Analyst - Level 2
This Career Path is for a Security Operations Center Analyst (SOC Analyst). This particular Career Path covers a more intermediate-level SOC role. As a SOC Analyst, your primary duty is to ensure that the organization’s digital assets are secure and protected from unauthorized access. That means that you are responsible for protecting both online and on-premise infrastructures, monitoring data to identify suspicious activity, and identifying and mitigating risks before there is a breach. In the event that a breach does occur, a SOC analyst will be on the front line, working to counter the attack. This career path is aligned to the Cyber Defense Incident Responder NICE/NIST Work Role.
Cisco
Network Operations Specialist
Target Network Analyst
Career Path
Entry Popular
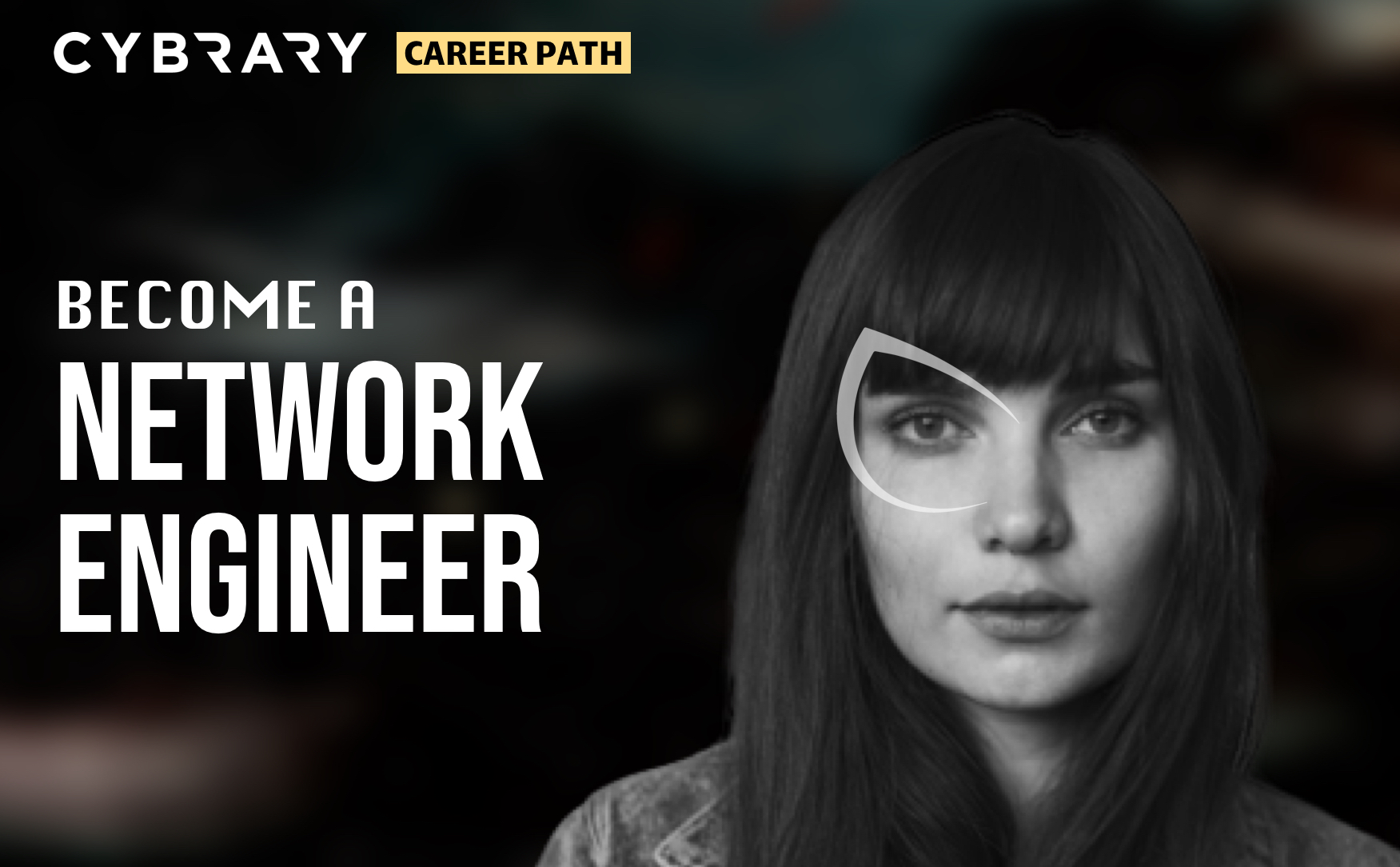
Become a Network Engineer
You have chosen a fantastic career in network engineering. More than 8 million network engineering jobs exist and it’s growing. On this career path, you will learn how to protect, administrate, and build best-in-class networks. Master management of systems performance, troubleshoot and diagnose network issues, understand infrastructure protocols, application and transport to empower organizations to scale securely. Cybrary’s trademark virtual lab environments provide students with real-world, hands-on skills and provide experience with today’s latest tools and technologies.
In our community, you will be guided by the top experts in network engineering. We have more than 40 mentors, including our Chief Mentor Mark Nibert, who manages a network operations center for the US government. You will be learning with more than ten thousand community members that are all building their careers in the cybersecurity and technology space. As you grow your career, you will now have a network of people that will help you continuously.
Cyber Defense Analyst
Security Architect
Certified Cloud Security Professional (CCSP)
Certified Information Systems Security Professional (CISSP)
SC-200: Microsoft Security Operations Analyst
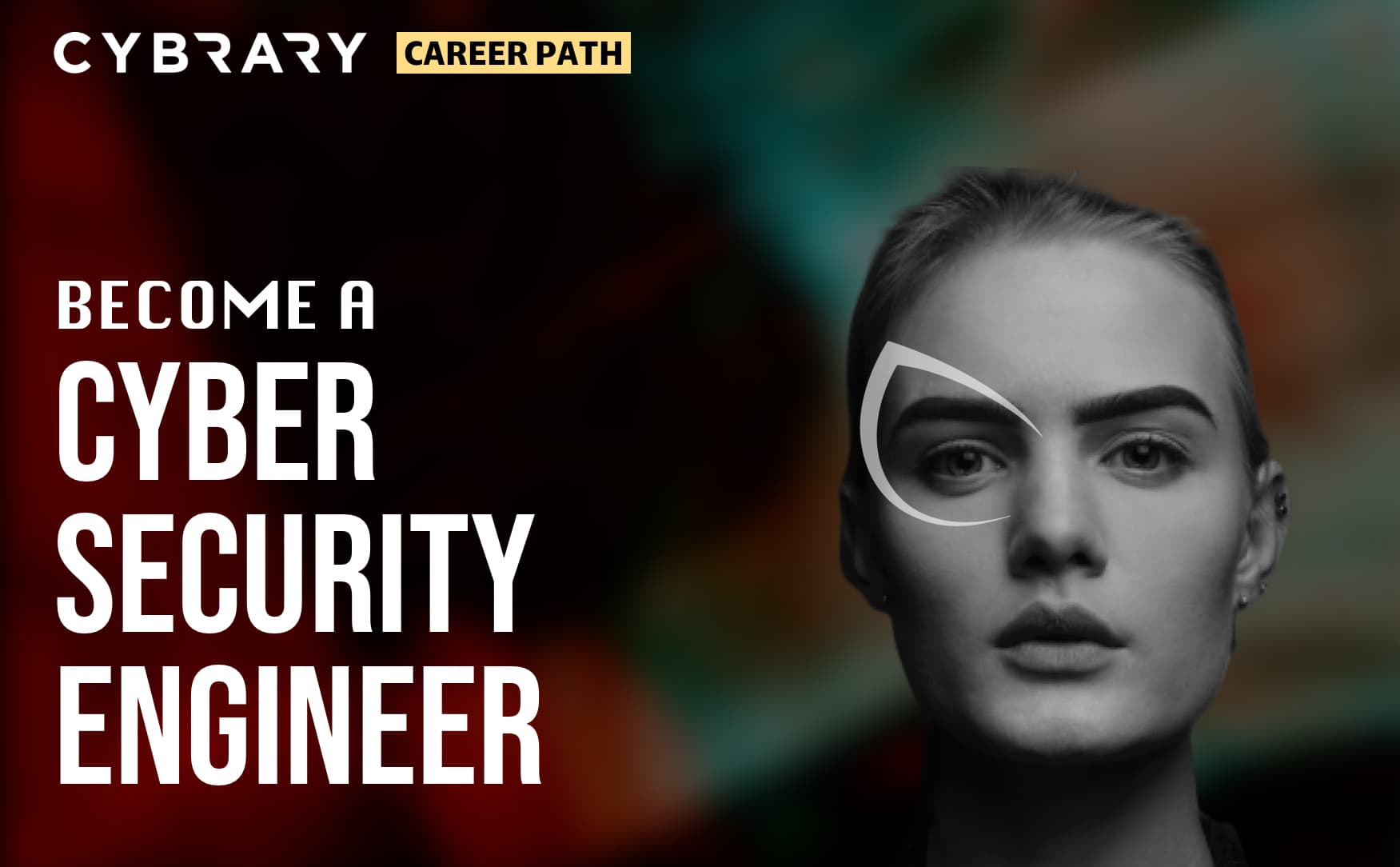
Become a Cyber Security Engineer
In this career path, you will learn what it takes to become a Security Engineer, a role in the highest demand with 57,000+ job openings according to cyberseek.org. Learn to resolve production security issues, configure and manage firewalls and intrusion detection systems (IDS), investigate incidents, perform forensics and run incident responses.
Cybrary offers practical, virtual lab environments specific to Cyber Security Engineering that will help you gain real-world, hands-on skills with the industry’s latest tools and technologies.
Microsoft Azure
Cloud
Cloud Fundamentals
Microsoft Azure Administrator (AZ-104)
Microsoft Azure Architect Design (AZ-304)
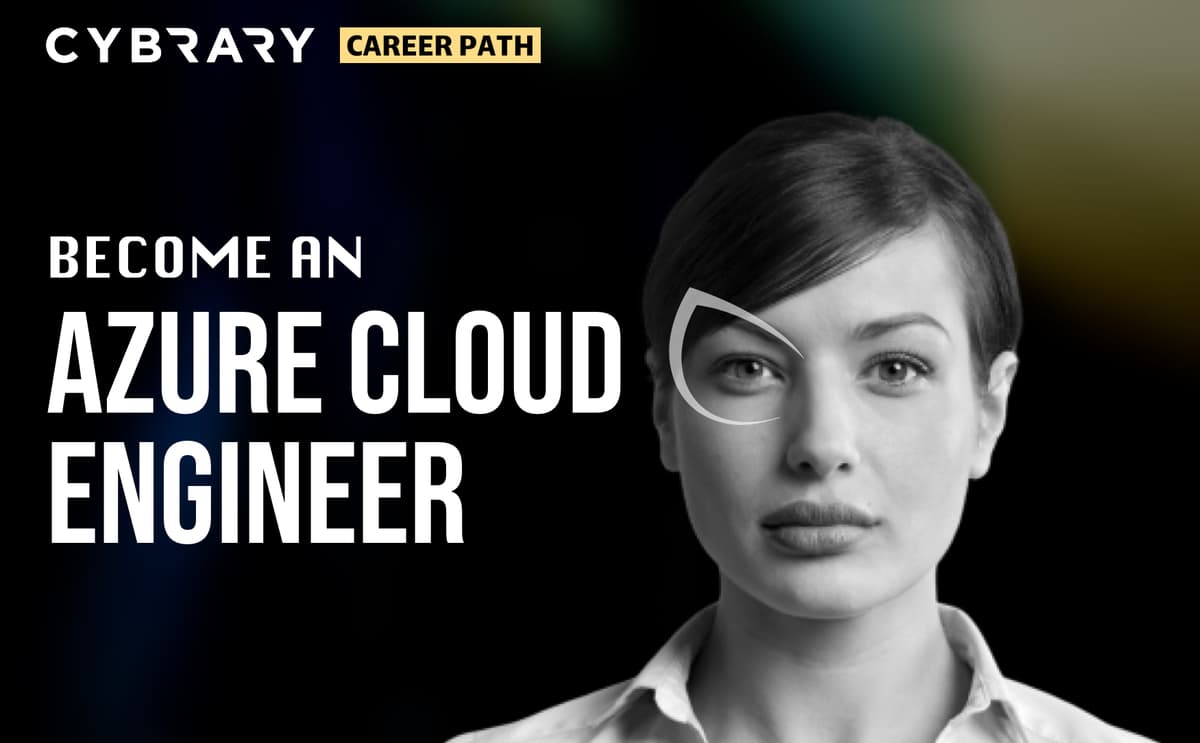
Become an Azure Cloud Engineer
This Azure Career Path provides a structured curriculum with specialized learning activities that will give you real-world training to become a successful cloud engineer in Microsoft Azure. In this career path, you will learn about cloud computing, cloud infrastructure, networking services, cloud security, virtual machines, and more while preparing you for multiple certifications like the Microsoft Azure AZ-900, Microsoft AZ-104, and Microsoft AZ-500.
Once you enroll in Cybrary’s Microsoft Azure Cloud Engineer career path, you will be learning alongside ten thousand community members who are all building their career in the cybersecurity, IT, and cloud space. As you grow your career you will now have a network of people through Cybrary who will help you continuously.
Authorizing Official/Designating Representative
Enterprise Architect
Executive Cyber Leadership
Security Architect
Leadership and Management
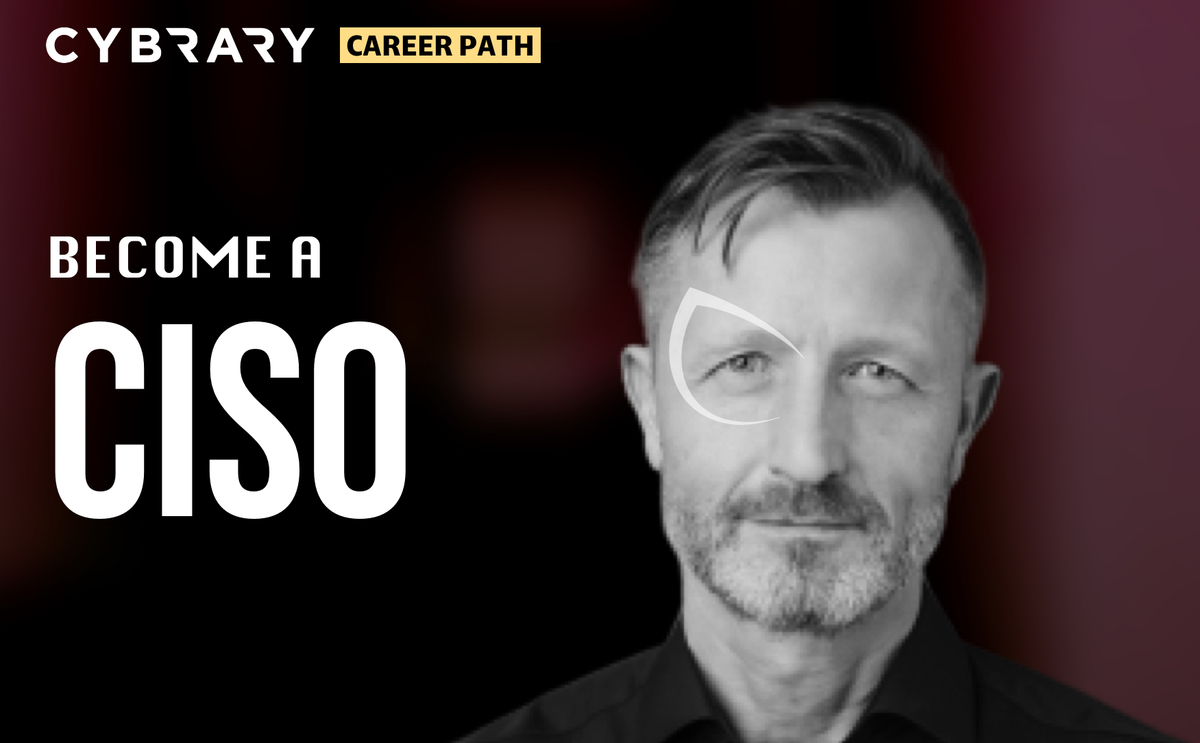
Become a CISO
Taught by CISOs for CISOs, this Career Path will provide you with a structured curriculum with specialized learning activities that will give you real-world training on how to become a successful CISO in the ever-changing security field. You will learn about corporate cybersecurity management, NIST 800-53 security and privacy controls, business continuity and disaster planning, enterprise security case management, and numerous competencies of the effective CISO.
Thank you! Your submission has been received!
Oops! Something went wrong while submitting the form.

Defensive Security and Cyber Risk
In this course, you will learn the basics of defensive security and cyber risk. You will review foundational risk management concepts such as calculating risk and strategies for dealing with risk. You will also explore the NIST CSF as a framework for understanding defensive security.
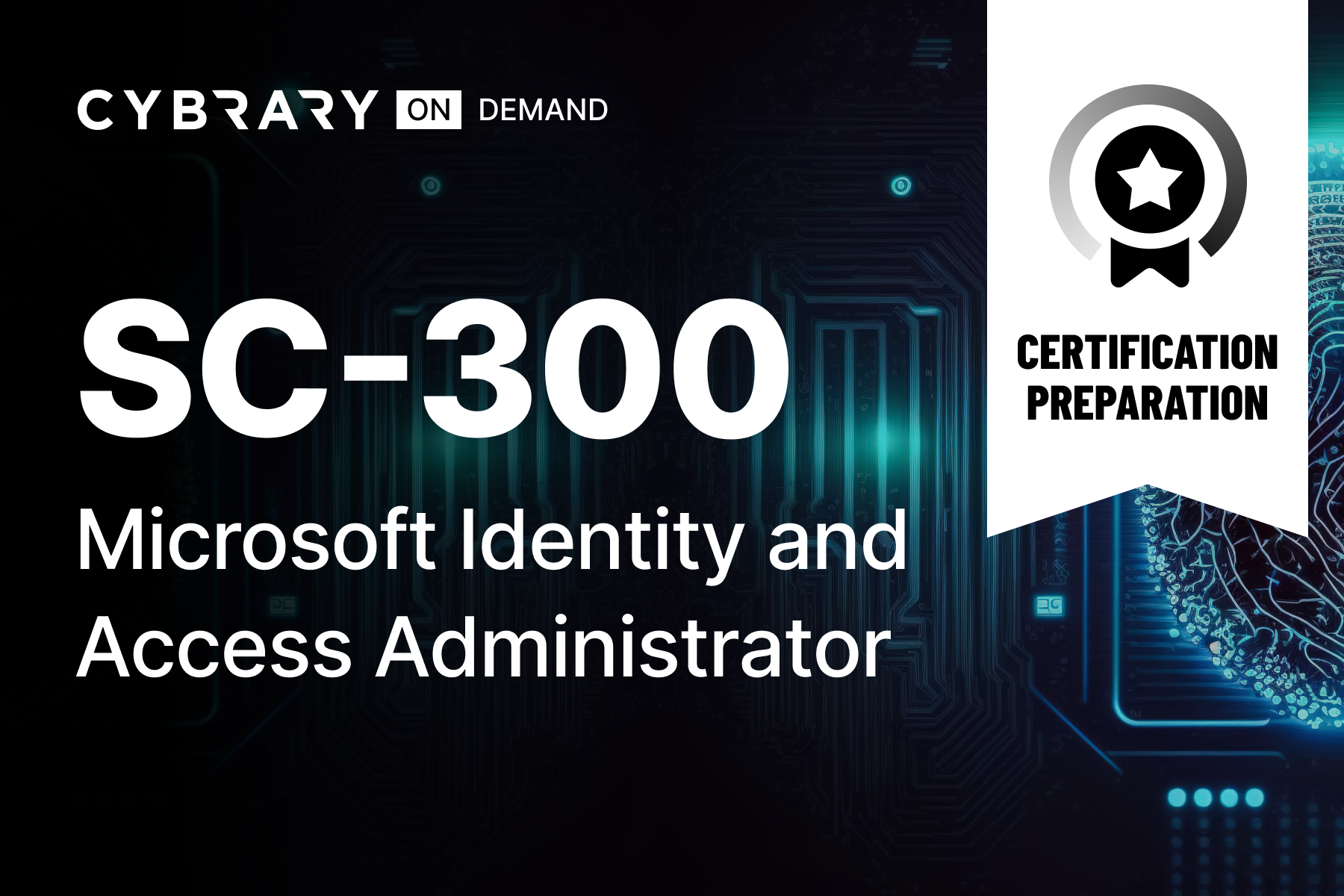
SC-300 Microsoft Identity and Access Administrator
Learn to design, implement, and operate your organization’s identity and access management (IAM) systems by using Microsoft Azure Active Directory, part of Microsoft Entra. As an IAM admin, you'll collaborate with many other roles to drive strategic identity projects, modernize identity solutions, and implement identity governance.
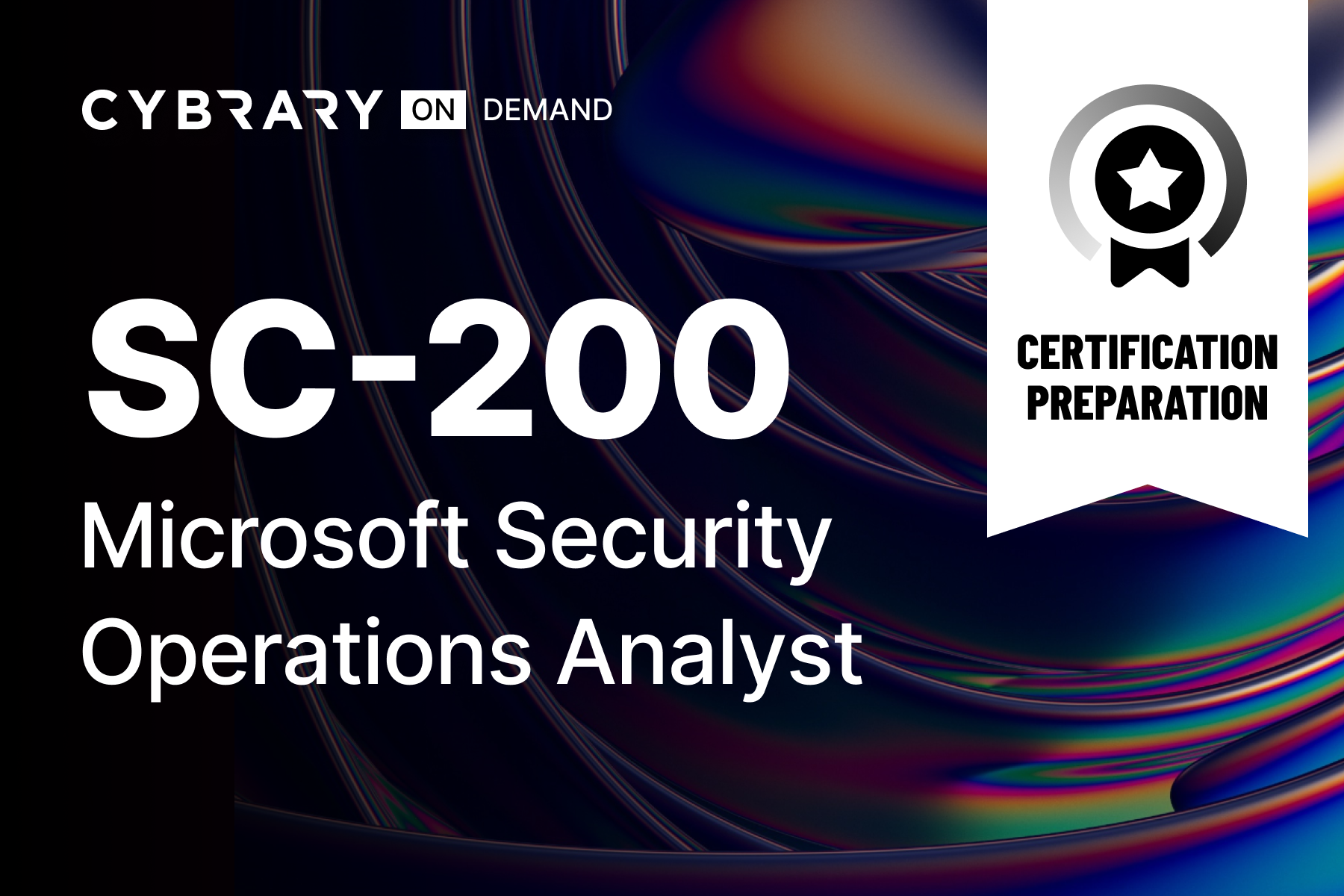
SC-200 Microsoft Security Operations Analyst
Learn to reduce risk by rapidly remediating active attacks in your environment, advising on improvements to threat protection practices, and referring policy violations to appropriate stakeholders. You will get the most out of this course if you are familiar with Microsoft 365, Azure cloud services, and Windows and Linux operating systems.
