Browse the Full Catalog
Cybrary’s comprehensive, framework-aligned catalog has been reorganized to provide you with an intentional, guided learning experience. Advance your career, prep for certifications, and build your skills whenever, wherever.

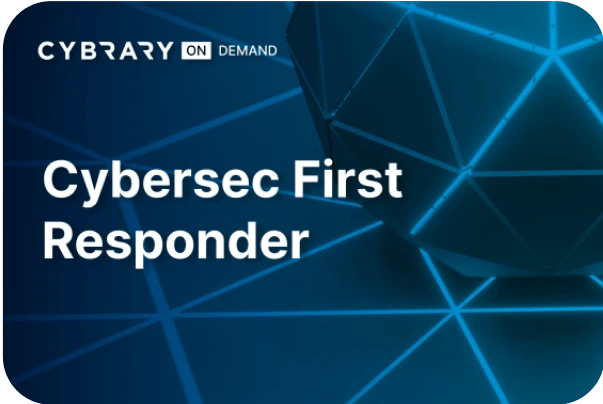


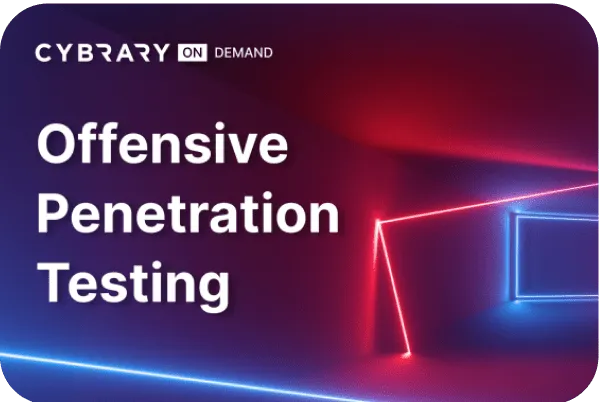



The content and tools you need to build real-world skills
Rapidly develop your skills via an integrated and engaging learning
experience on the Cybrary platform.
Bite-sized Video Training
Manageable instruction from industry experts
Hands-On Learning
Put your skills to the test in virtual labs, challenges, and simulated environments
Practice Exams
Prepare for industry certifications with insider tips and practice exams
Earn Industry Badges
Complete coursework to earn industry-recognized badges via Credly

CISSP Certification Prep
Our CISSP certification course and training for the CISSP certification, essential for cybersecurity professionals aiming to move up in their career. This course will cover all aspects of security, risk management, and architecture to help you prepare for the CISSP exam. Learn from experienced professionals and gain the knowledge needed to become a certified security expert.

Comptia Security+ Certification Prep
CompTIA’s Security+ is a globally recognized certification that equips IT professionals with cybersecurity principles and security best practices, and is often used as a requirement for entry-level cybersecurity positions. This certification prep path is designed to provide you with a comprehensive overview of the concepts and skills you will need to pass the certification exam.
.webp)
IT & Cybersecurity Foundations Career Path
Get everything you need to start your cybersecurity career journey, and stop wasting your time (and money) sorting through unreliable information from questionable sources. While this industry offers very lucrative career opportunities, finding accurate, relevant information to break into the field can be incredibly frustrating. That’s why we’ve crafted this IT and Cybersecurity Foundations path, featuring thoughtful, bite-sized content from expert instructors covering core concepts found across all cybersecurity roles. These instructors have helped thousands of other beginners grasp essential IT and cybersecurity topics. Get ready to take your first steps into cybersecurity by diving into core concepts needed for any cybersecurity role. Build practical skills and gain confidence as you begin your cybersecurity journey.
.webp)
SOC Analyst Career Path: Training for Advancement
SOC Analysts are the front line of defensive security operations and currently among the most in-demand roles in cybersecurity. They are responsible for receiving, analyzing, and triaging security tickets within an organization’s Security Operations Center. This SOC analyst training path is designed to equip you with the foundational knowledge and key skills needed to succeed in an entry-level SOC Analyst role. Through a series of 20 courses and hands-on virtual labs, you’ll gain experience in Defensive Security Fundamentals, Log Analysis, Network-Based Detection, and Host-Based Detection. Completing this SOC analyst training, along with the associated assessments, will prepare you to launch your career and explore advanced specialties like Threat Intelligence, Threat Hunting, Incident Response, Malware Analysis, and Digital Forensics.