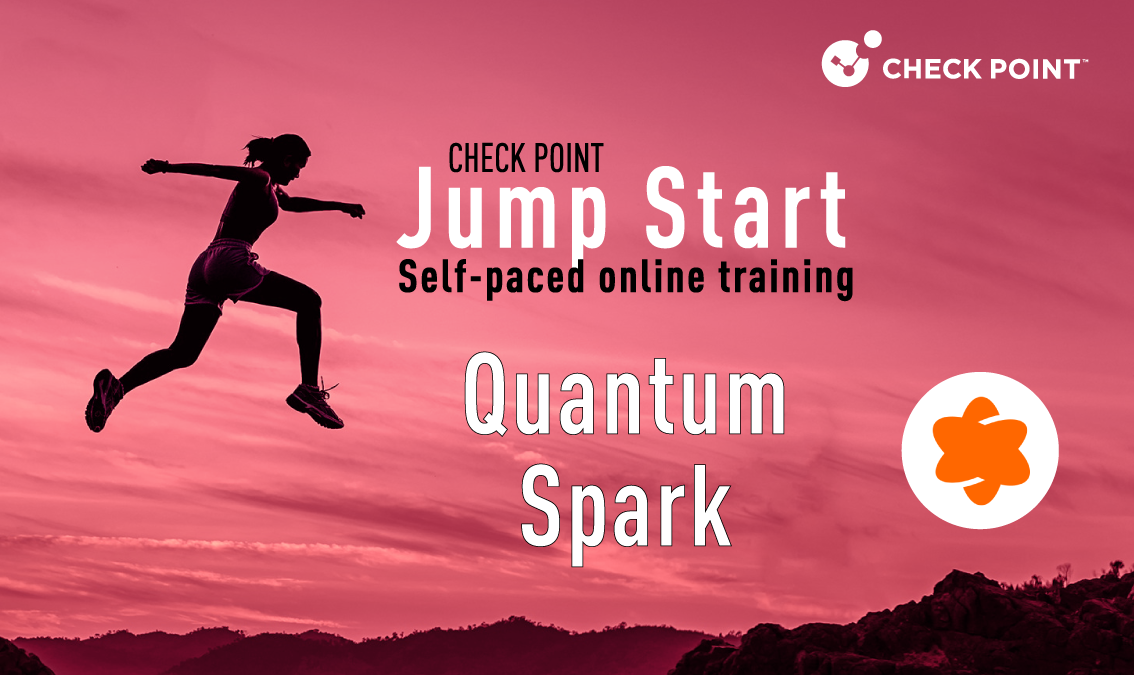
Check Point Jump Start: Quantum Spark Network Security
The Check Point Quantum Spark 1500 Pro security gateway family delivers enterprise-grade security in a series of simple and affordable, all-in-one security solutions to protect small business employees, networks, and data from cyber-theft. This course is suitable for new learners with no prior experience with Check Point Network Security products.
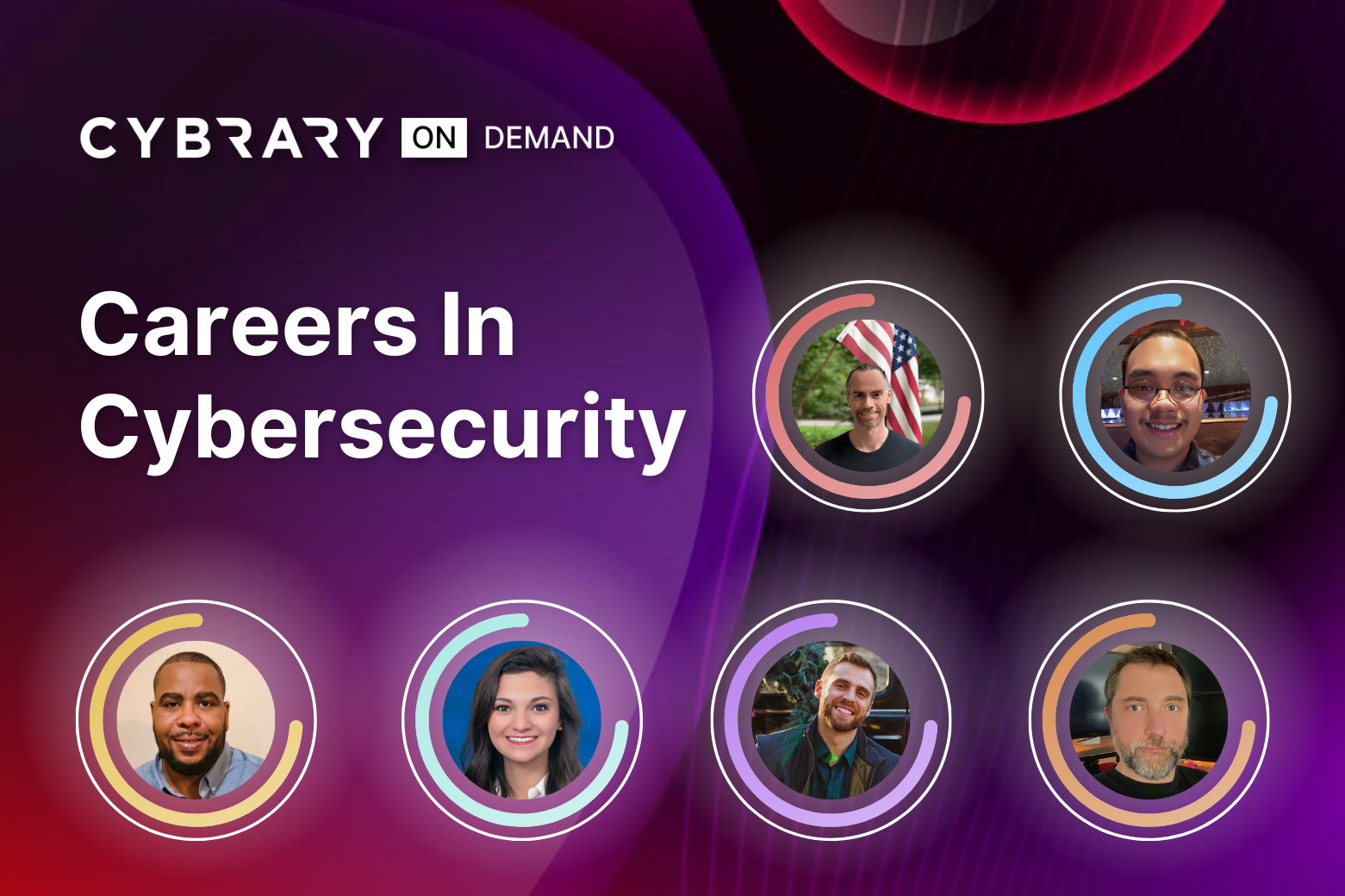
Careers In Cybersecurity
Getting started in cybersecurity can be tough, and the number of careers seems endless. This course will get you hands-on with six of the most common cybersecurity roles, including penetration tester, digital forensics, cloud security, governance, security analyst, and security engineer. Decide which career is right for you today!
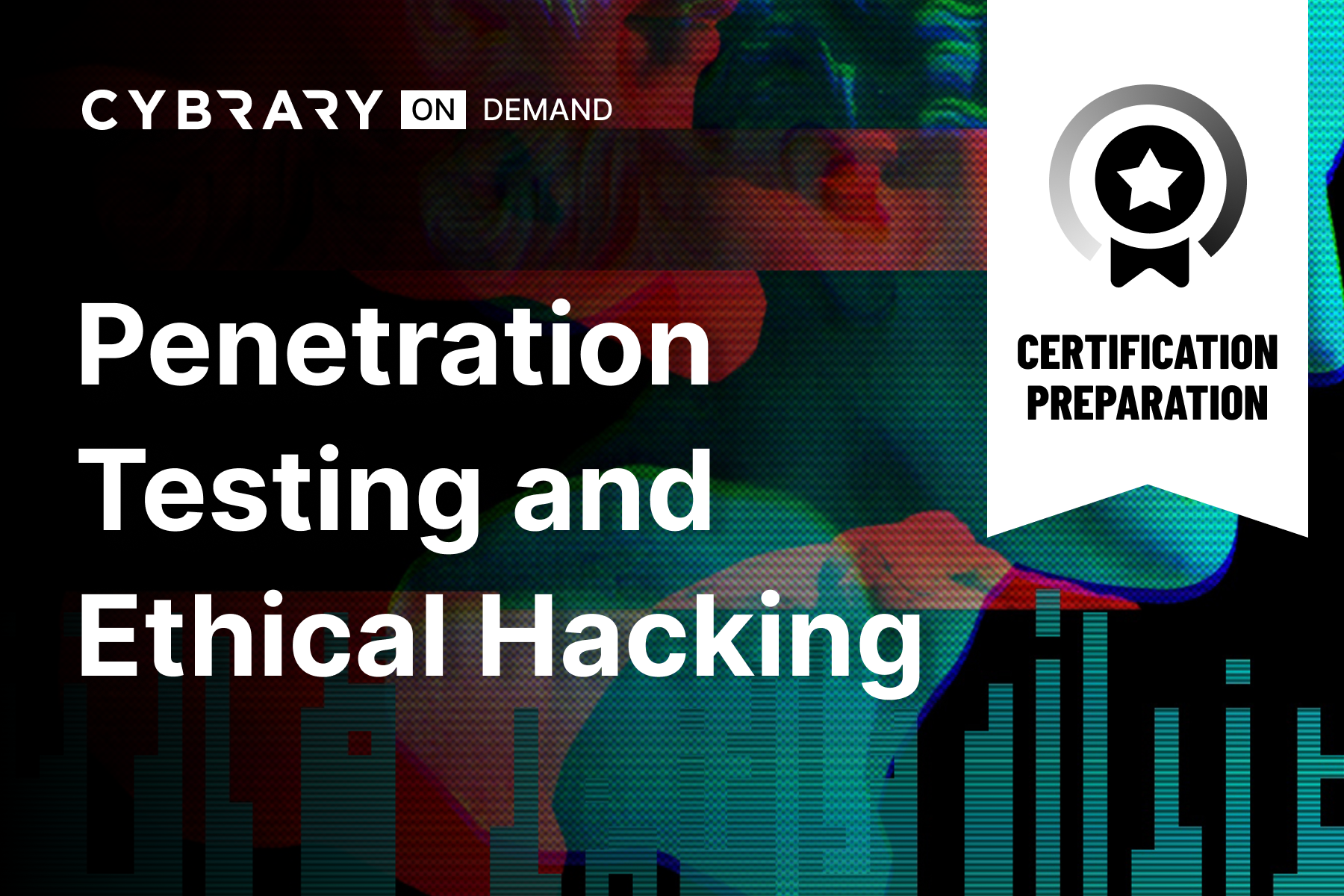
Penetration Testing and Ethical Hacking
To assess the strength of your organization’s cybersecurity posture, you need to gather information, perform scanning and enumeration, and show how an adversary could hack into your systems. This ethical hacking course will give you those skills and prepare you for related certification exams so you can prove your capabilities.
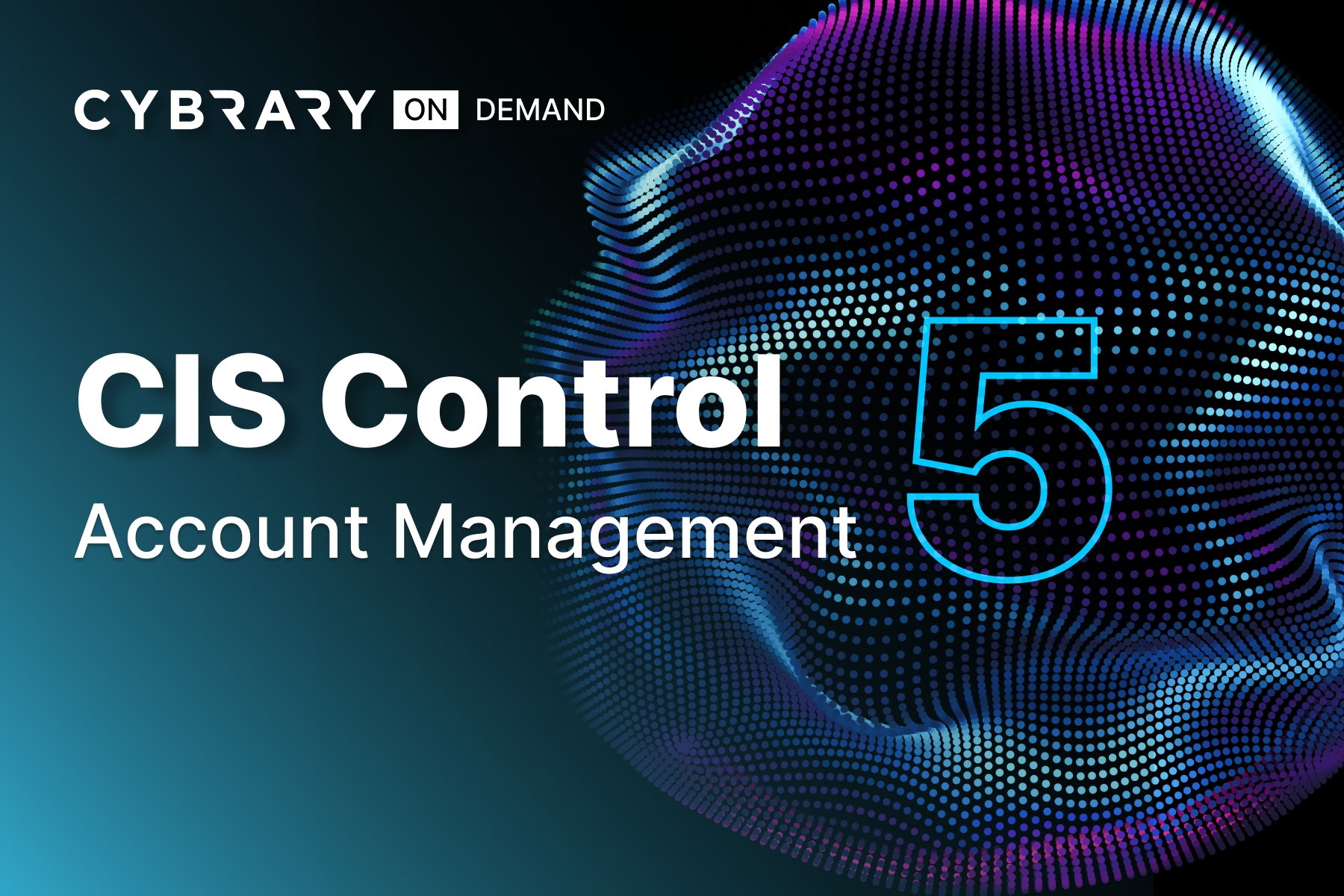
CIS Critical Security Control 5: Account Management
Welcome to our course series on CIS Top 18 Critical Security Controls v8. In this course covering control 5: Account Management, you'll learn best practices for establishing group policies for complex and unique passwords for users. Plus, gain hands-on experience with disabling dormant accounts and centralizing account management.
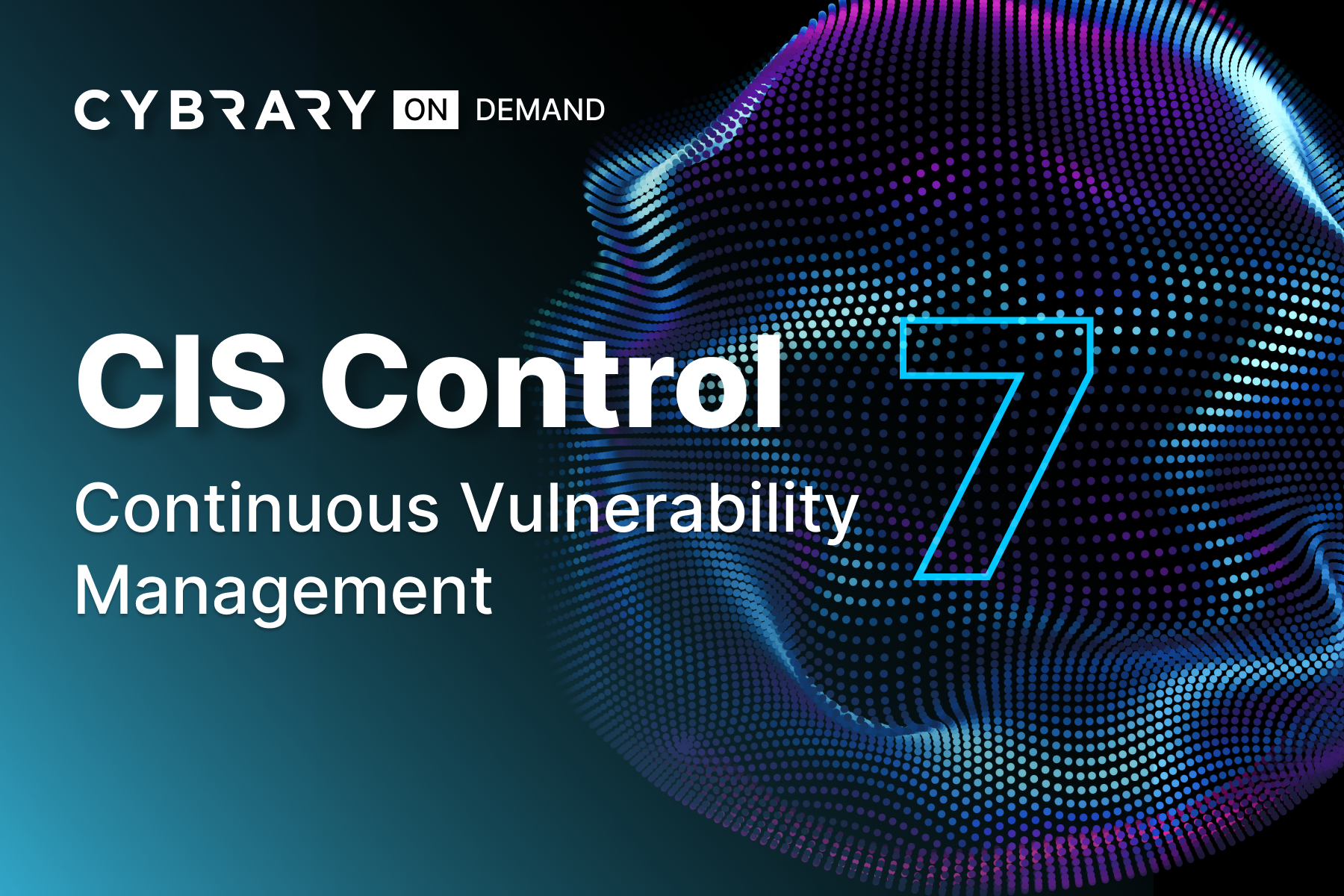
CIS Critical Security Control 7: Continuous Vulnerability Management
Welcome to our course series on CIS Top 18 Critical Security Controls v8. In this course covering control 7: Continuous Vulnerability Management, you'll demonstrate how to configure a repository for Linux servers and configure a Windows System Update Service for a domain controller. Get hands-on with automated OS and application patch management!
Cybrary Challenges
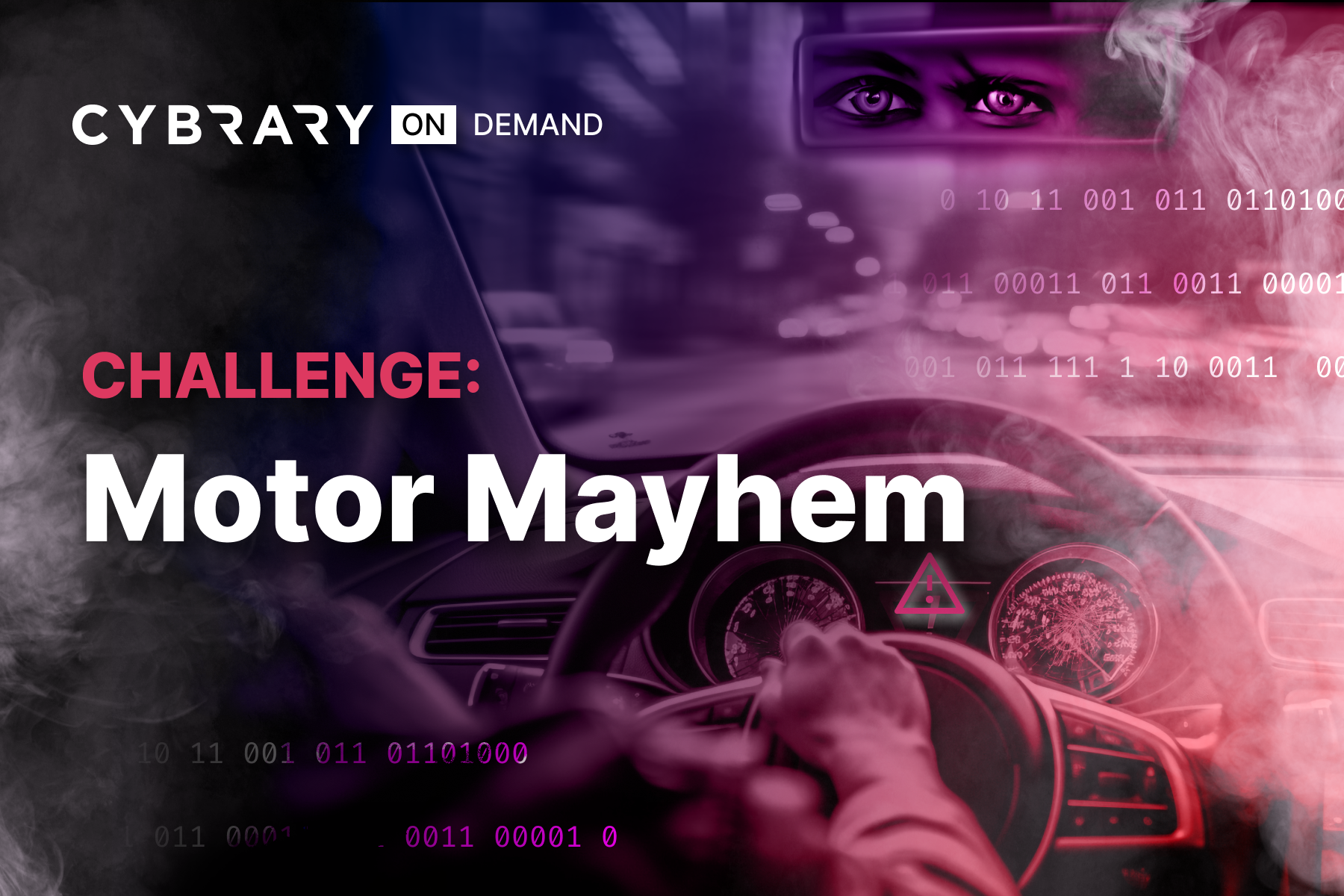
Cybrary Challenge: Motor Mayhem
In this challenge, you will take on a CAN Bus challenge by analyzing a CAN Bus dump file. The Controller Area Network (CAN bus) is a message-based protocol found in the automobiles that we use today. Every action of the car is recorded into this dump file. This allows us to read vehicle operations such as turn signals and other vehicle operations.
Cybrary Challenges
Cybrary Challenges
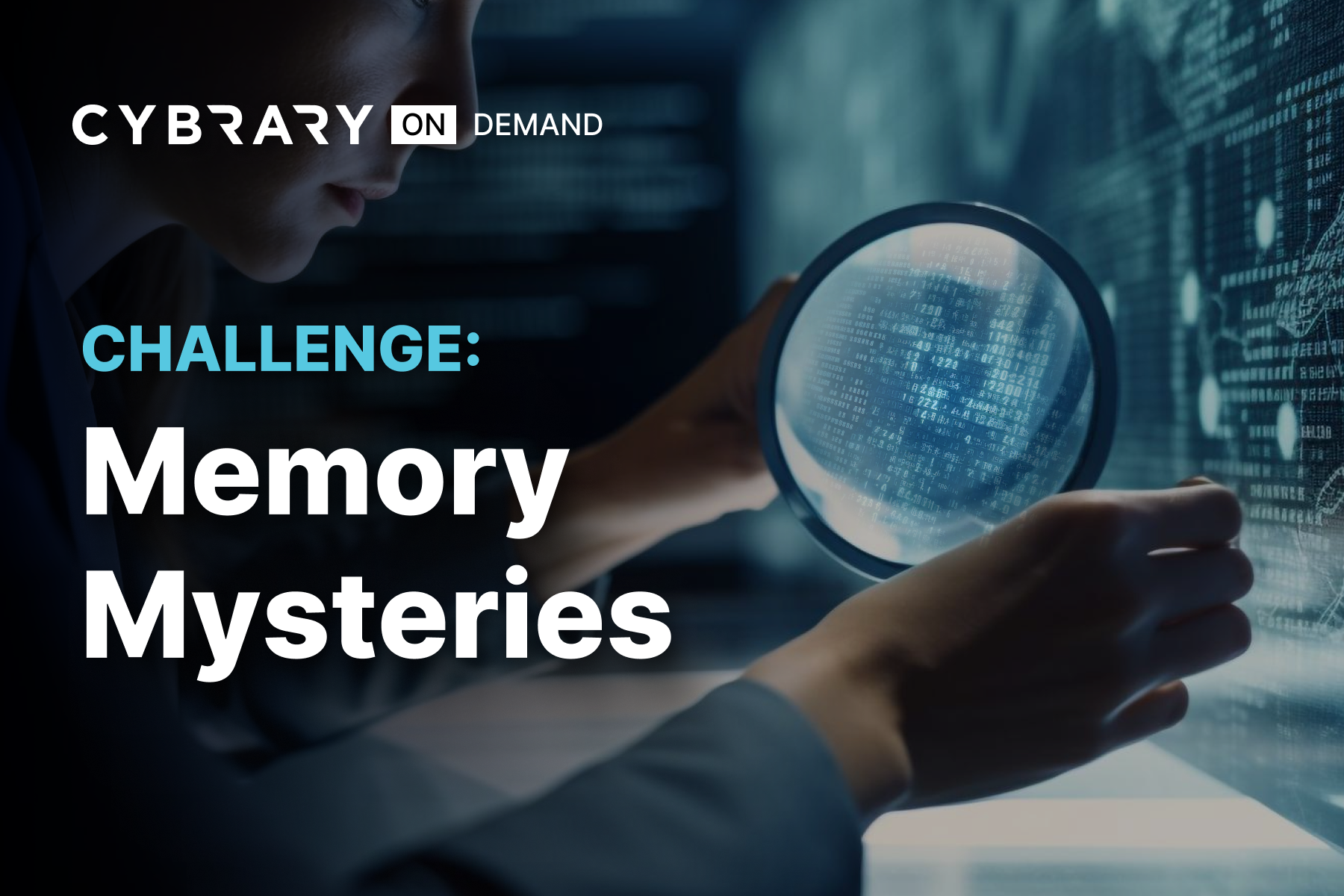
Challenge: Memory Mysteries
In this challenge, you will take on a memory forensics challenge by analyzing a dump file. Memory forensics is an important piece of evidence when conducting a forensics investigation. Unique insights such as network connections and injected processes can be found through memory analysis.
Cybrary Challenges
Cybrary Challenges
Cybrary Challenges
Cybrary Challenges
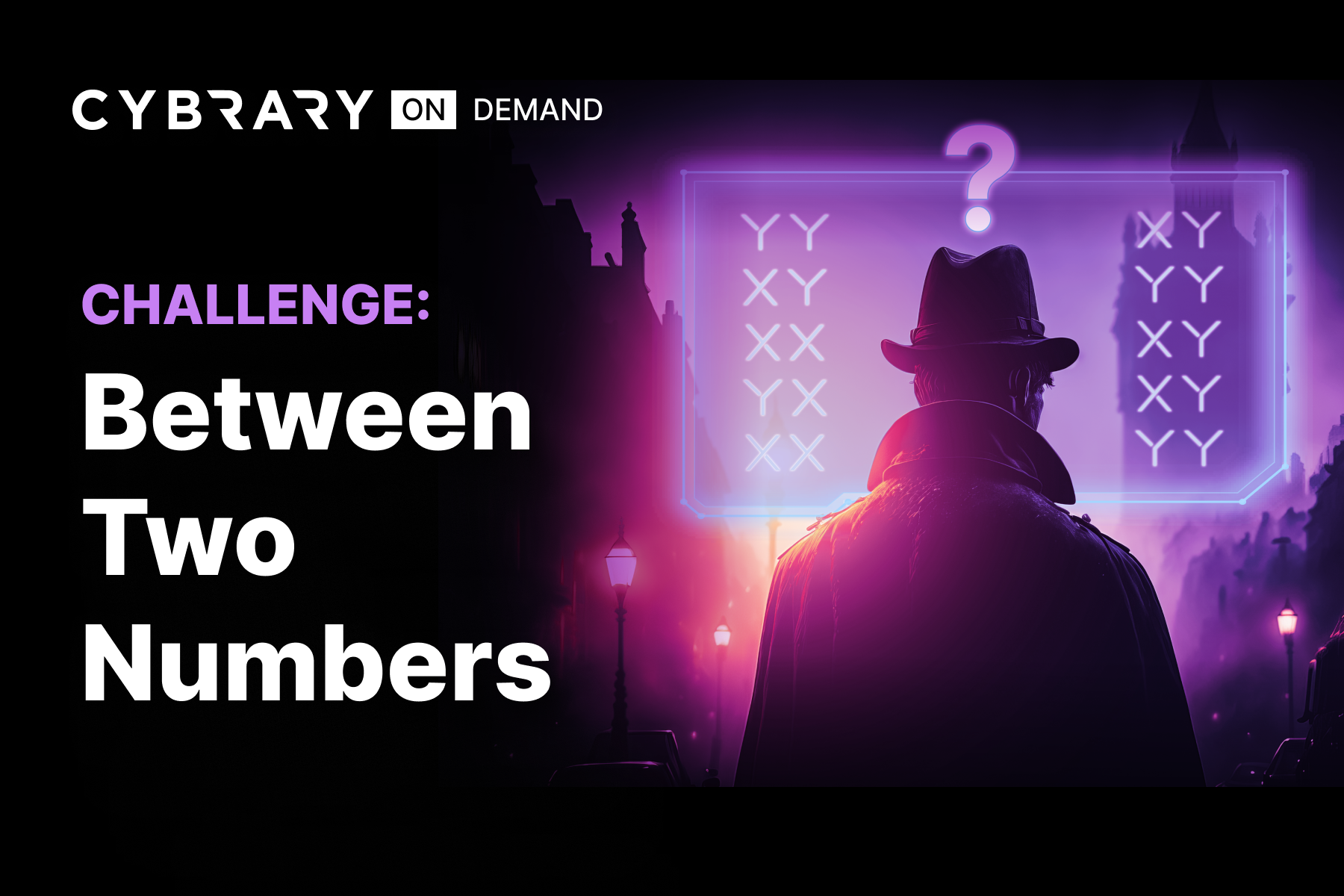
Challenge: Between Two Numbers
In this challenge, you will take on a reverse engineering challenge by analyzing a binary file. Malicious attackers will always use malware or custom binary files to execute their goals. The goal is to show from an analyst point of view (POV) how to do basic file analysis.
Cybrary Challenges
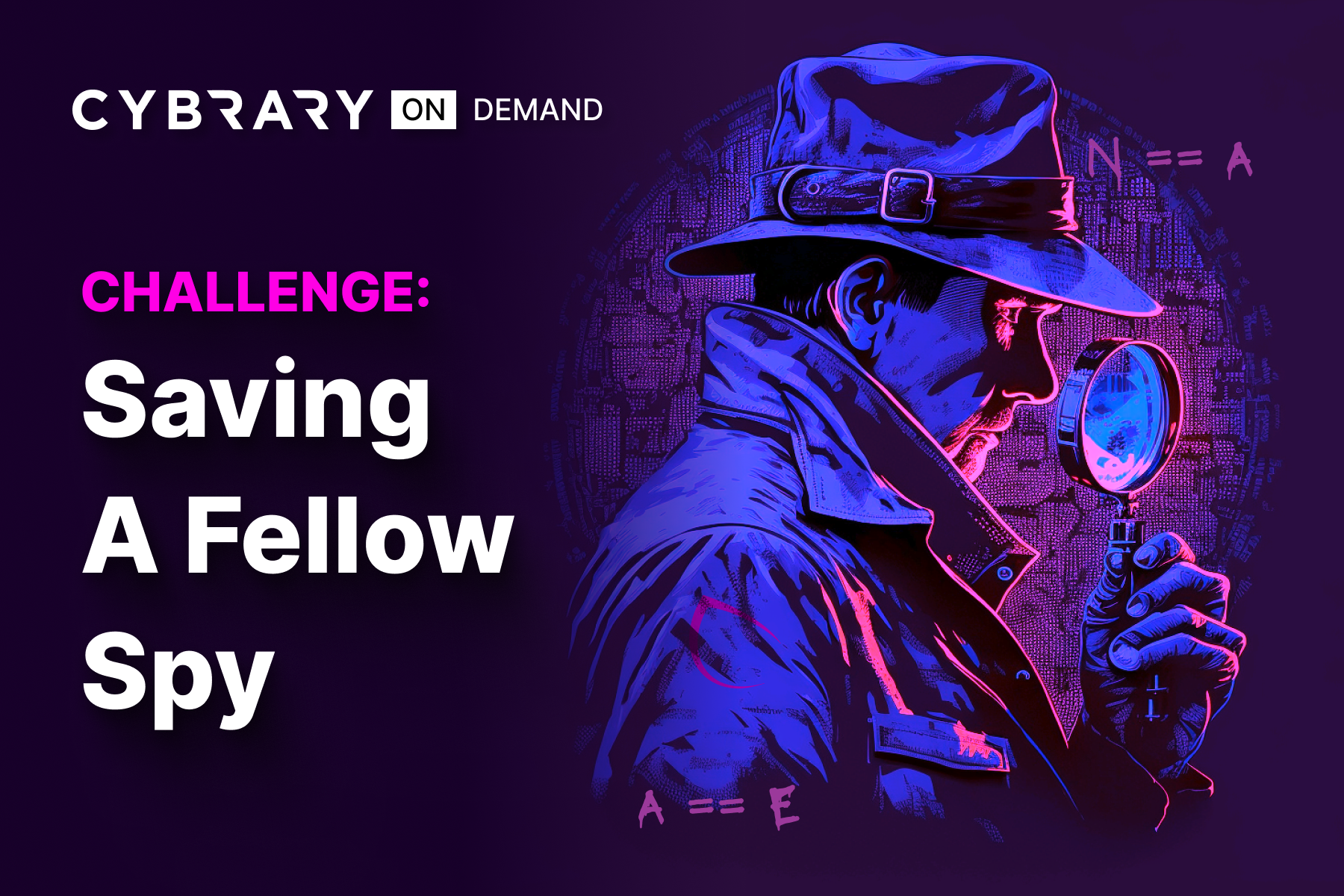
Challenge: Saving A Fellow Spy
You will take on a cryptography challenge in this challenge by decrypting intercepted encrypted messages. Malicious attackers use cryptography to their advantage for attacks and remaining undetected. The goal is to show how attackers can effortlessly embed data within messages to hide their activity.
Cybrary Challenges
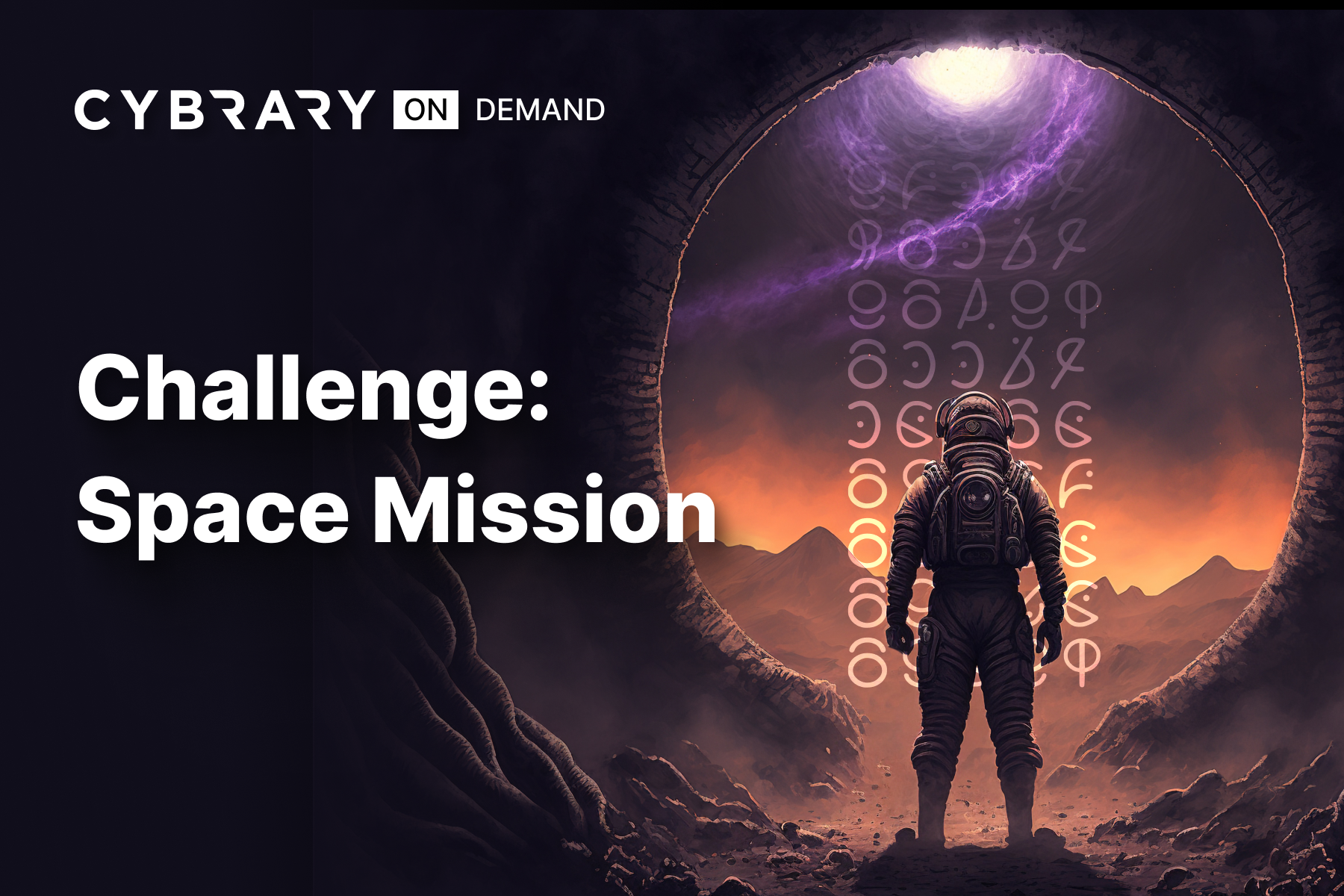
Challenge: Space Mission
In this challenge, you will take on a cryptography challenge by decrypting intercepted encrypted messages. Malicious attackers use cryptography to their advantage for attacks and remaining undetected. The goal is to show how attackers can effortlessly embed data within messages to hide their activity.
Cybrary Challenges
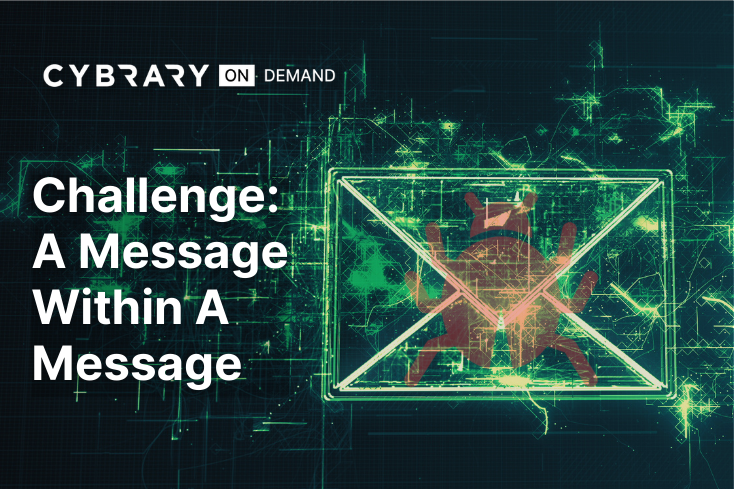
Challenge: A Message Within A Message
In this challenge, you will take on a cryptography challenge by decrypting intercepted encrypted messages. Malicious attackers use cryptography to their advantage for attacks and remaining undetected. The goal is to show how attackers can effortlessly embed data within messages to hide their activity.
Cybrary Challenges
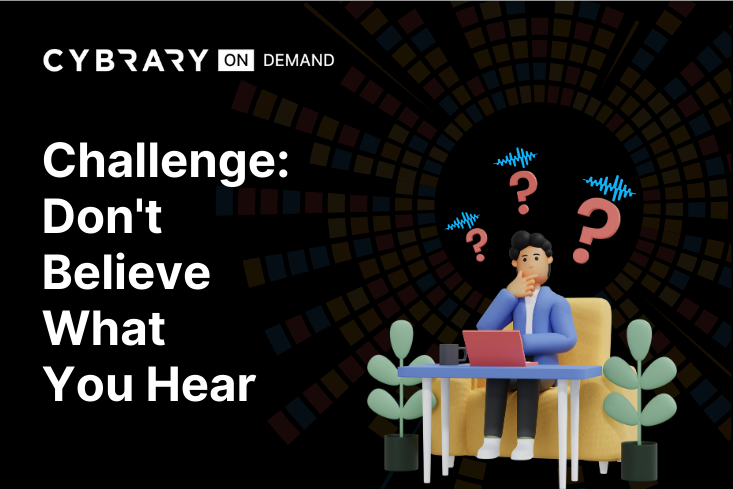
Challenge: Don't Believe What You Hear
In this challenge, you will take on a Steganography challenge identifying embedded data within an audio file. Malicious attackers use Steganography for attacks such as macro-enabled Word documents, to conceal covert communication, and more. The goal is to show how attackers can effortlessly embed data within files to hide their activity.
Cybrary Challenges
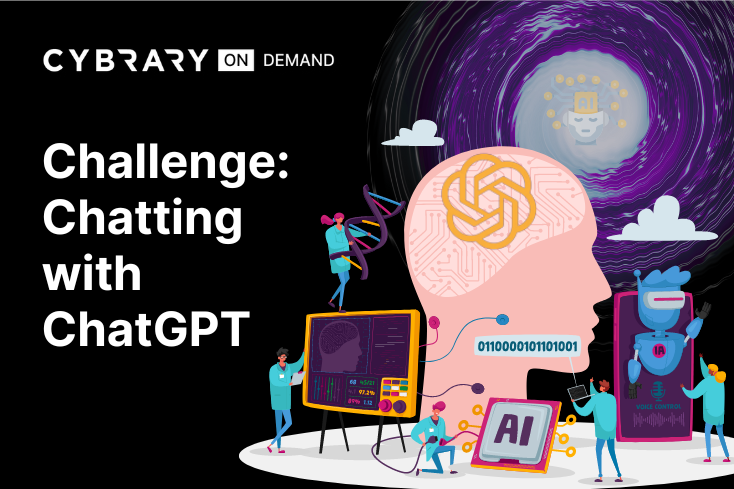
Challenge: Chatting with ChatGPT
In this challenge, we will take a different approach and dive into the world of AI technology. By completing this challenge, you will learn more about ChatGPT, how it works, its capabilities, and its limitations. As cybersecurity professionals, it is essential to adapt to the ever-changing technology and security landscape.