Penetration Tester Career Path
Penetration Testers are professional ethical hackers. They are responsible for assessing an organization's defenses from the perspective of a threat actor. This program is designed to provide you with the foundational knowledge and key skills required to succeed as an entry-level Penetration Tester. Over the course of 22 courses and hands-on virtual labs, you will learn Offensive Security Fundamentals, Reconnaissance and Enumeration, Application Attacks, Network Attacks, and Evasion.
.webp)
Security Engineer Career Path
Security Engineers are security-minded architects and builders. They are responsible for planning and implementing security controls throughout an IT environment. This career path is designed to provide you with foundational knowledge and key skills required to succeed as an entry-level Security Engineer. In this path, you will learn Security Engineering Fundamentals, Infrastructure Security, Application Security, and Data Security.
Completing this career path and the associated Assessments will prepare you for pursuing a career as a Security Engineer and further specializing in advanced skills like Cloud Security and Cybersecurity Architecture.
.webp)
Leadership and Management Career Path
Effective Leadership and Management is critical to any security-related function. Leaders and Managers are responsible for providing the vision and advocacy required for an organization to effectively manage cyber risks. This career path is designed to provide you with foundational knowledge and key skills required to succeed as an effective leader within any security domain.

OWASP Top 10 - A08:2021 - Software and Data Integrity Failures

OWASP Top 10 - A04:2021 - Insecure Design
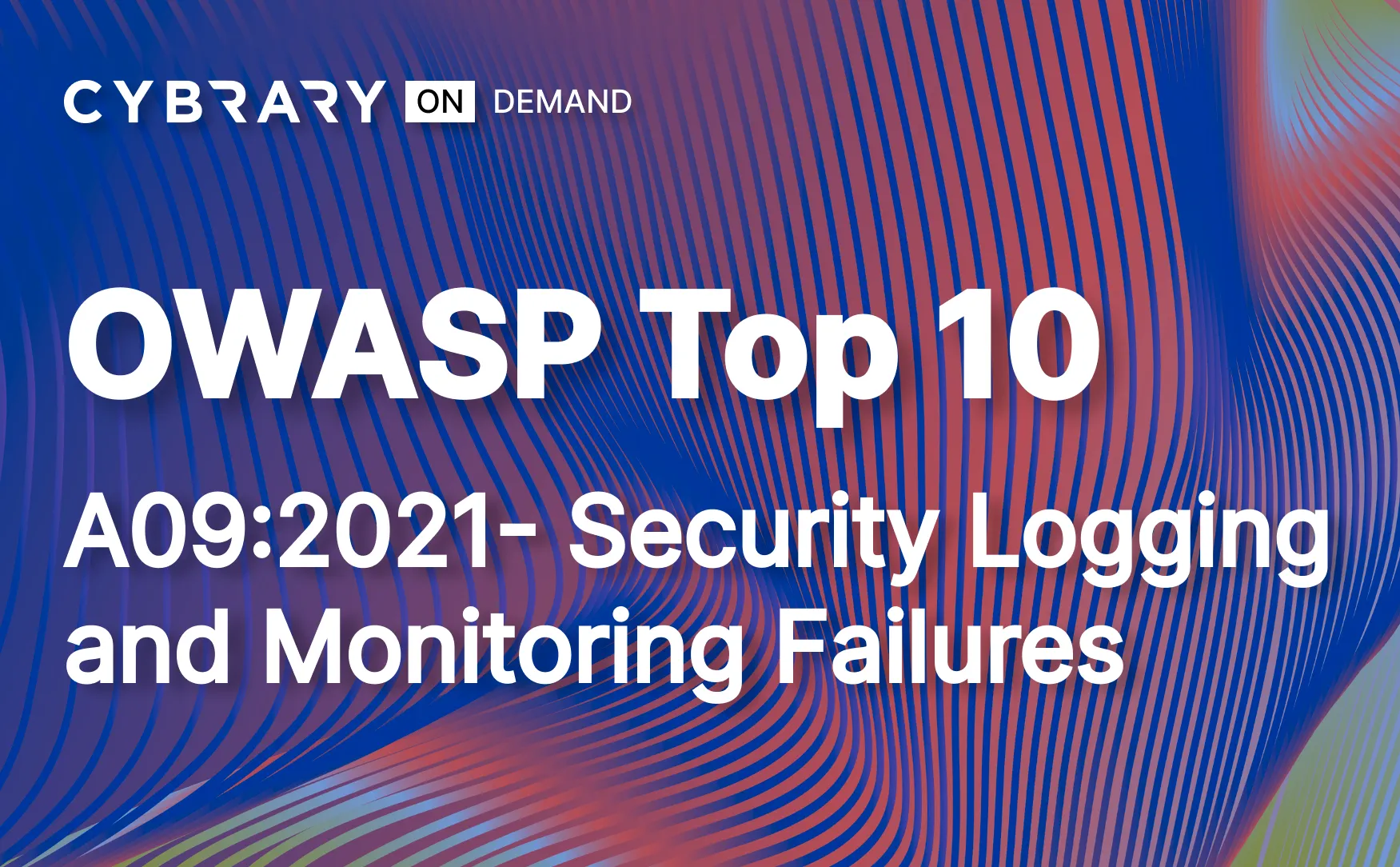
OWASP Top 10 - A09:2021 - Security Logging and Monitoring Failures