Cybrary Challenges
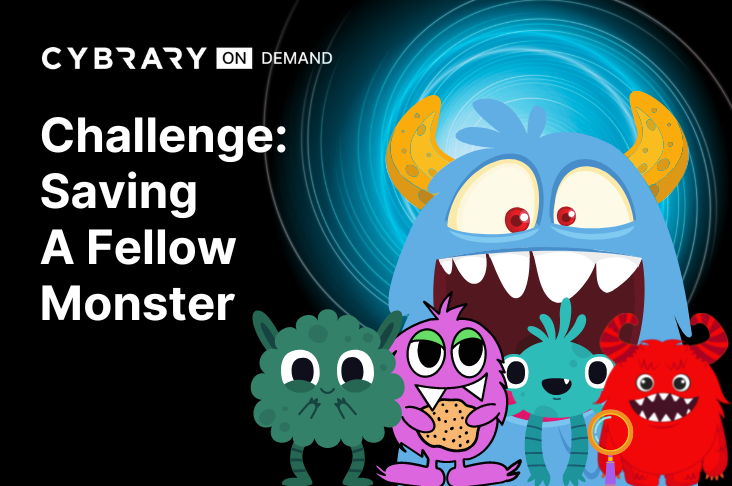
Challenge: Saving a Fellow Monster
In this challenge, you will take on a Steganography challenge identifying embedded data within an image. Malicious attackers use Steganography for attacks such as macro-enabled Word documents, to conceal covert communication, and more. The goal is to show how attackers can effortlessly embed data within files to hide their activity.
Cybrary Challenges
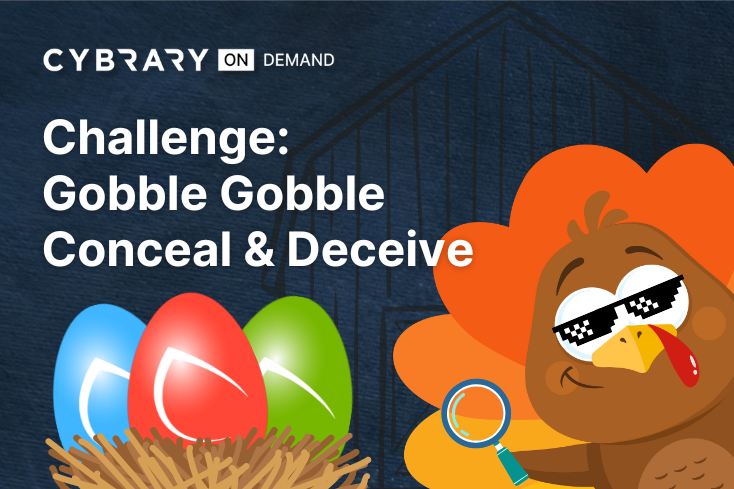
Challenge: Gobble Gobble Conceal & Deceive
In this challenge, you will take on a Steganography challenge identifying embedded data within an image. Malicious attackers use Steganography for attacks such as macro-enabled Word documents, to conceal covert communication, and more. The goal is to show how attackers can effortlessly embed data within files to hide their activity.
Cybrary Challenges
Cybrary Challenges
Cybrary Challenges
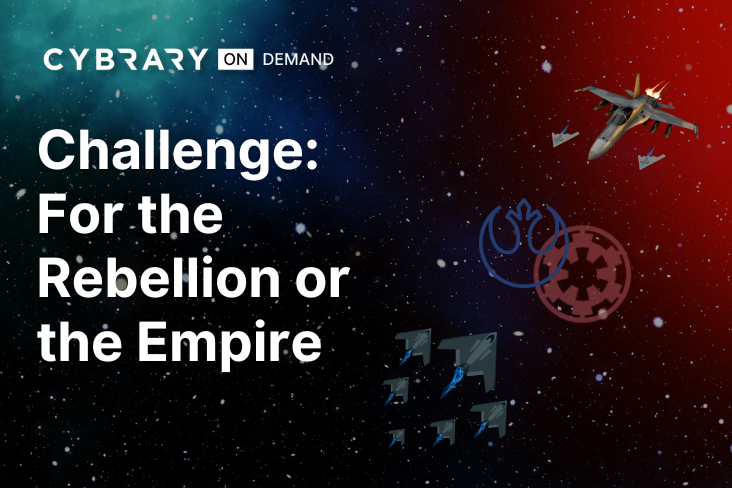
Challenge: For the Rebellion or the Empire
This challenge will have you analyze traditional Registry artifacts to identify unauthorized activity. The goal is to see from a blue teamer's point of view the actions an unauthorized user may take on a victim's system when there are inadequate security controls in place.
Cybrary Challenges
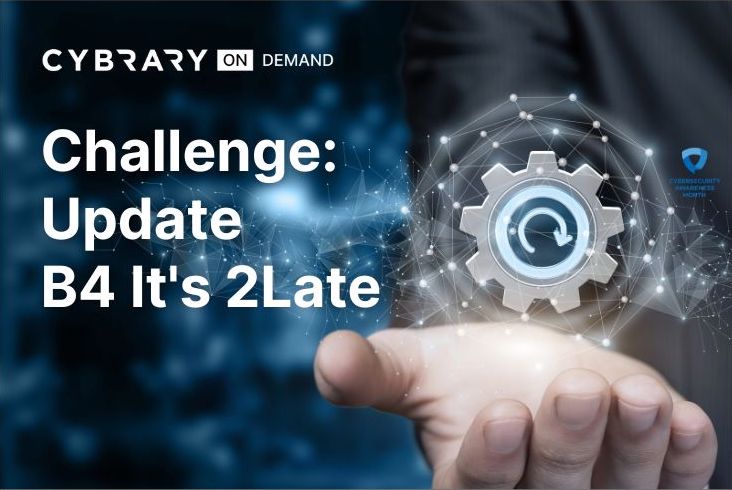
Challenge: Update B4 It's 2Late
National Cybersecurity Awareness Month has four themes; the last being 'Update Your Software.' This challenge will have you analyze a log and identify a web application attack. The goal is to piece together the narrative from the suspicious requests and understand how attacks like these can happen when you do not update your software.
Cybrary Challenges
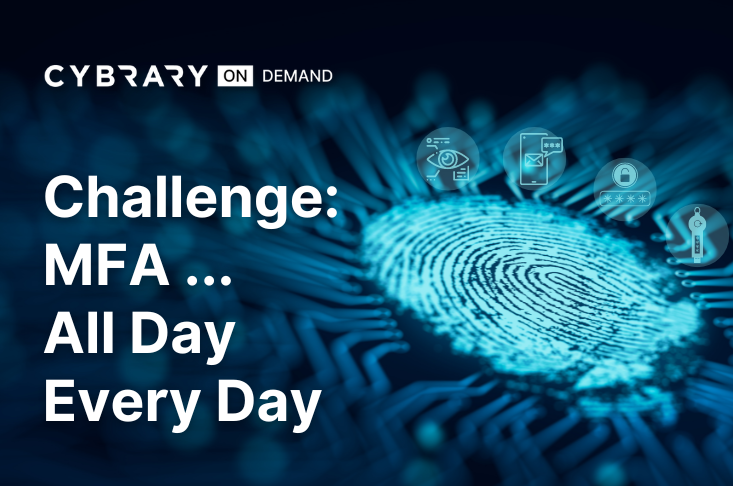
Challenge: MFA ... All Day Every Day
National Cybersecurity Awareness Month has several themes, one of which is Multi-Factor Authentication (MFA). This MFA challenge will have you analyze a log and identify the potential MFA attack. The goal is to review suspicious requests and identify how MFA can be attacked in real-world use cases.
Cybrary Challenges
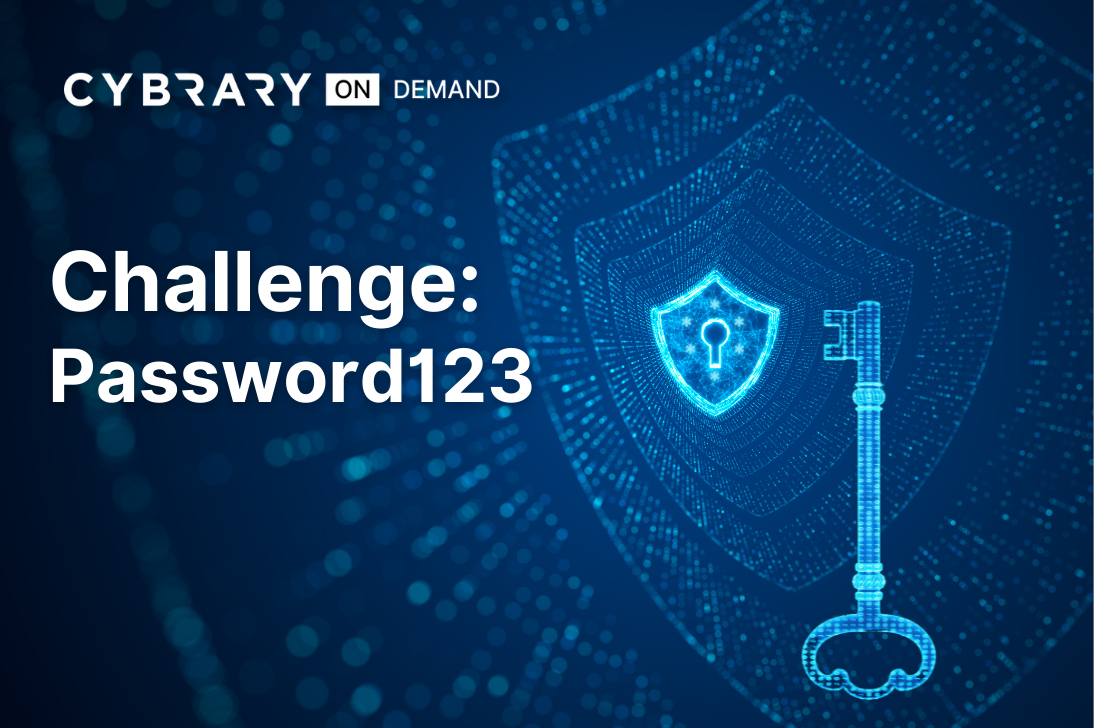
Challenge: Password123
National Cybersecurity Awareness Month has several themes, one of which is Password Complexity Awareness. This password challenge will have you analyze a password and validate if it is secure. The goal is to attempt to crack a password and gain hands-on skills to evaluate the importance of password complexity for real-world use cases.
Cybrary Challenges
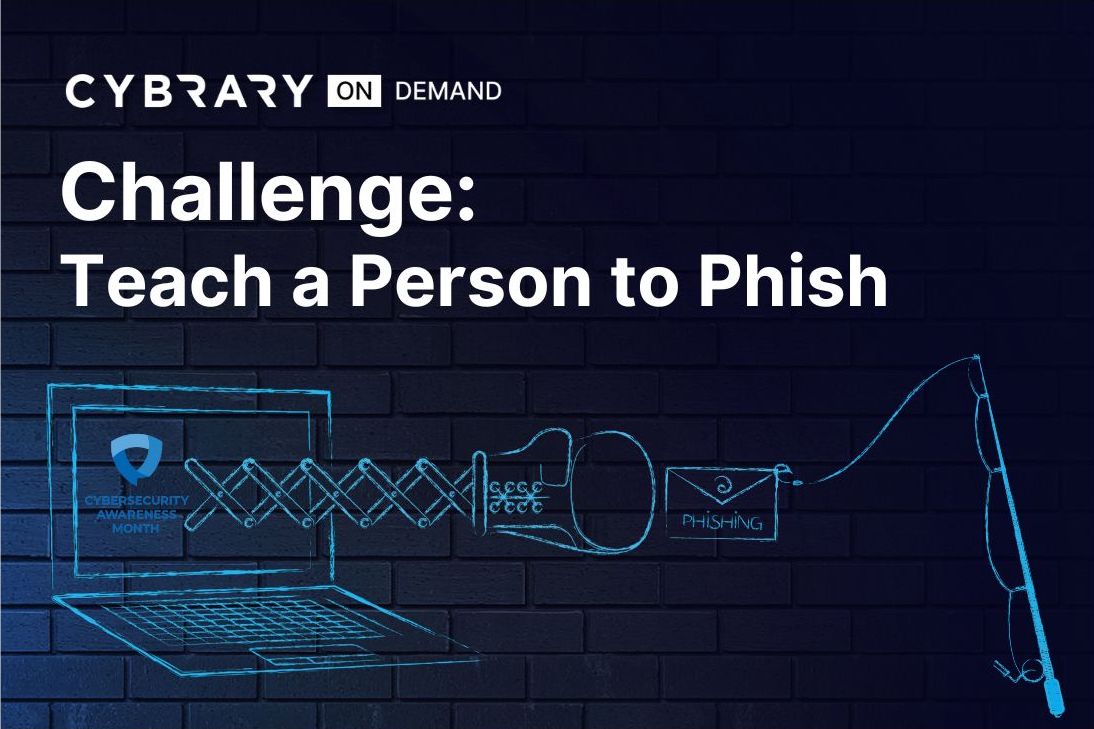
Challenge: Teach a Person to Phish
National Cybersecurity Awareness Month has several themes, one of which is Phishing Awareness. This phishing challenge will have you analyze a real phish caught in the wild! The goal is to identify exactly why it was flagged as a phish and gain hands-on skills to validate a suspicious email!
Cybrary Challenges

Challenge: Episode II - Attack of the Encoders
Adversaries commonly use encoding, encryption, and hashing to obscure their scripts and attacks. As a CTF player, you will need to analyze alerts and uncover the true nature of a suspicious string embedded in a file. Can you help figure out what it’s trying to say?
Cybrary Challenges
Cybrary Challenges
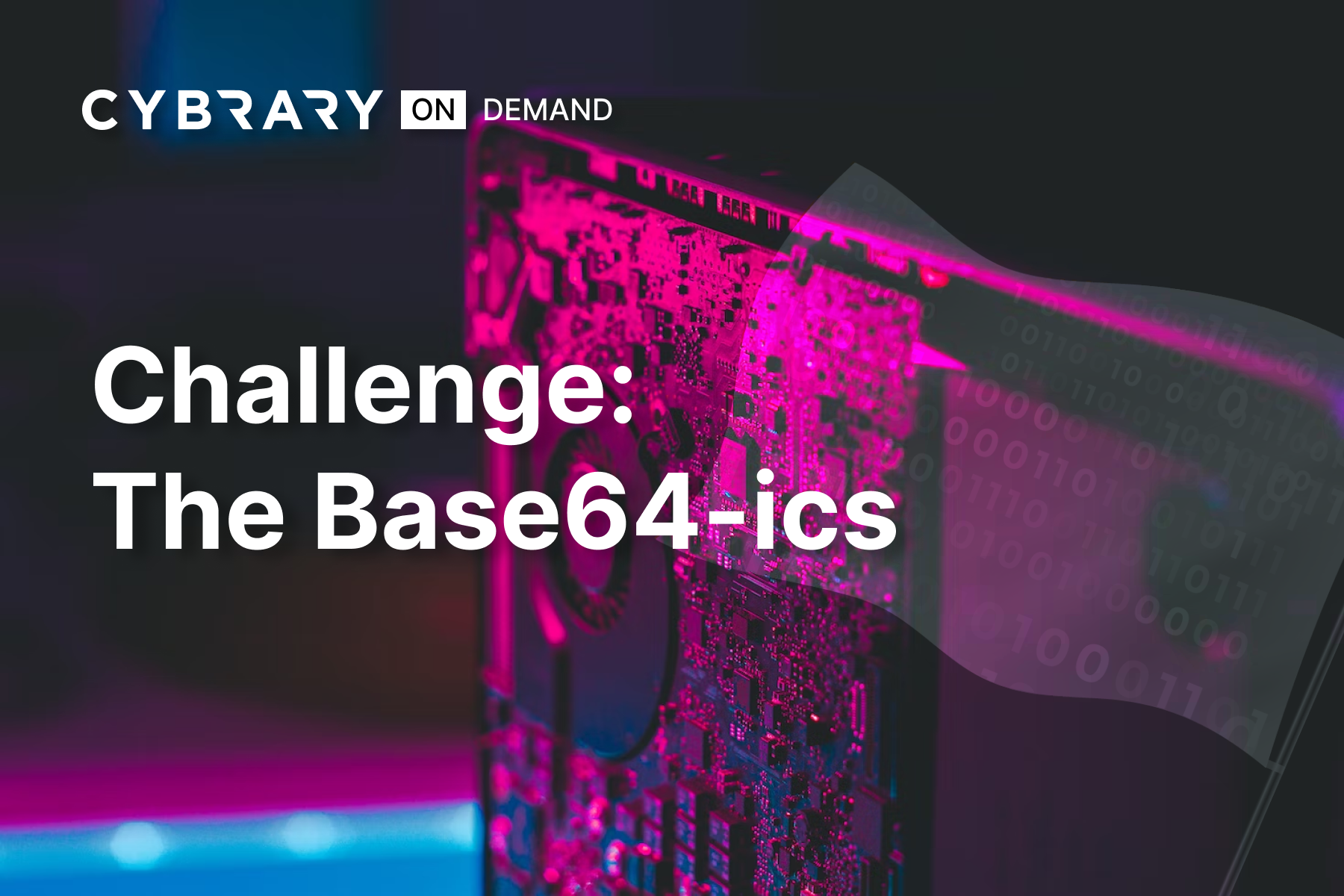
Challenge: The Base(64)ics
Threat actors commonly use legitimate tools in nefarious ways. As a CTF player, you’ll need to find creative ways to uncover these types of tactics. While evaluating a recent alert in your EDR, you’ve come across a weird string at the end of a powershell command. Can you help figure out what it’s trying to say?
Cybrary Challenges
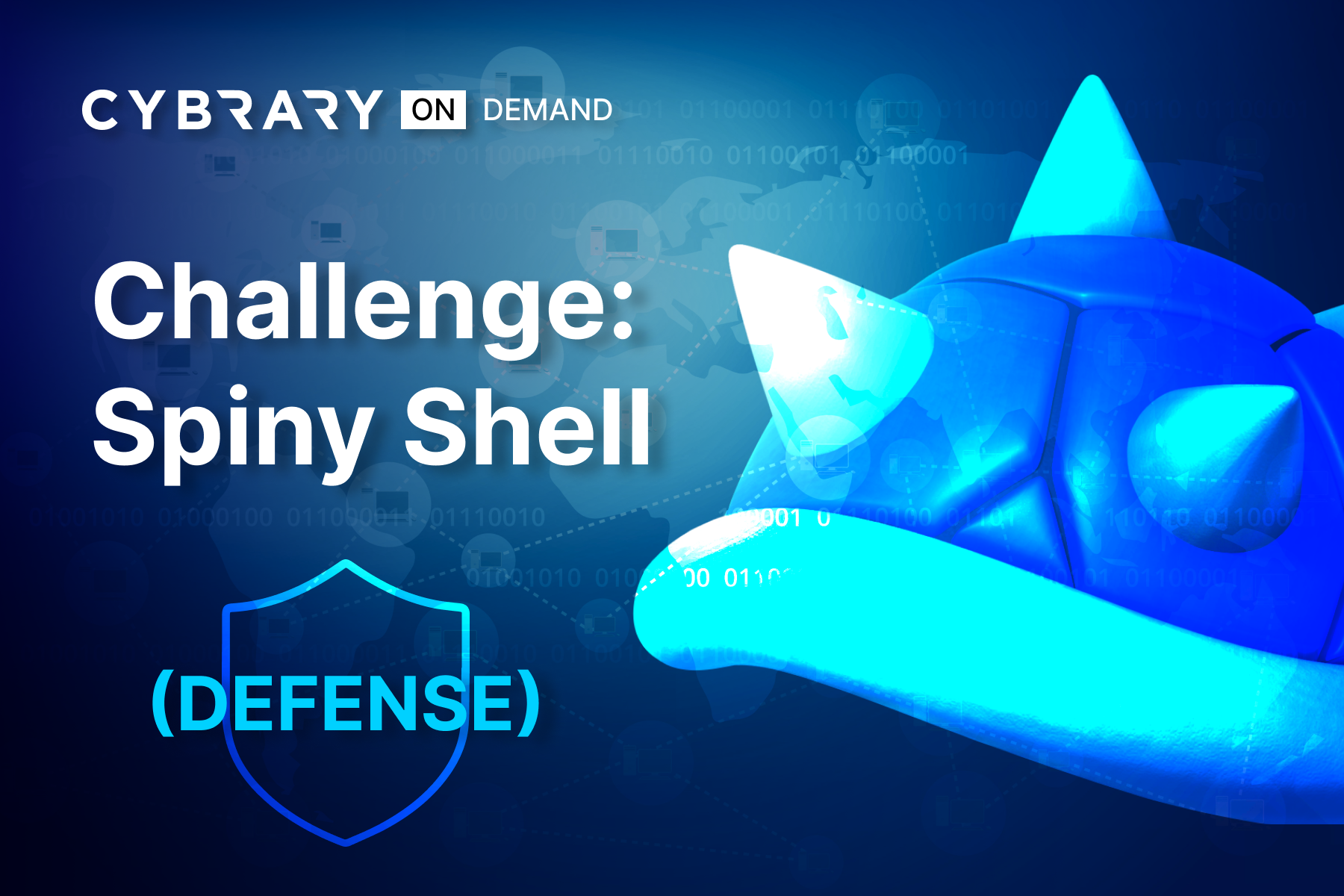
Challenge: Spiny Shell
You receive an alert about a suspicious command execution on a Windows endpoint. Early analysis suggests PowerShell has not locked down appropriately. Can you validate if anything malicious is underway? Now that you have some basic information discovered, dive deeper into the suspicious command to identify the attacker's infrastructure and setup!
CVE Series
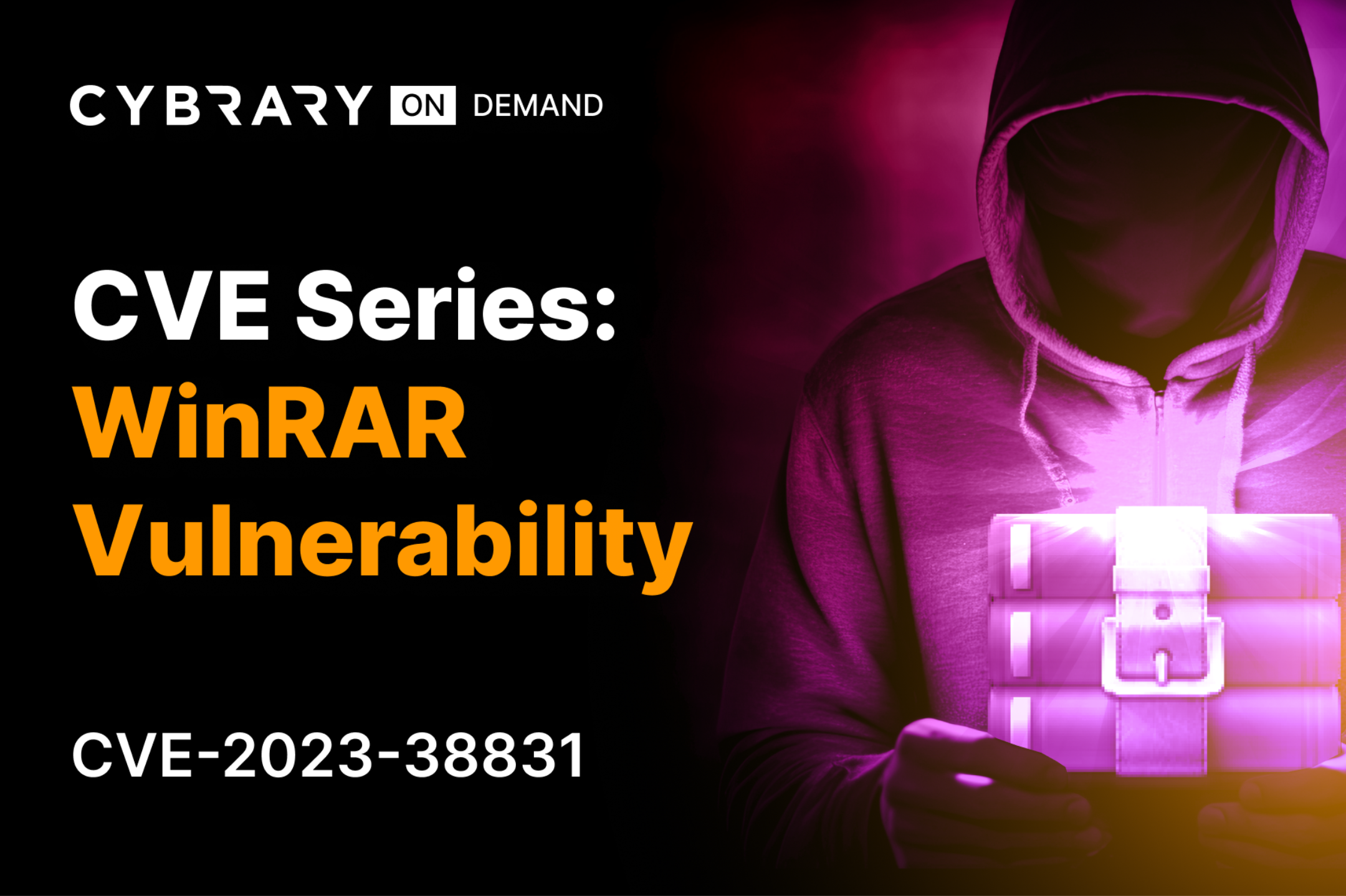
CVE Series: WinRar Vulnerability (CVE-2023-38831)
WinRAR, a popular 1990s file archiver still used by 500 millions users worldwide, suffers from a high severity Remote Code Execution (RCE) vulnerability. In this course you’ll be putting on your Red Team hat to create your own malicious file and gain control of the victim’s computer by leveraging this CVE!
CVE Series
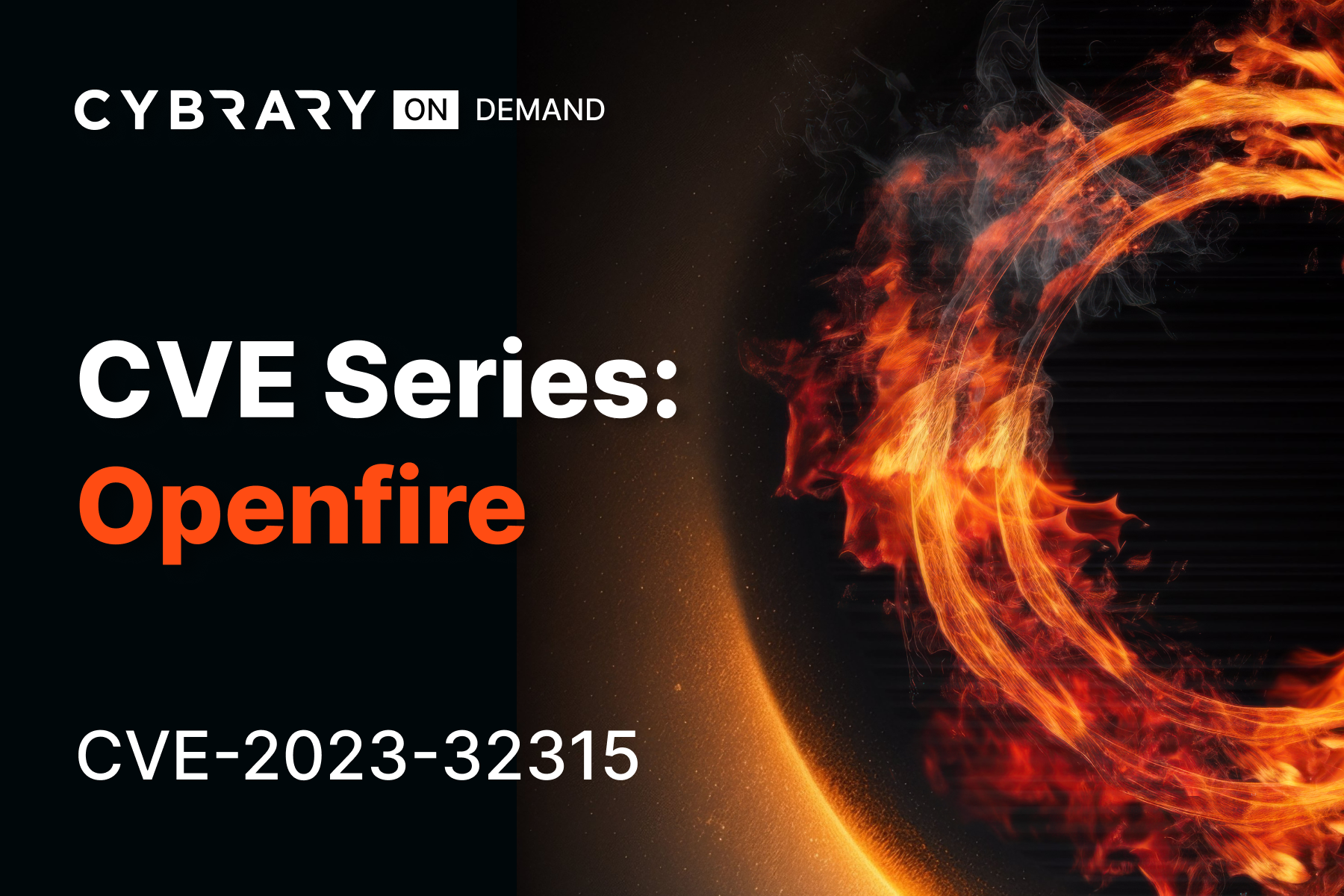
CVE Series: Openfire (CVE-2023-32315)
If you're a cybersecurity practitioner who wants to know more about how to exploit and defend against CVE-2023-32315 (Openfire Path Traversal to RCE), you won't want to miss this course. You will identify the vulnerability, exploit it, and mitigate it in a hands-on, secure lab environment. Don't let Openfire catch you off guard.
CVE Series
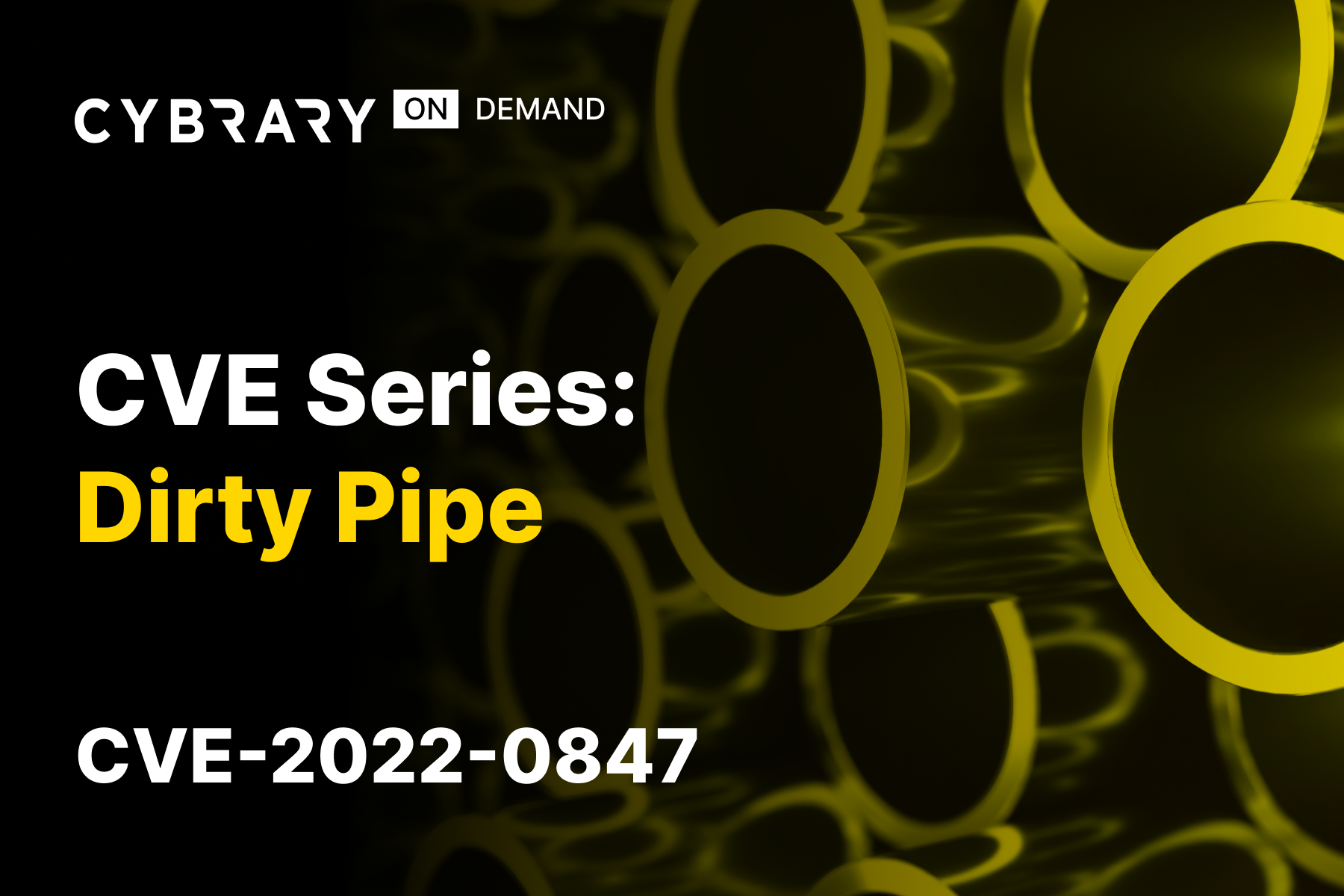
CVE Series: Dirty Pipe (CVE-2022-0847)
Dirty Pipe (CVE-2022-0847) is the most critical vulnerability to impact Linux distributions in years. By exploiting this local kernel flaw, adversaries can quickly escalate privileges and even gain root access. Exploit and mitigate this vulnerability in this hands-on course that gives you the skills you need to protect your organization.
CVE Series

CVE Series: noPac (CVE-2021-42278 and CVE-2021-42287)
Ready to defend your organization against the critical noPac double vulnerability (CVE-2021-42278 and CVE-2021-42287) that can lead to advanced privilege escalation on Windows systems? Get ahead of the curve in this hands-on course that allows you to both exploit and mitigate this vulnerability with potentially significant, far-reaching impacts.
CVE Series

CVE Series: Polkit (CVE-2021-4034)
The Polkit vulnerability (CVE-2021-4034) is a critical vulnerability impacting every major Linux distribution. Its attack vector allows privilege escalation and can even give the attacker root access. Exploit and mitigate this vulnerability in this hands-on course that gives you the skills you need to protect your organization.
CVE Series
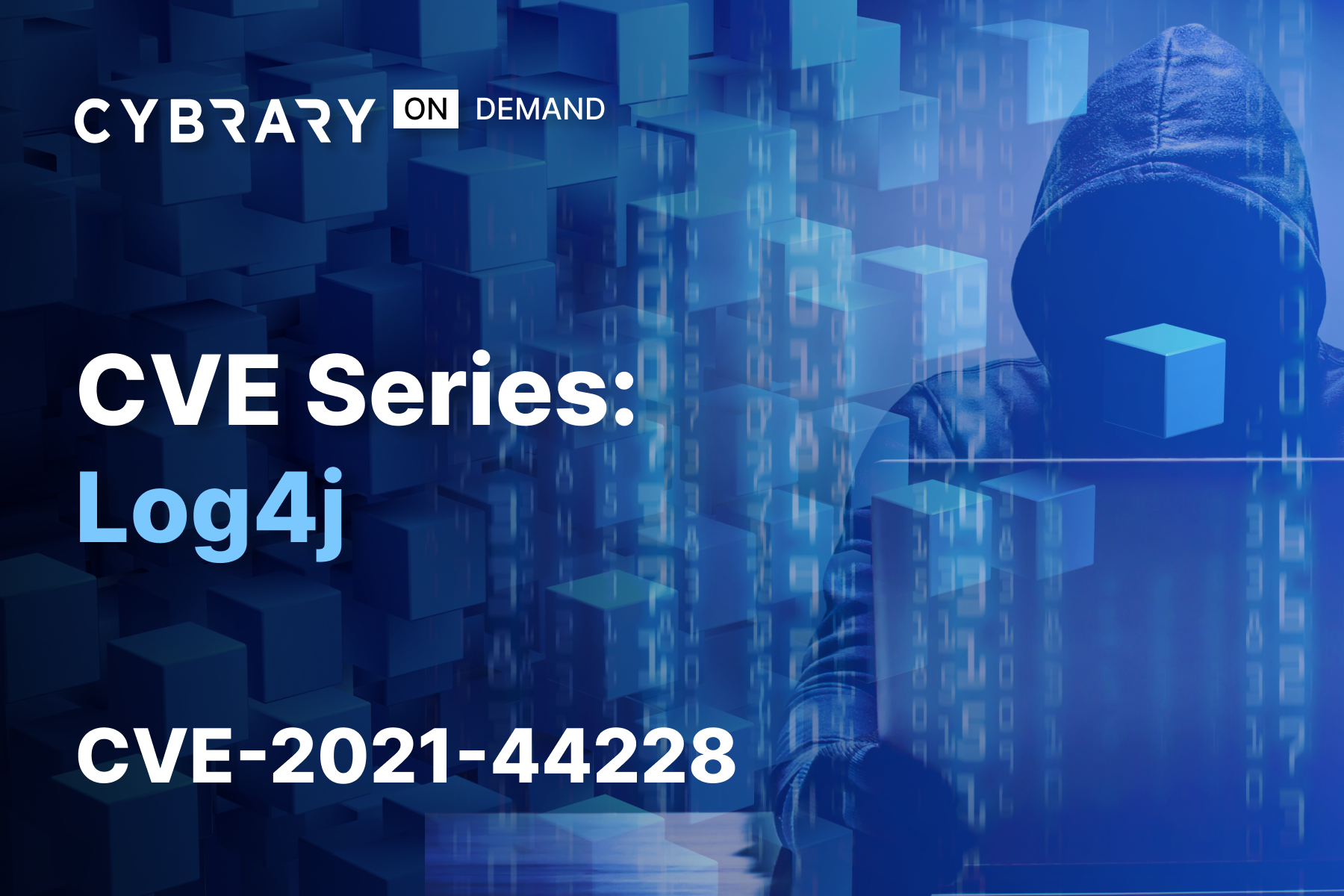
CVE Series: Log4J (CVE-2021-44228)
The Log4J vulnerability (CVE-2021-44228) took the world by storm in late 2021. Do you have what it takes to exploit and mitigate this critical vulnerability that experts say had the biggest global impact since Shellshock? Find out in this course, where you'll put your defensive and pen testing skills to the ultimate test in a virtual lab.
CVE Series
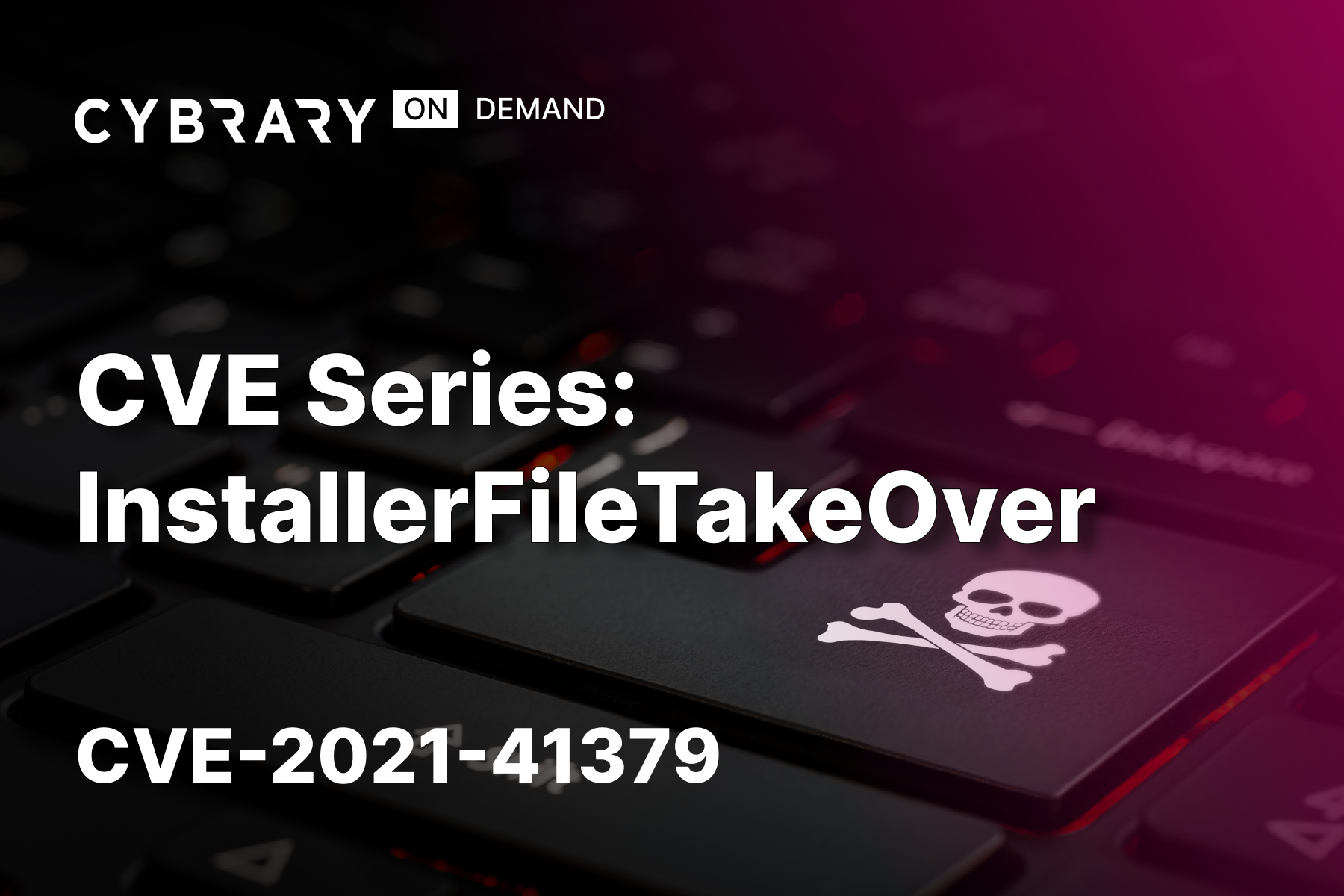
CVE Series: InstallerFileTakeOver (CVE-2021-41379)
InstallerFileTakeOver (CVE-2021-36934) is a Windows elevation of privilege vulnerability that emerged in late 2021 and could allow a threat actor to acquire elevated SYSTEM-level access. You will exploit this vulnerability in a virtual lab environment and learn how to mitigate it so you can protect your organization.
CVE Series
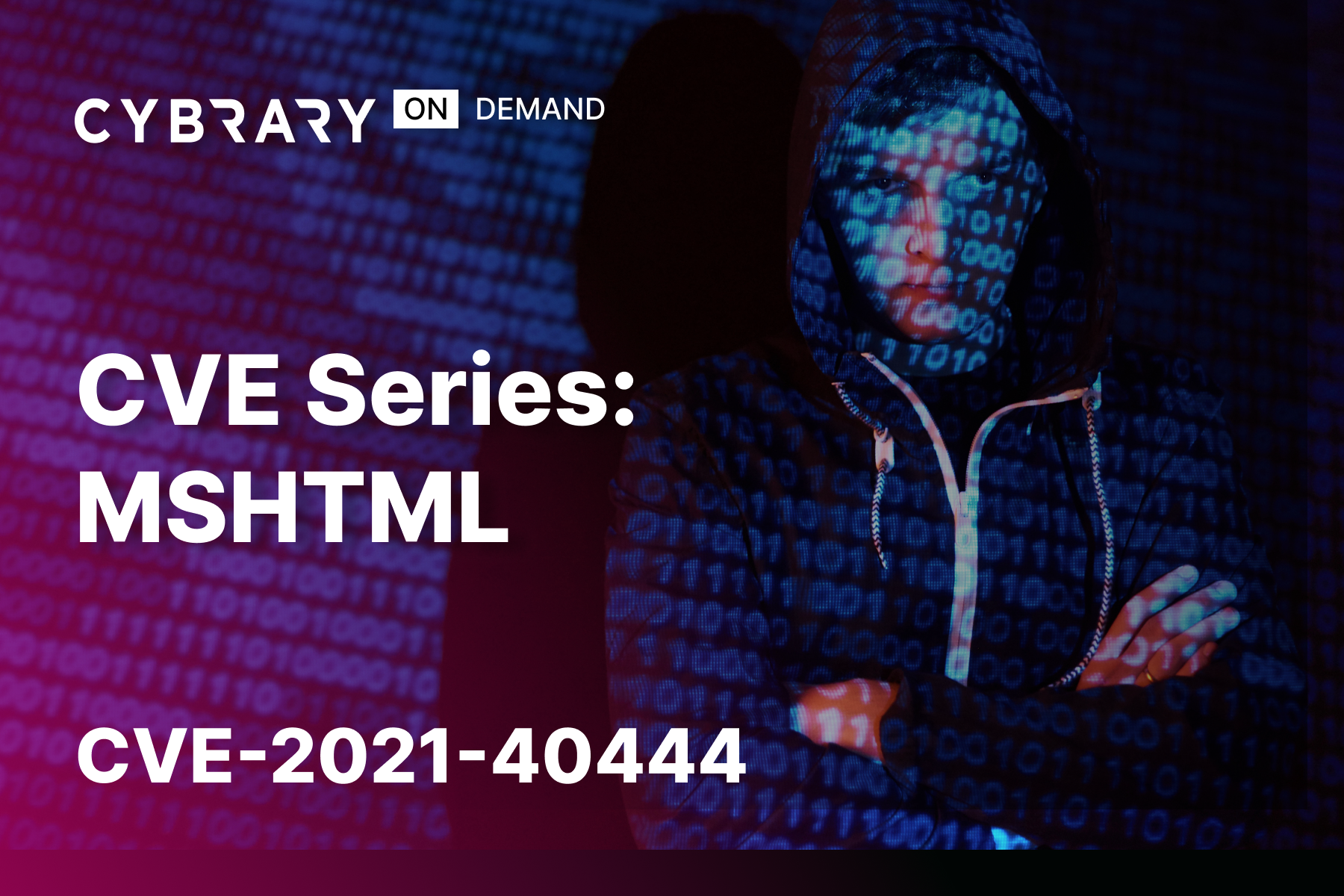
CVE Series: MSHTML Vulnerability (CVE-2021-40444)
The MSHTML Windows remote code execution vulnerability (CVE-2021-40444) identified in September 2021 could allow a threat actor to execute code on a victim’s machine. In this advanced course, you will exploit and mitigate this vulnerability in a secure lab environment, giving you the skills you need to protect your organization.