OWASP Top 10 - A10:2021 - Server-Side Request Forgery (SSRF)

OWASP Top 10 - A05:2021 - Security Misconfiguration

OWASP Top 10 - A02:2021 - Cryptographic Failures

OWASP Top 10 - A07:2021 - Identification and Authentication Failures

OWASP Top 10 - A06:2021 - Vulnerable and Outdated Components
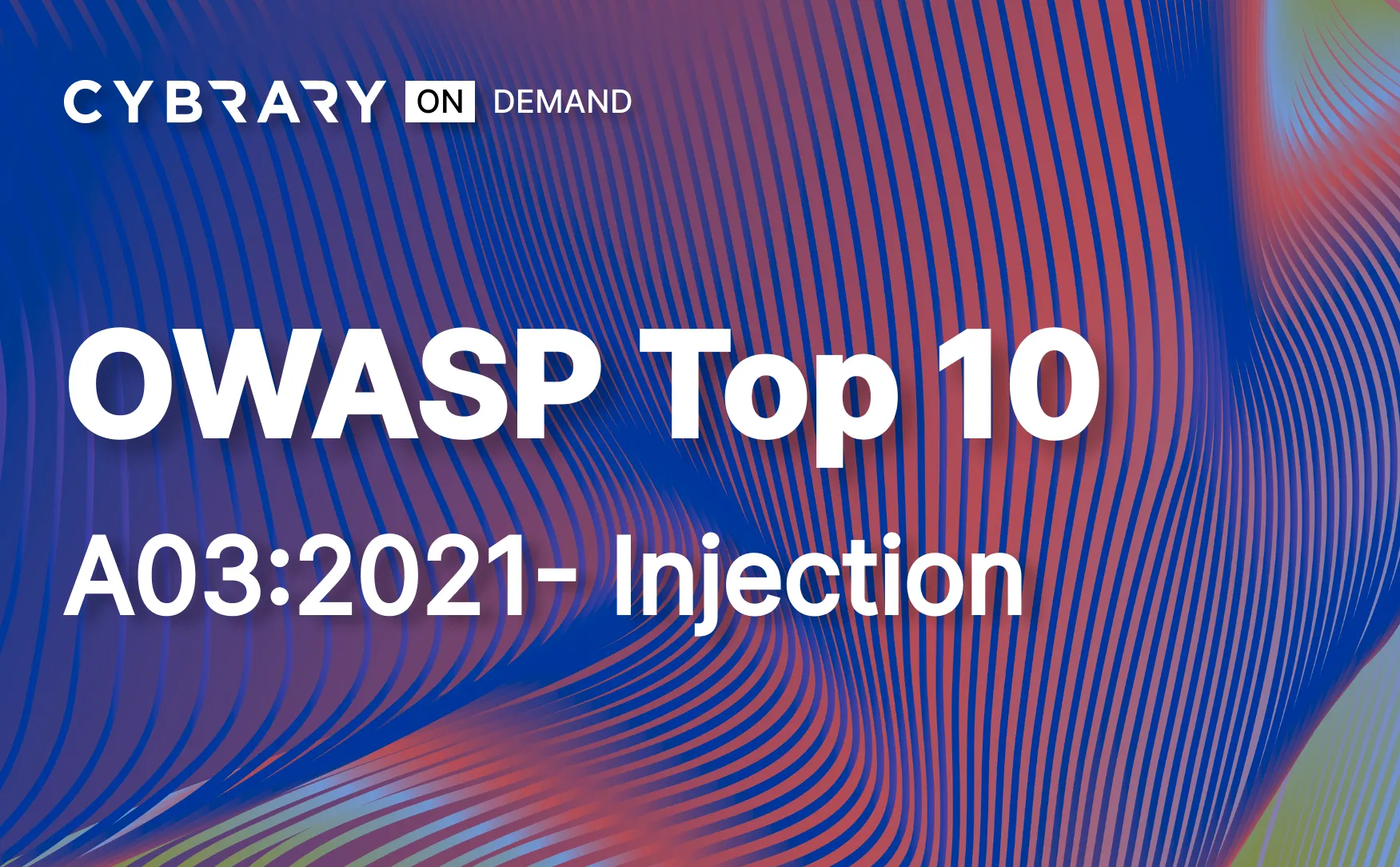
OWASP Top 10 - A03:2021 - Injection