CVE Series

CVE Series: HiveNightmare (CVE-2021-36934)
HiveNightmare (CVE-2021-36934) is a serious vulnerability that gives threat actors access to sensitive data in the Windows Registry. Don't get stung by HiveNightmare. Get hands-on experience mitigating and exploiting this vulnerability in a secure lab environment by taking this course today.
CVE Series
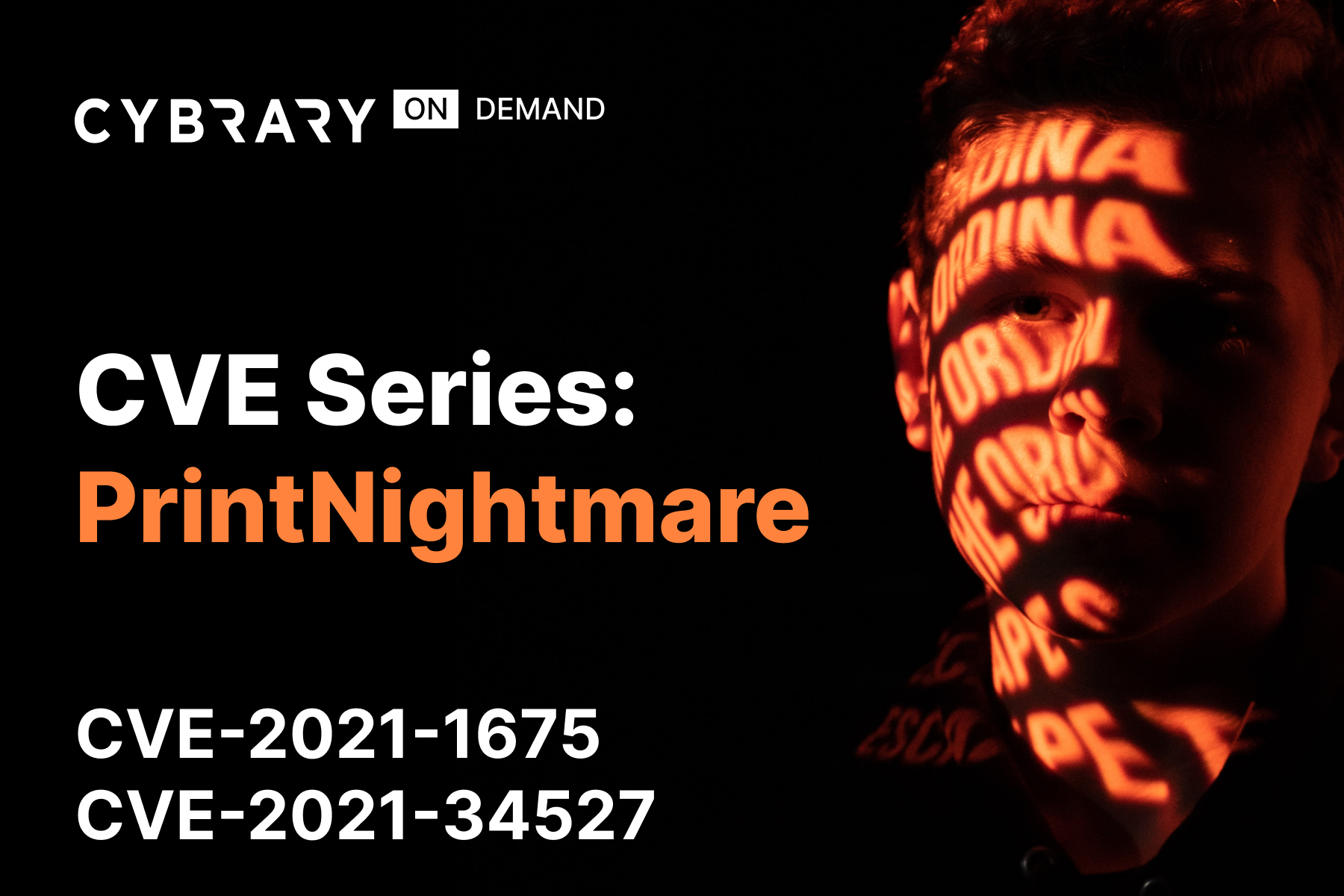
CVE Series: PrintNightmare (CVE-2021-1675 and CVE-2021-34527)
PrintNightmare (CVE-2021-1675 and CVE-2021-34527) is a critical vulnerability in the Windows Print Spooler service running on almost every Windows operating system. Dive into a hands-on lab and course where you will exploit and mitigate the vulnerability. Don't get caught unaware by PrintNightmare.
CVE Series
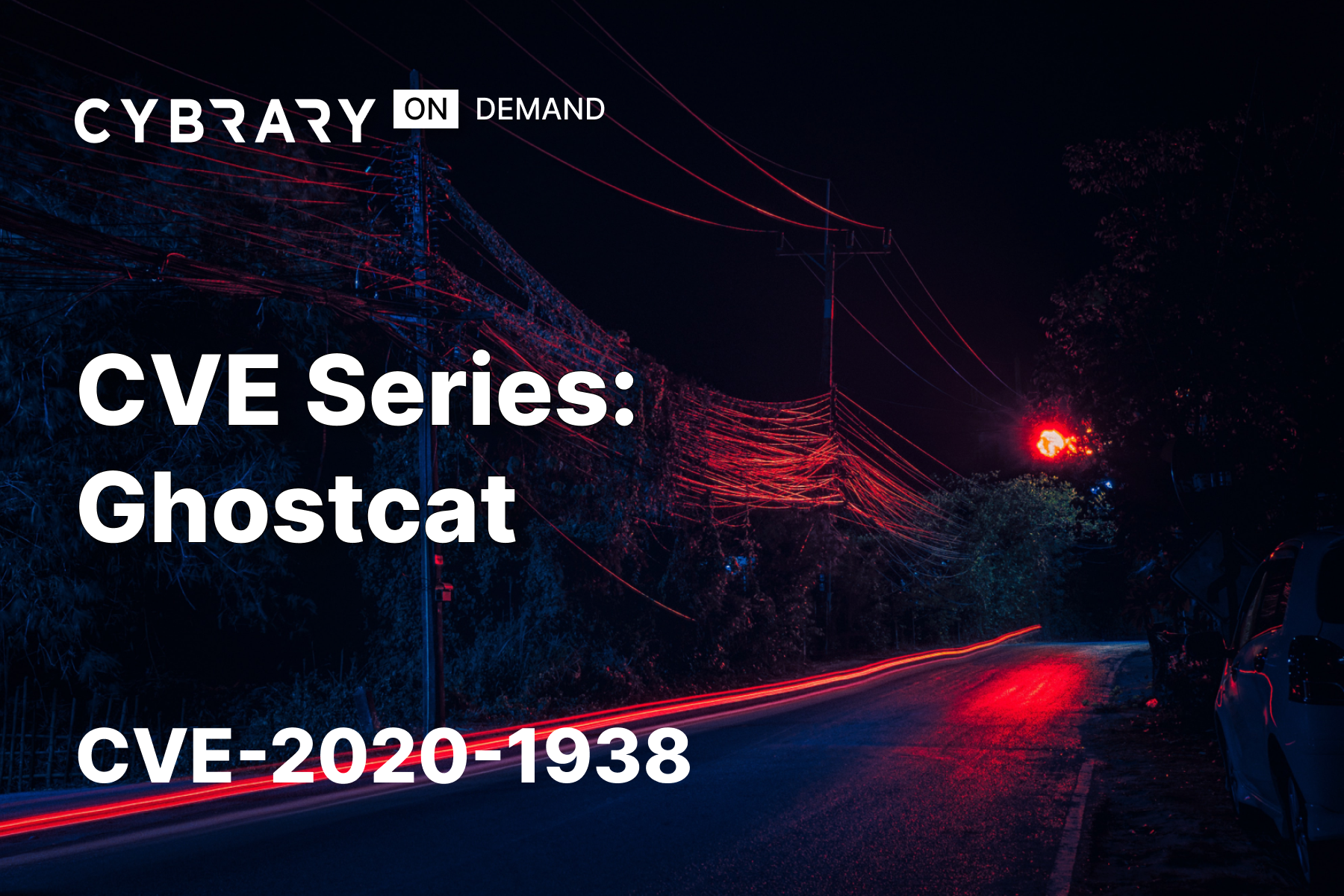
CVE Series: Ghostcat (CVE-2020-1938)
If you're a cybersecurity practitioner who wants to know more about how to exploit and defend against Ghostcat (CVE-2020-1938), the you won't want to miss this course. You will identify the vulnerability, exploit it, and even mitigate it in a hands-on, secure lab environment. Don't let Ghostcat catch you off guard.
CVE Series
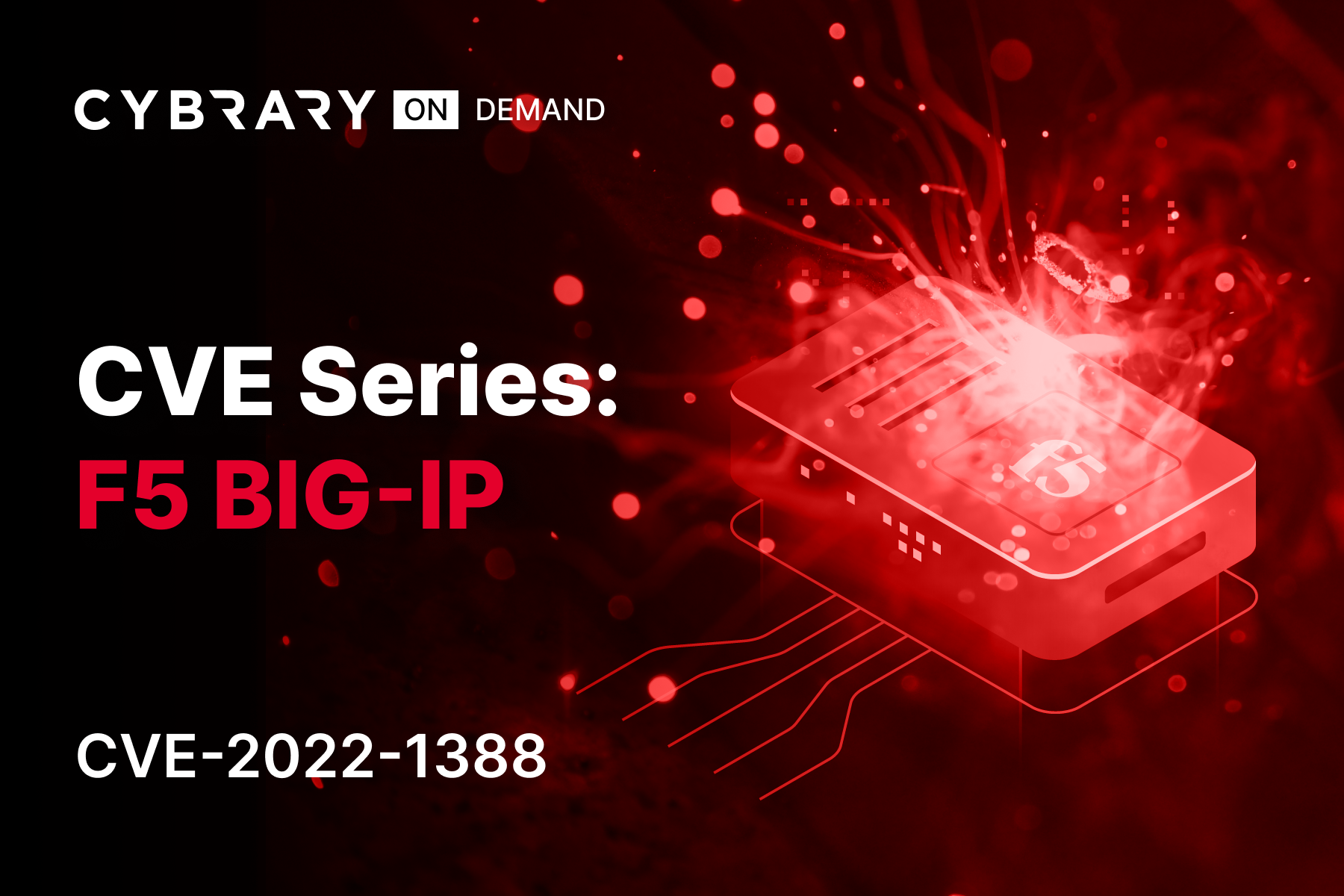
CVE Series: F5 BIG-IP (CVE-2022-1388)
The BIG-IP iControl REST vulnerability (CVE-2022-1388) is a critical flaw that allows unauthenticated attackers to execute system root-level commands remotely. This vulnerability was given a CVSS score of 9.8 due to how easy it is to exploit and the level of access it grants attackers. Learn how to exploit and mitigate this vulnerability today!
CVE Series
CVE Series
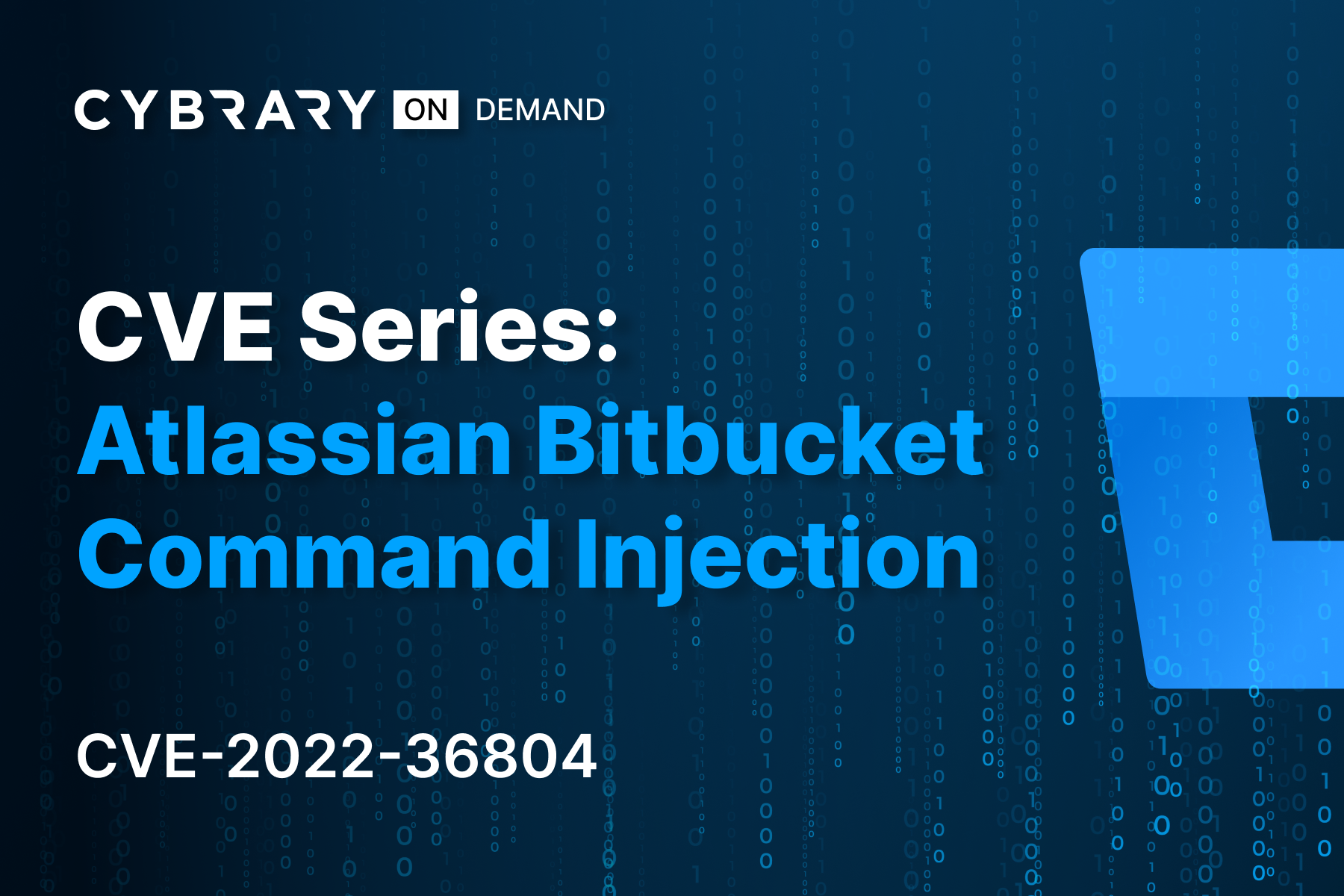
CVE Series: Atlassian Bitbucket Command Injection (CVE-2022-36804)
The Atlassian Bitbucket command injection flaw (CVE-2022-36804) is a remote, unauthenticated, command injection vulnerability affecting application programming interface (API) endpoints in Bitbucket Server and Data Center. Stop an attacker from stealing sensitive information or installing malware as you exploit and mitigate this vulnerability!
CVE Series
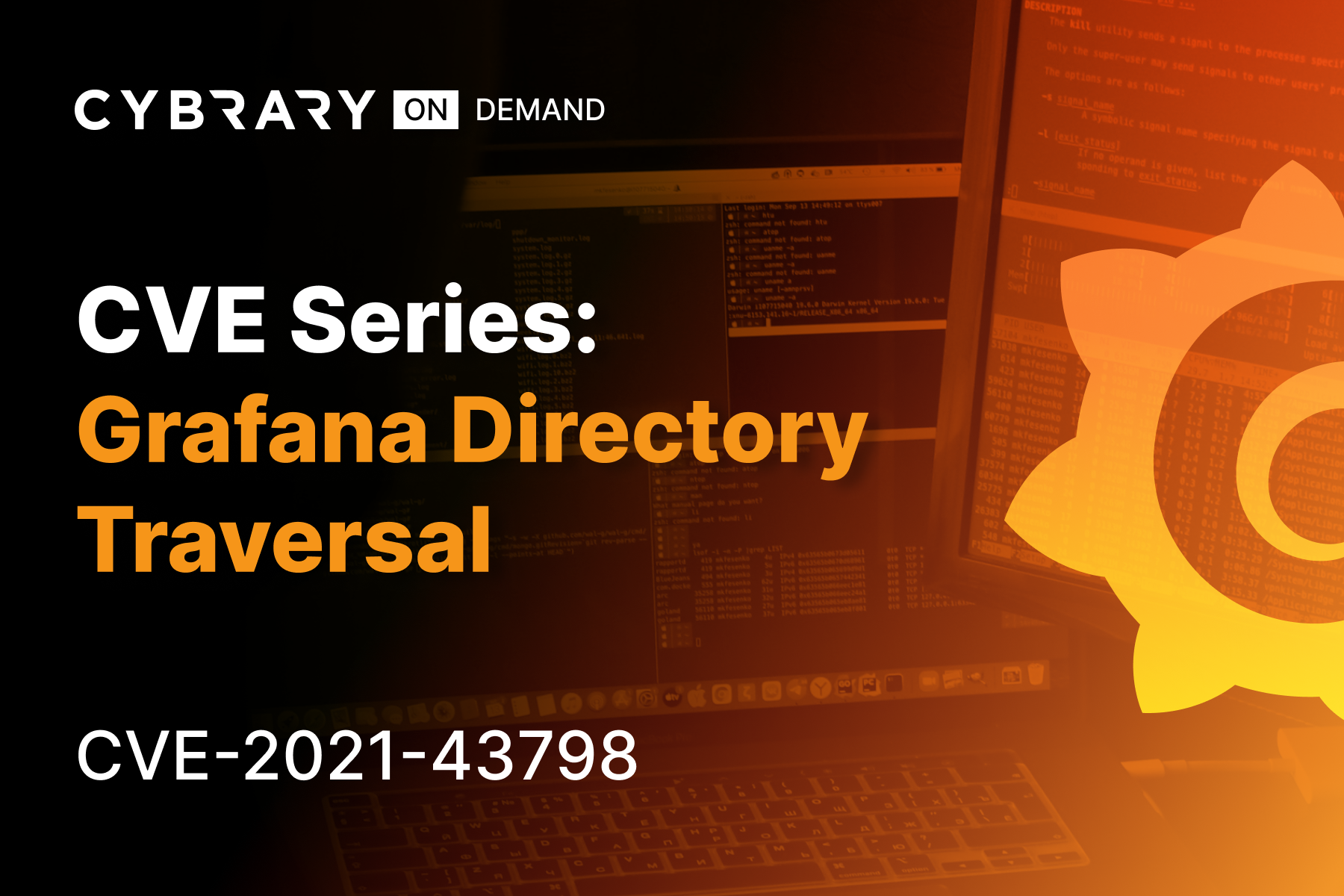
CVE Series: Grafana Directory Traversal (CVE-2021-43798)
The Grafana Directory Traversal vulnerability (CVE-2021-43798) is a critical arbitrary file reading vulnerability impacting global Grafana servers and has been exploited in the wild. Take this course to learn how to exploit and mitigate this vulnerability!
CVE Series

CVE Series: Blind NoSQL Injection (CVE-2021-22911)
The Blind NoSQL Injection vulnerability (CVE-2021-22911) is a critical flaw impacting Rocket.Chat servers across the globe and has been known to be exploited in the wild. Stop an adversary from potentially executing commands on a victim system by learning how to exploit and mitigate this vulnerability!
CVE Series

CVE Series: Apache HTTPD (CVE-2021-42013)
The Apache HTTPD vulnerability (CVE-2021-42013) is a critical flaw impacting servers across the globe. This vulnerability gives an attacker the ability to enumerate a system and execute commands on the victim system if exploited. Exploit and mitigate the vulnerability in a secure lab environment!
CVE Series
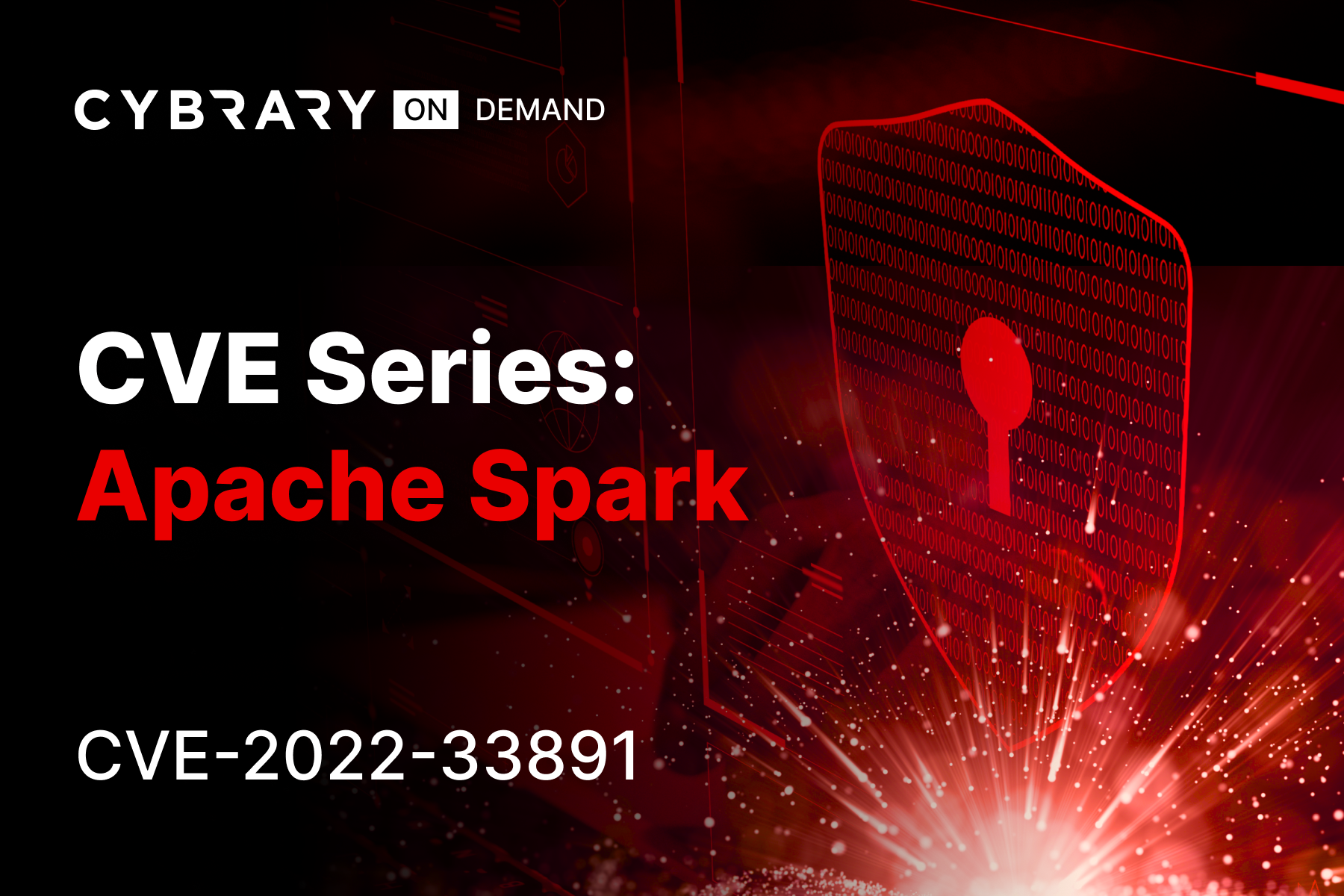
CVE Series: Apache Spark (CVE-2022-33891)
Apache Spark is the biggest open-source project used for large-scale data processing and machine learning. Companies love it for its fast speed and ease of use. But a security flaw allows an adversary to just add a shell command to the URI to perform an arbitrary shell command execution. Exploit this flaw today using two attack vectors!
CVE Series
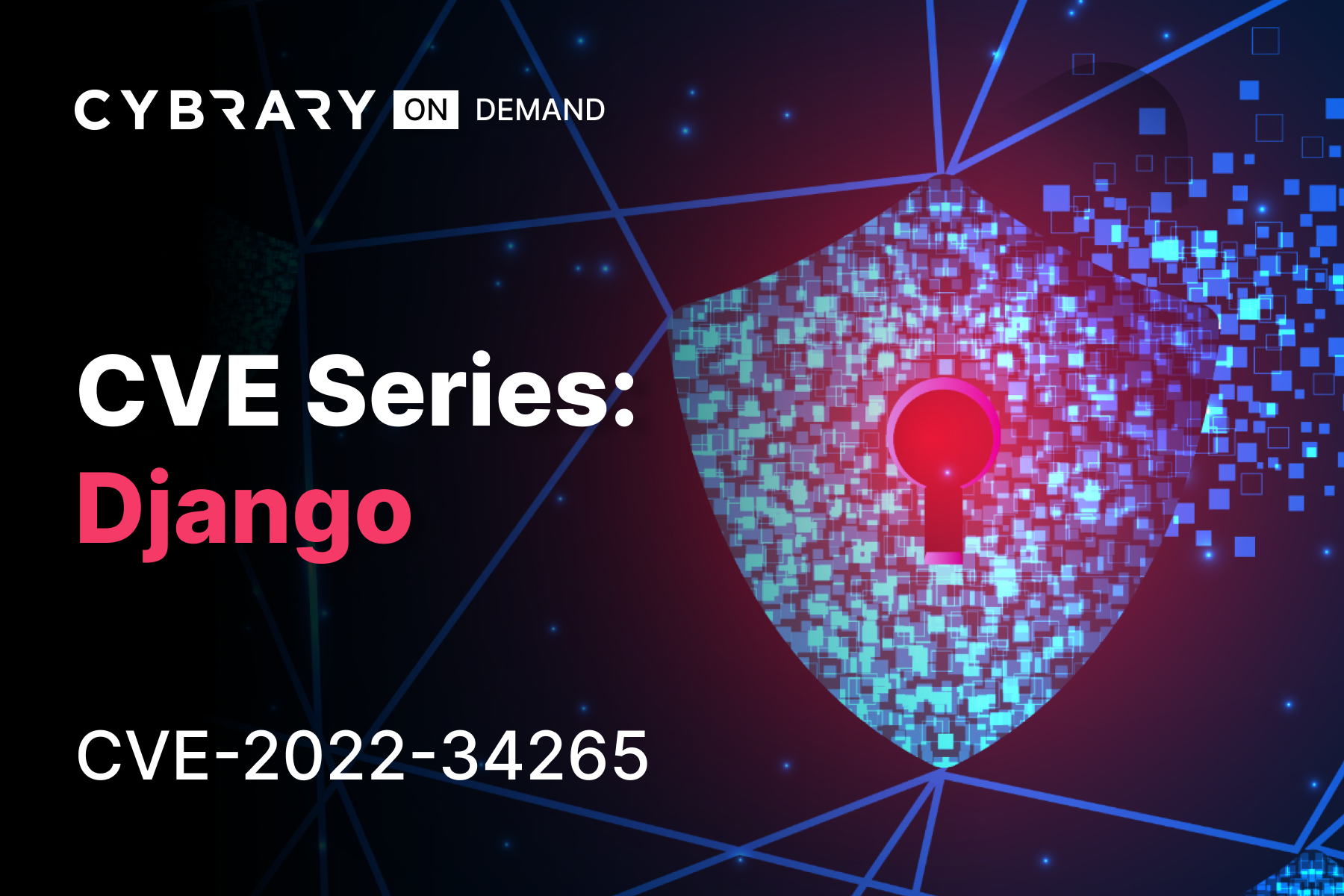
CVE Series: Django (CVE-2022-34265)
Django is a Python-based web framework design for fast, easy application creation. Popular apps like Instagram and Clubhouse use Django, but are you prepared to exploit and mitigate the high-risk Django flaw (CVE-2022-34265) that leaves applications vulnerable to SQL injection attacks? Put your pentest skills to the test in our course!
CVE Series
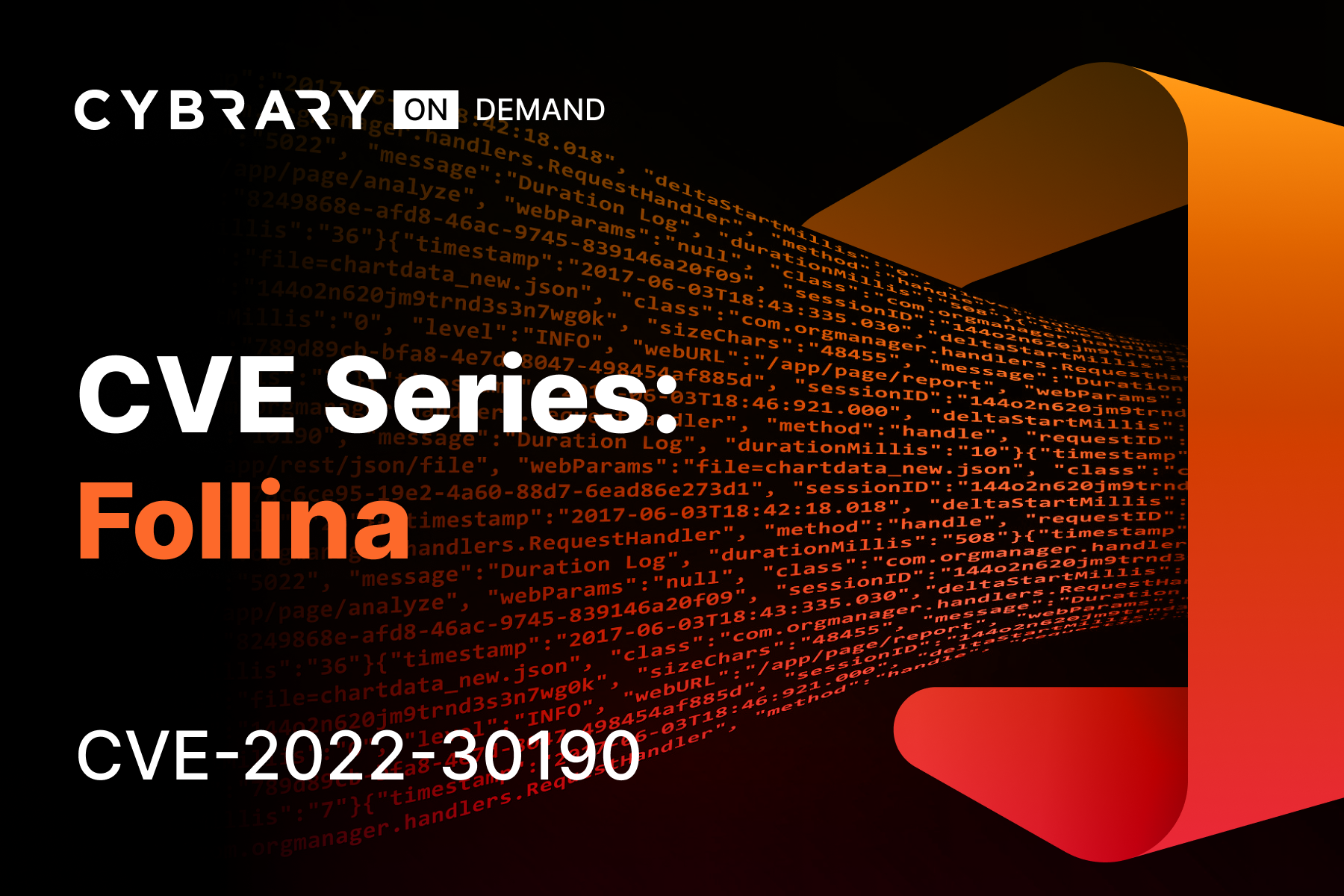
CVE Series: Follina (CVE-2022-30190)
The Follina exploit (CVE-2022-30190) is a Windows Remote Code Execution (RCE) vulnerability that could allow a threat actor to acquire an initial level of access after a successful phishing attack. Take our course to gain the skills you need to identify the vulnerability, detect it, and mitigate it (with current best knowledge).
CVE Series
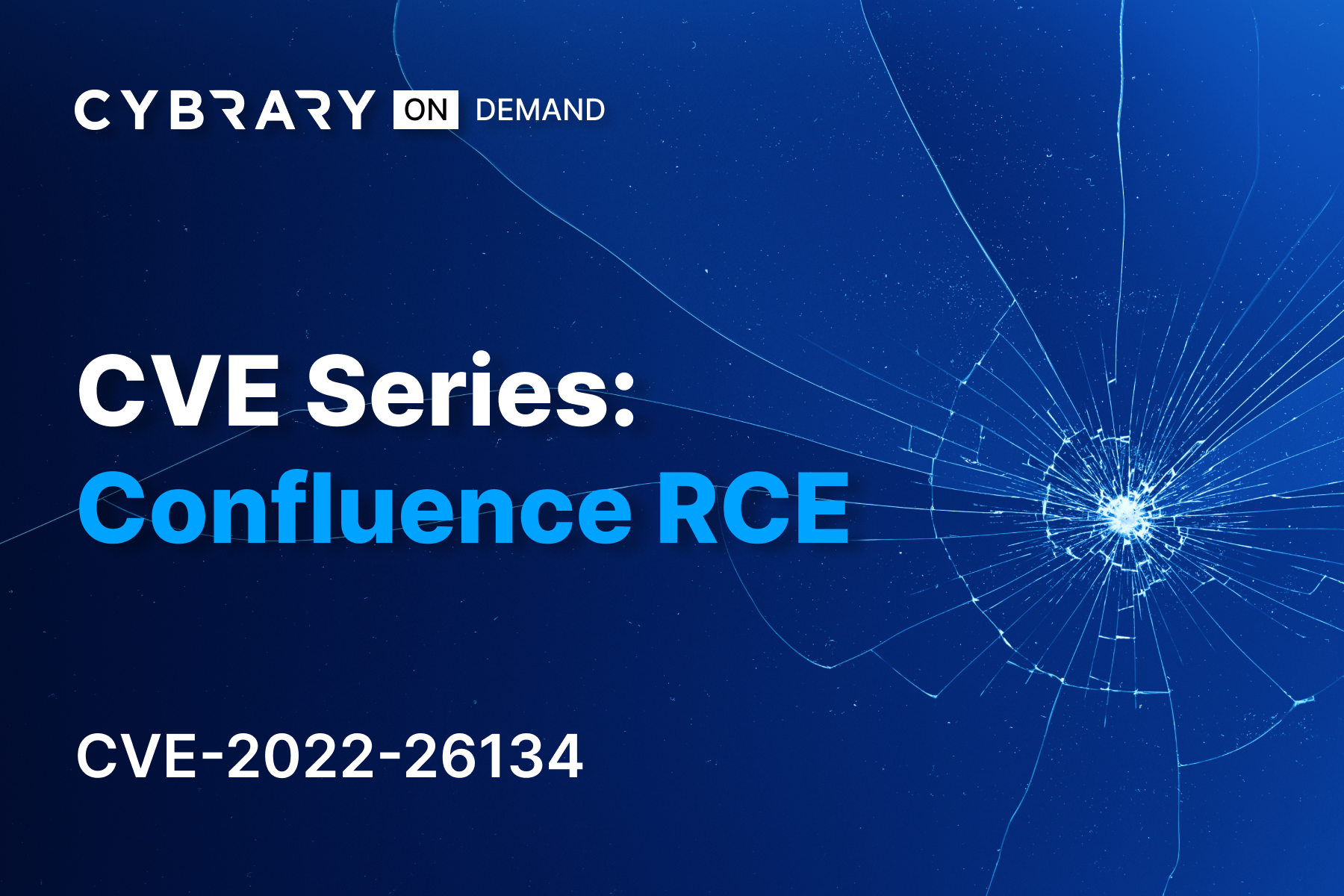
CVE Series: Confluence RCE (CVE-2022-26134)
CVE-2022-26134 is an Object-Graph Navigation Language (OGNL) injection flaw impacting Atlassian Confluence & Data Center software. Leveraging this remote code injection (RCE) flaw, adversaries can execute arbitrary code on a server. Atlassian tools are popular with more remote work, so mitigation is key. Exploit, detect, & mitigate this flaw!
CVE Series

CVE Series: OpenSSL Infinite Loop (CVE-2022-0778)
The OpenSSL infinite loop vulnerability (CVE-2022-0778) is a critical flaw impacting systems running OpenSSL versions 1.0.2, 1.1.1 and 3.0. If exploited, this vulnerability allows adversaries to perform a denial-of-service (DOS) attack. Take our course to exploit this vulnerability in a secure lab environment.
CVE Series
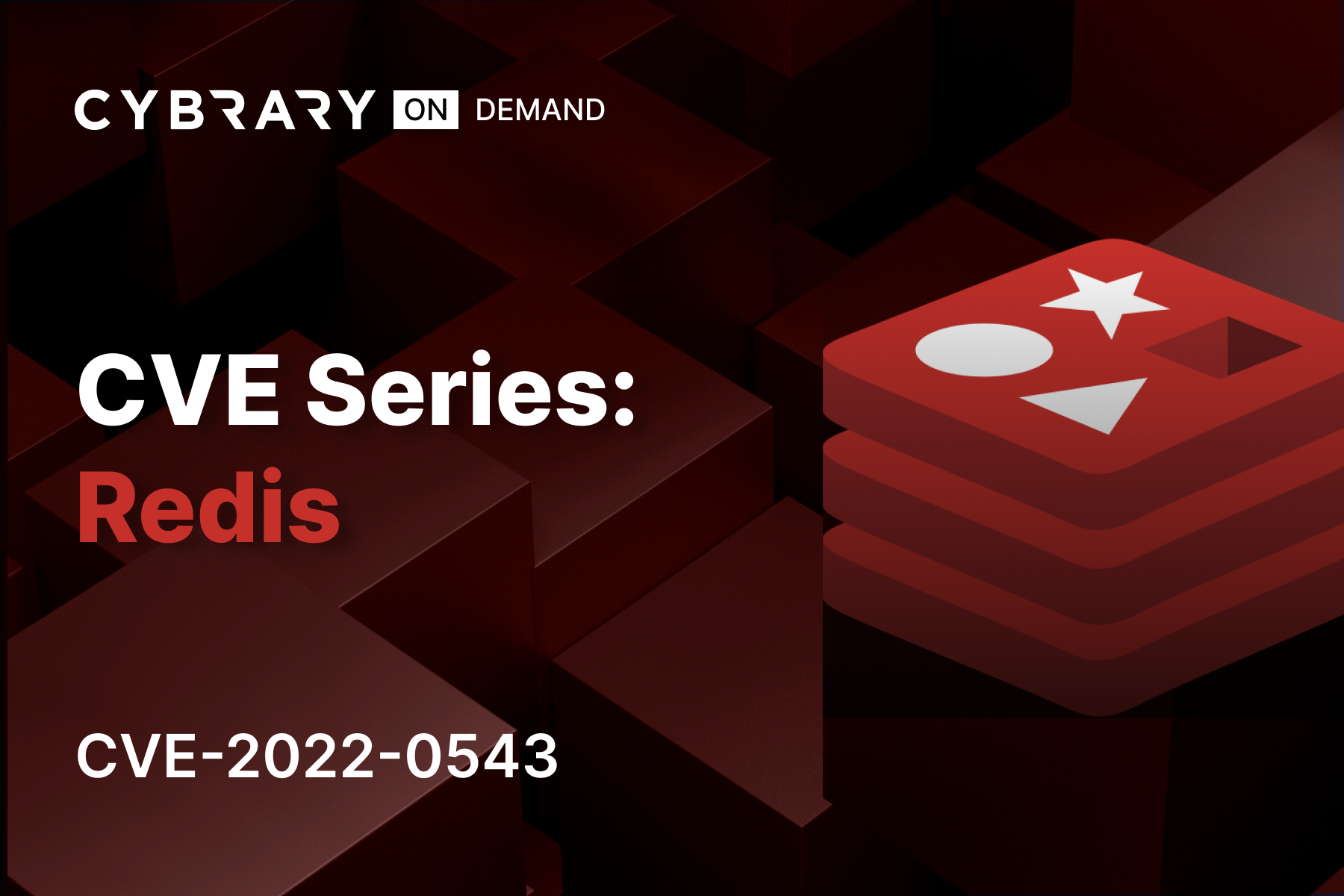
CVE Series: Redis (CVE-2022-0543)
The Redis vulnerability (CVE-2022-0543) is a critical flaw impacting Linux systems across the globe. By exploiting this vulnerability, any user can remotely execute commands as a root user on a system. Take our course to exploit and mitigate the vulnerability in a secure lab environment, giving you the skills you need to protect your organization.
CVE Series

CVE Series: Spring4Shell (CVE-2022-22965)
Spring4Shell (CVE-2022-22965) is a critical Remote Code Execution (RCE) vulnerability affecting Spring, a common application framework library used by Java developers. You will exploit and mitigate this vulnerability in a virtual lab, giving you the skills you need to “Spring” into action and protect your organization!
Threat Actor Campaigns
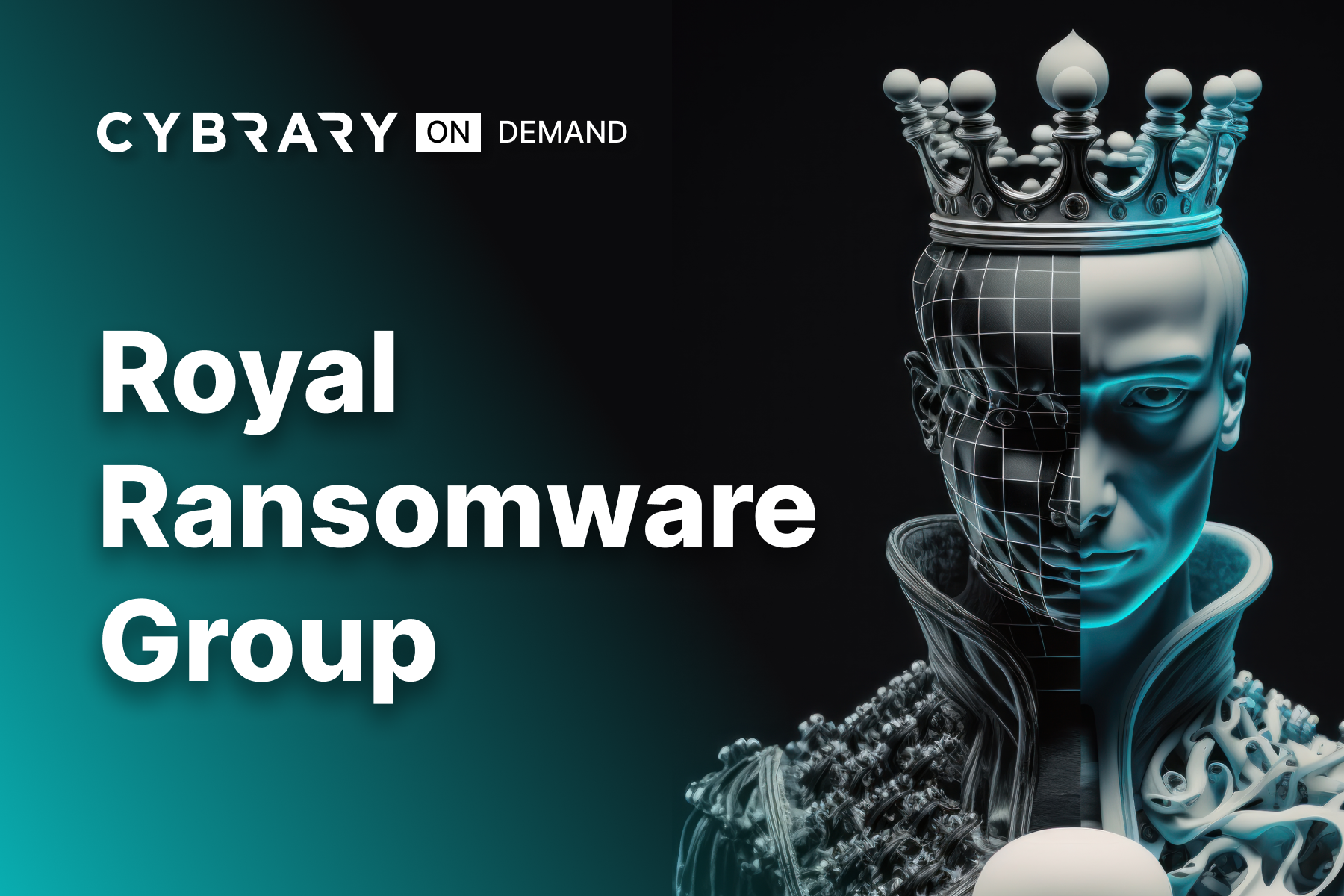
Royal Ransomware Group
Royal is a spin-off group of Conti, which first emerged in January of 2022. The group consists of veterans of the ransomware industry and brings more advanced capabilities and TTPs against their victims. Begin this campaign to learn how to detect and protect against this newer APT group!
Threat Actor Campaigns
Threat Actor Campaigns
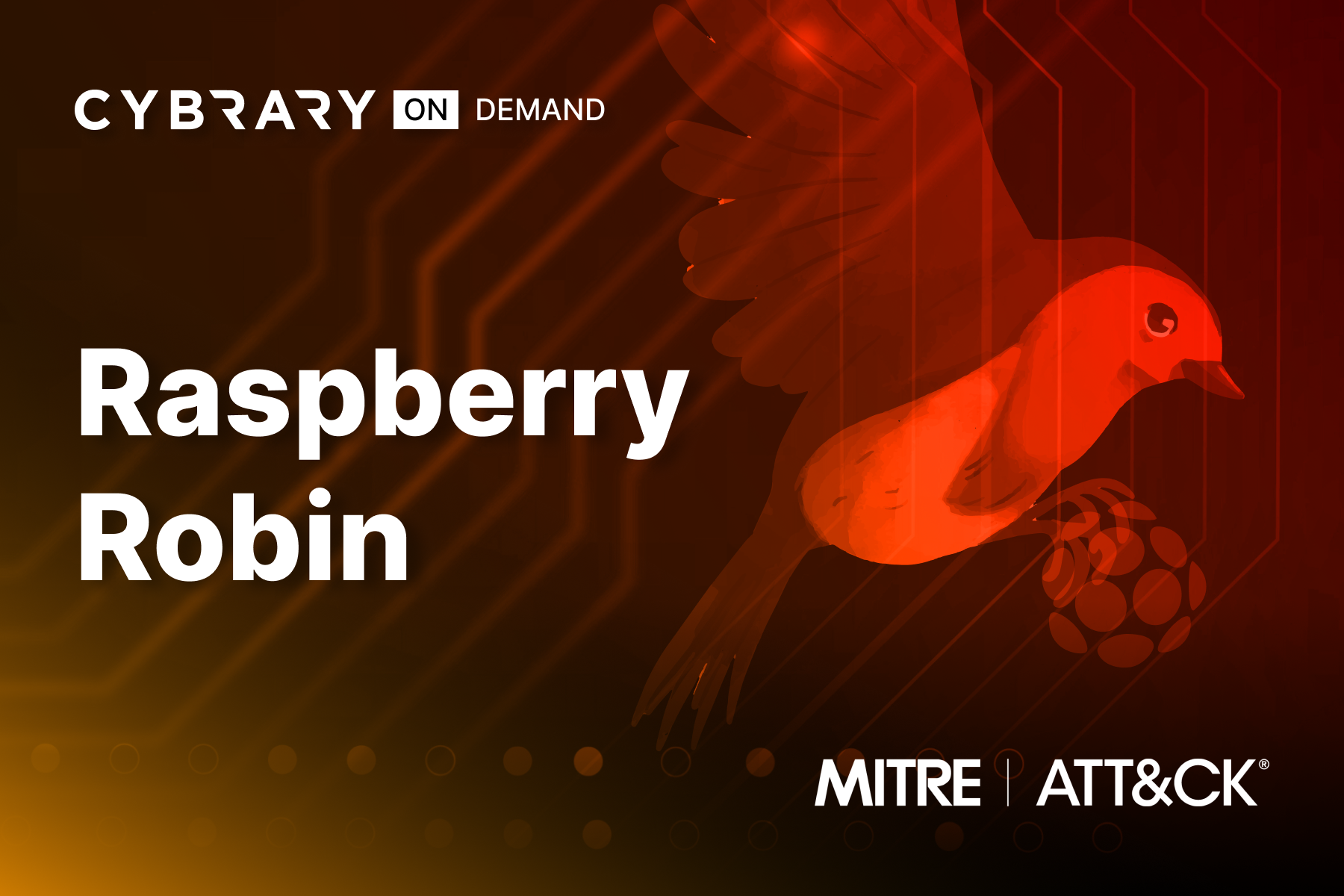
Raspberry Robin
Raspberry Robin is a malware family that continues to be manipulated by several different threat groups for their purposes. These threat actors (Clop, LockBit, and Evil Corp) specialize in establishing persistence on a compromised host and creating remote connections to use later. Once established, these C2 connections can be used for multiple purposes, including data exfiltration, espionage, and even further exploitation.
Threat Actor Campaigns
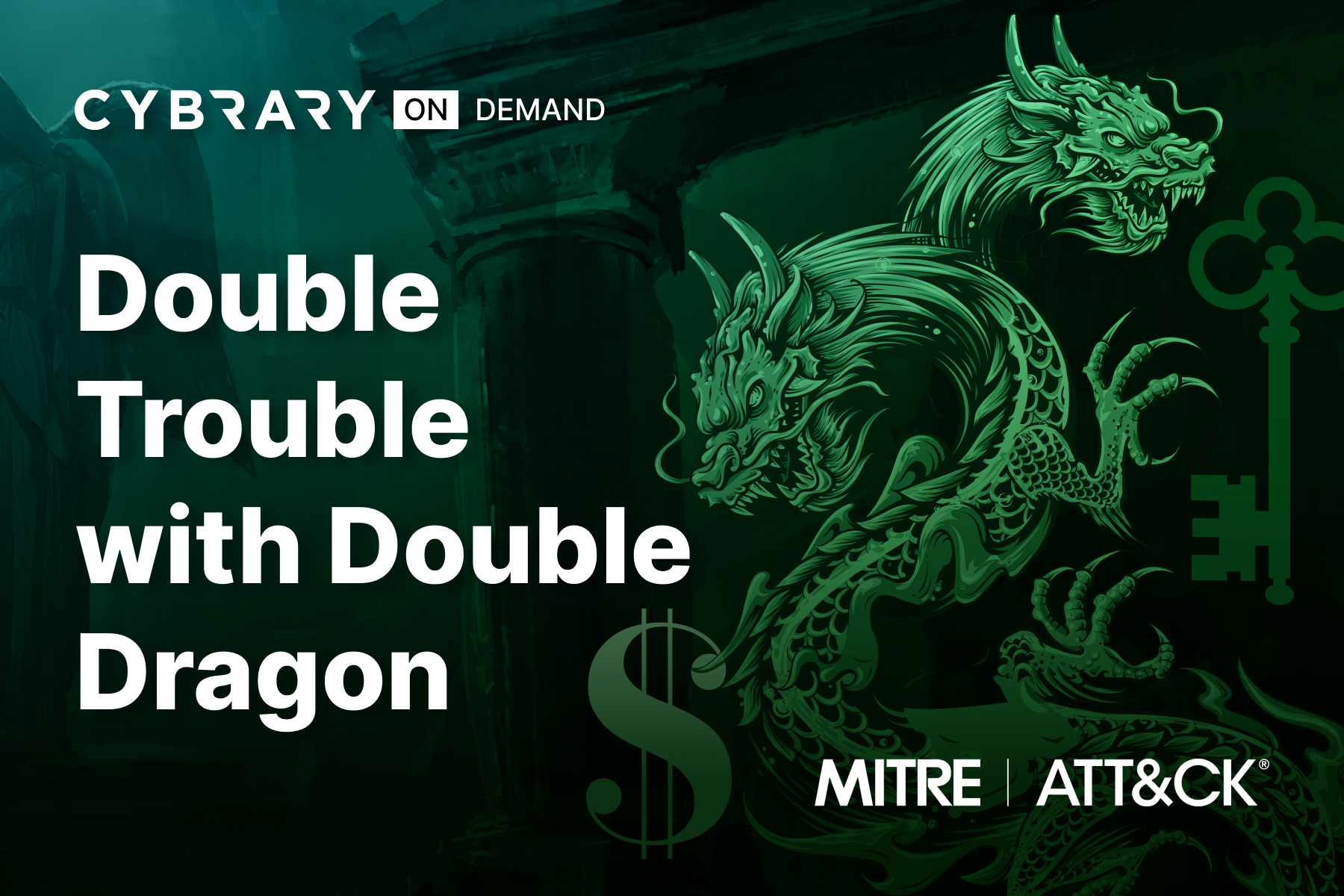
Double Trouble with Double Dragon
Advanced Persistent Threats (APTs) conduct state-sponsored cyberattacks that can radically disrupt global business operations. Launch this campaign to start detecting sophisticated techniques leveraged by APT41, known as "Double Dragon" because they cause double trouble with both espionage and financially-motivated attacks!
Threat Actor Campaigns
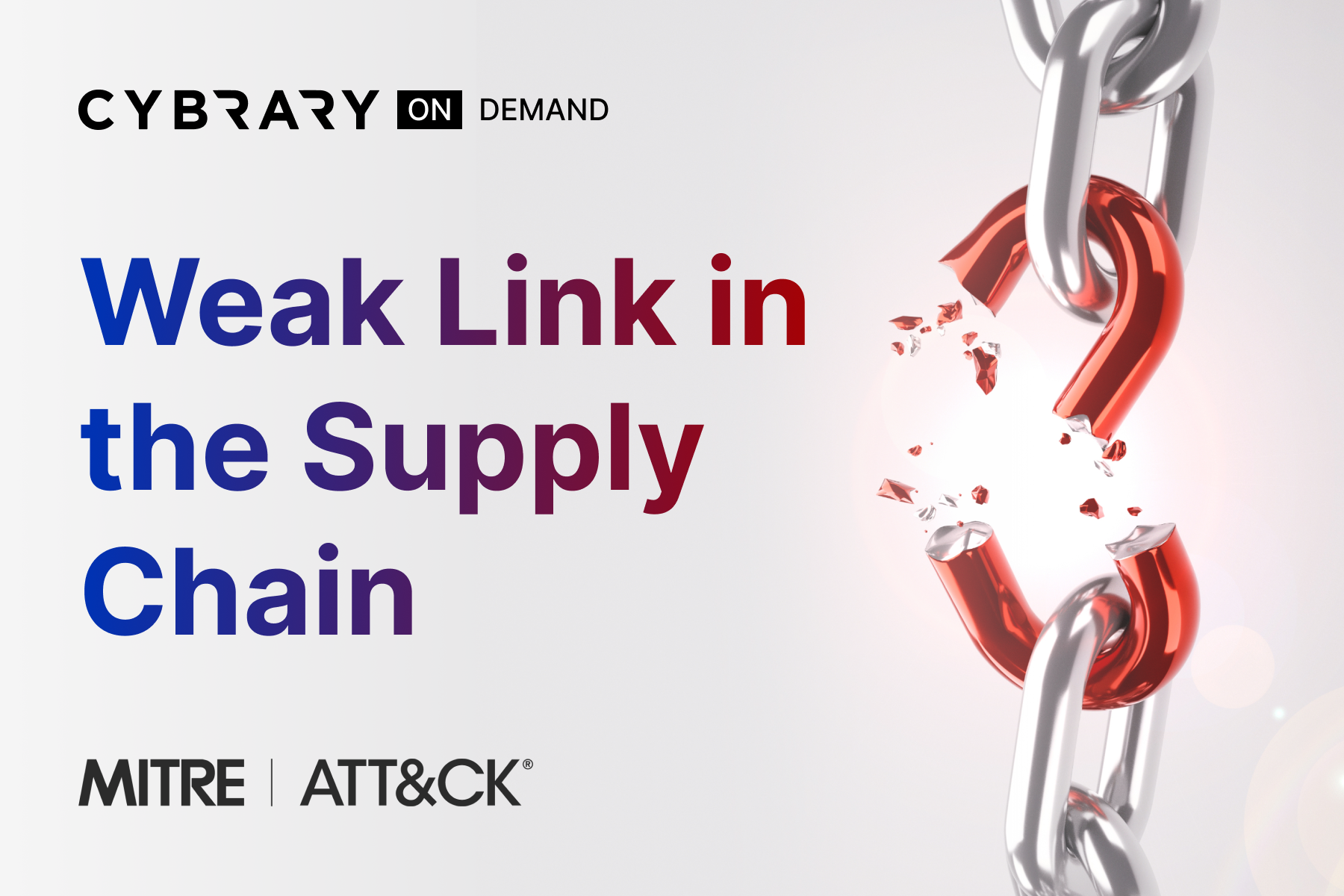
Weak Link in the Supply Chain
Threat actors known as Advanced Persistent Threats (APTs) conduct highly sophisticated attacks sponsored by nation-states. They maintain a committment to stealth and often use custom malware. This campaign emulates a supply chain attack by APT29 that is similar to the SolarWinds compromise and it has the end goal of stealing sensitive information.
Threat Actor Campaigns

Spinning a Web Shell for Initial Access
Certain threat actors specialize in targeting vulnerable web servers and gain initial access by exploiting public-facing applications. Then they act as access brokers for ransomware gangs. Such campaigns highlight the need to protect against known vulnerabilities. Understanding these techniques is key to protecting your organization.
Threat Actor Campaigns
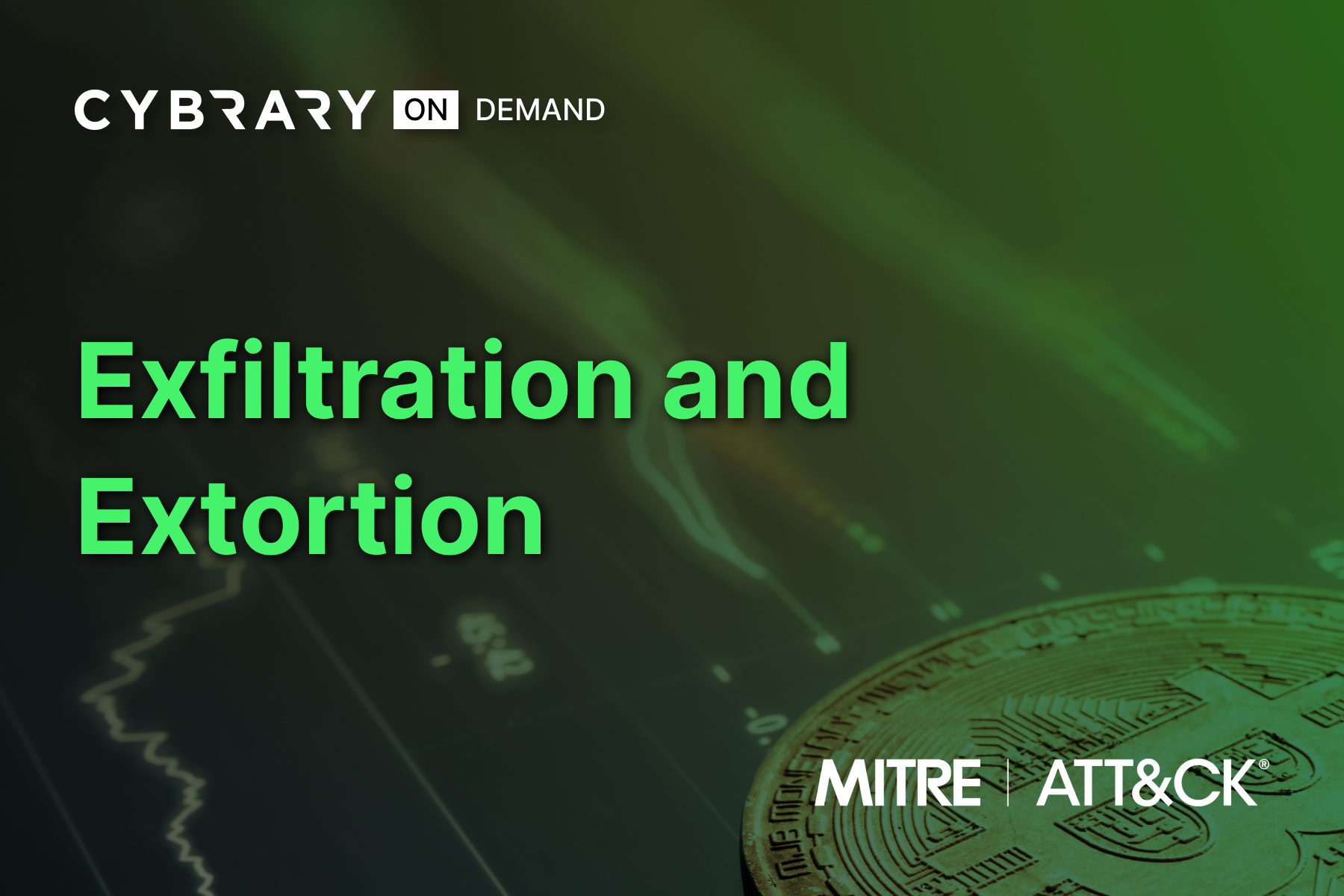
Exfiltration and Extortion
Threat actors will use stolen data exfiltrated from victim systems to extort organizations. Once they gain a foothold, they delete critical system files and threaten to release the data or disrupt operations if the victims do not pay up. Understanding these techniques is vital to defending your organization from such attacks.
Threat Actor Campaigns
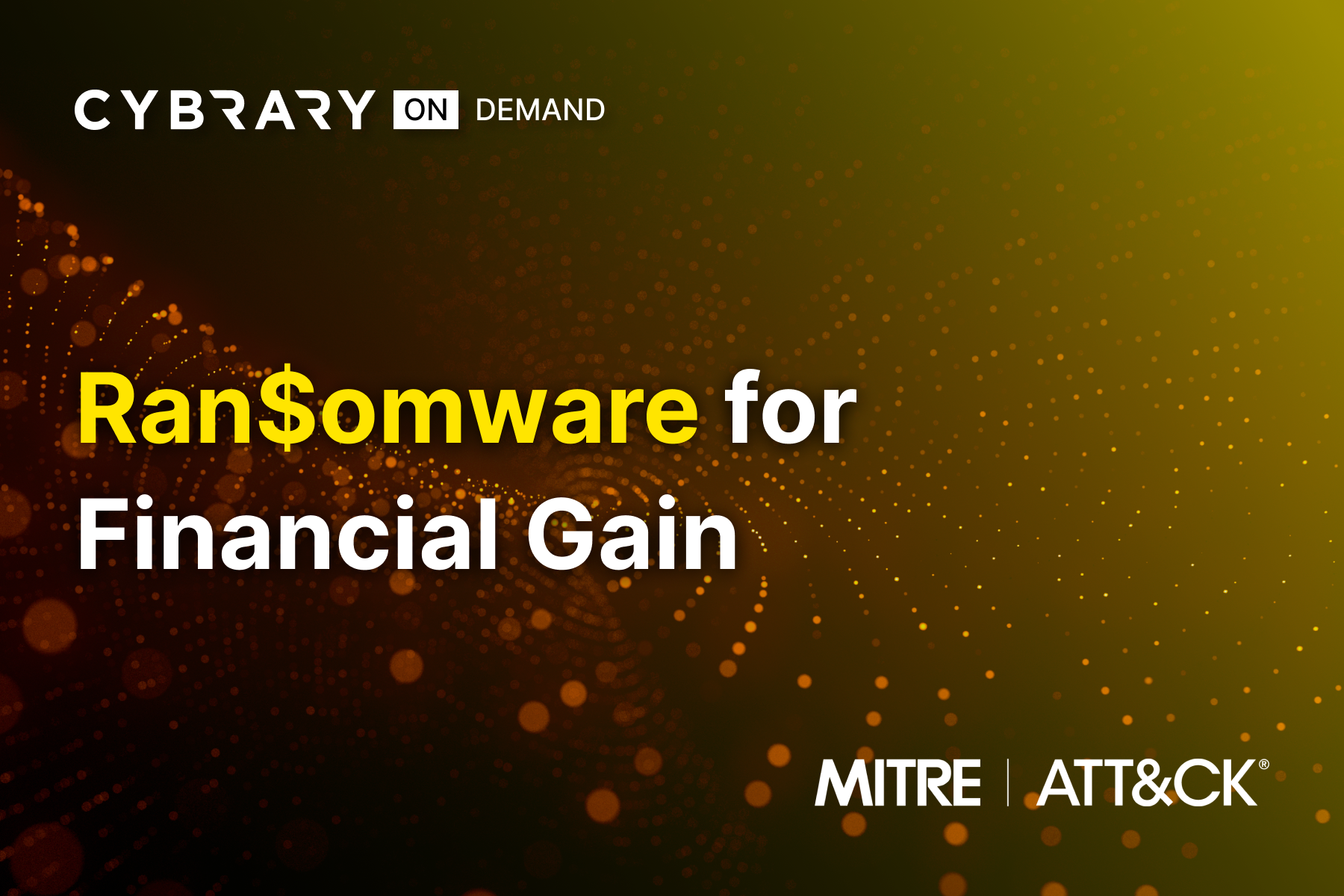
Ransomware for Financial Gain
Threat actors continue to leverage ransomware to extort victim organizations. What was once a simple scheme to encrypt target data has expanded to include data disclosure and targeting a victim’s clients or suppliers. Understanding the techniques threat actors use in these attacks is vital to having an effective detection and mitigation strategy.
Instructors
Industry Seasoned. Cybrary Trained. Our instructors are current industry professionals trained by Cybrary to ensure consistency in quality and content.