OWASP Top 10 - A01:2021 - Broken Access Control

OWASP Top 10 - A04:2017 - XML External Entities

Protocol Tunneling

Exfiltration Over Alternative Protocol: Asymmetric Encrypted Non-C2 Protocol
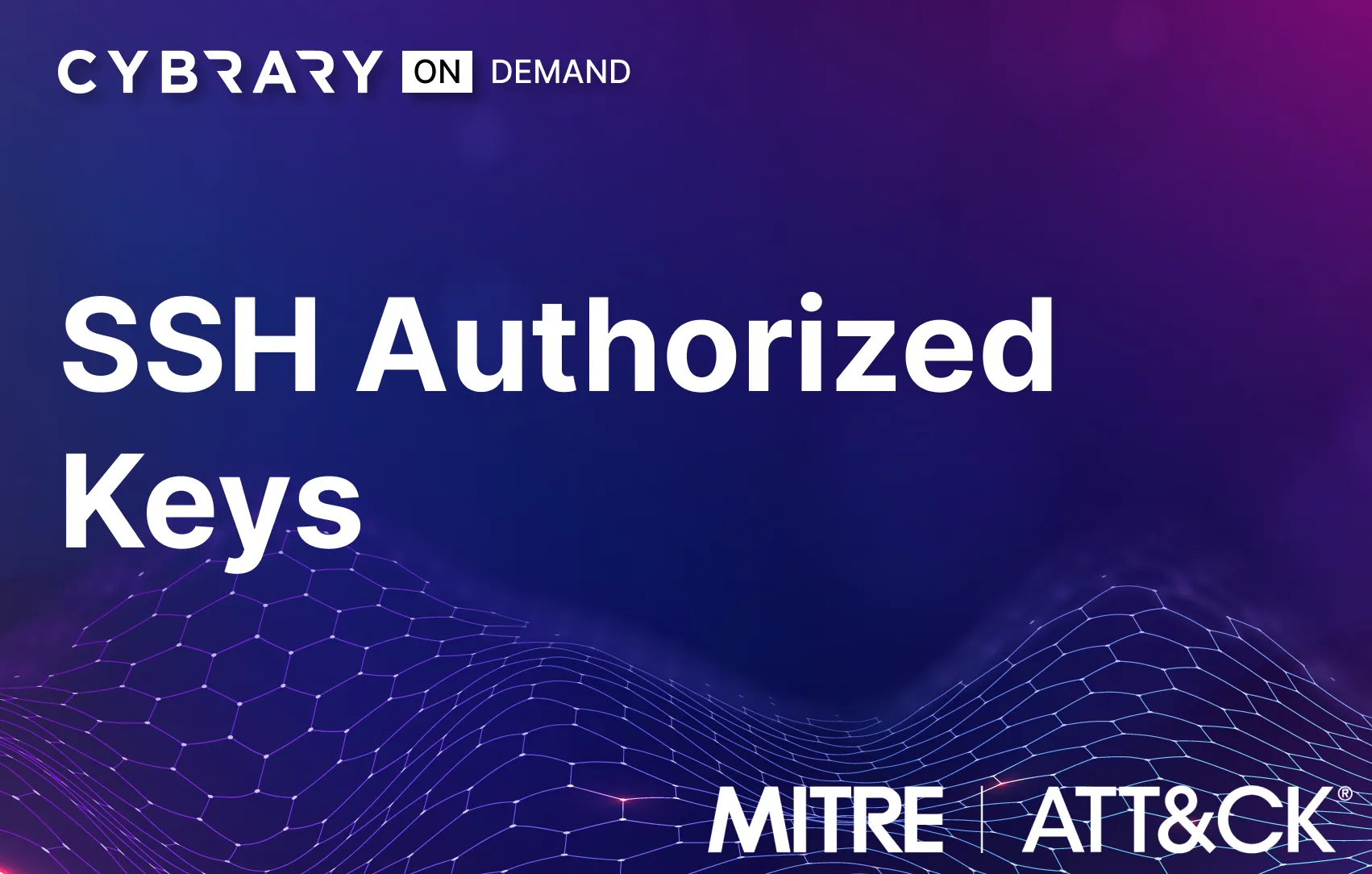
SSH Authorized Keys
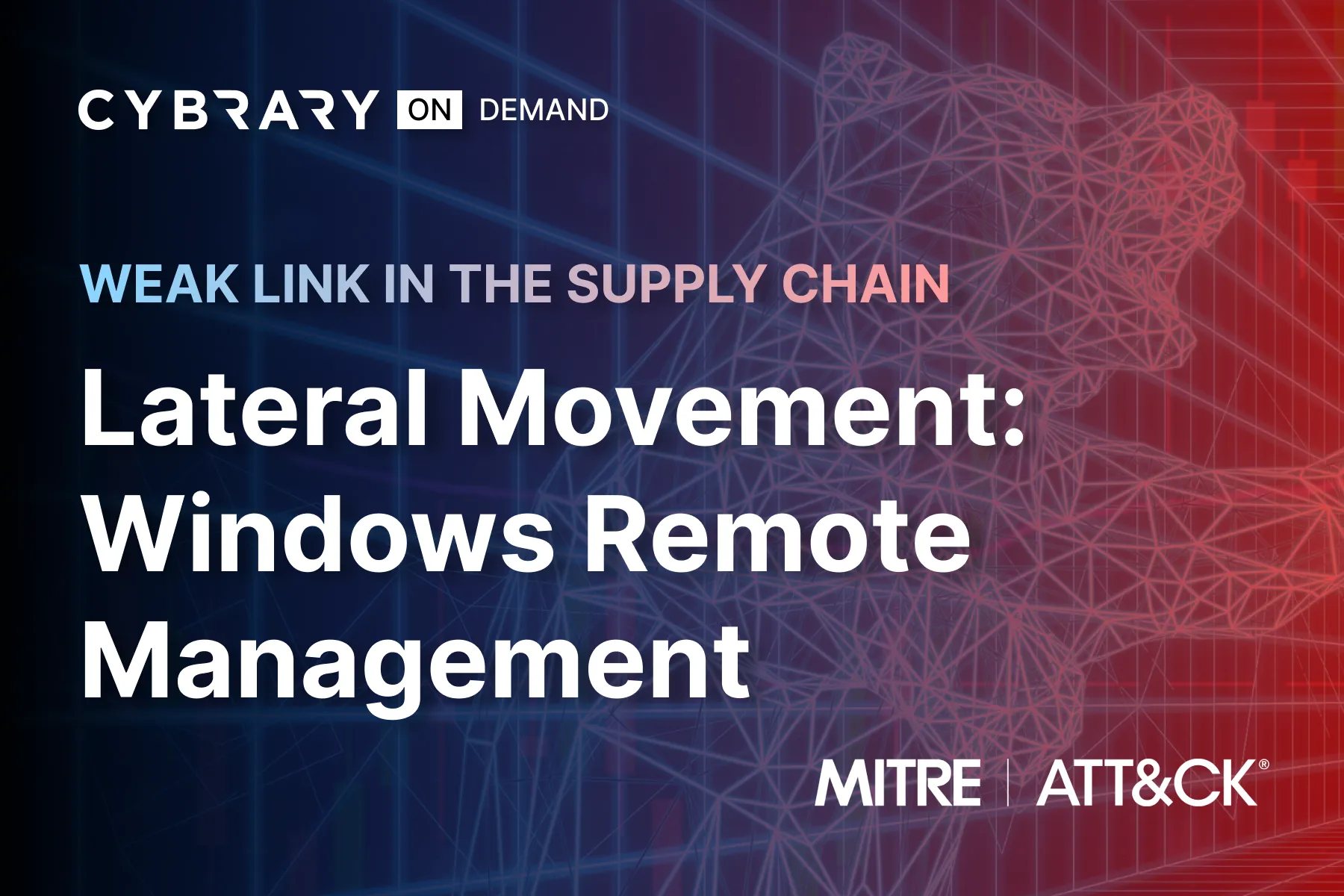
Lateral Movement: Windows Remote Management
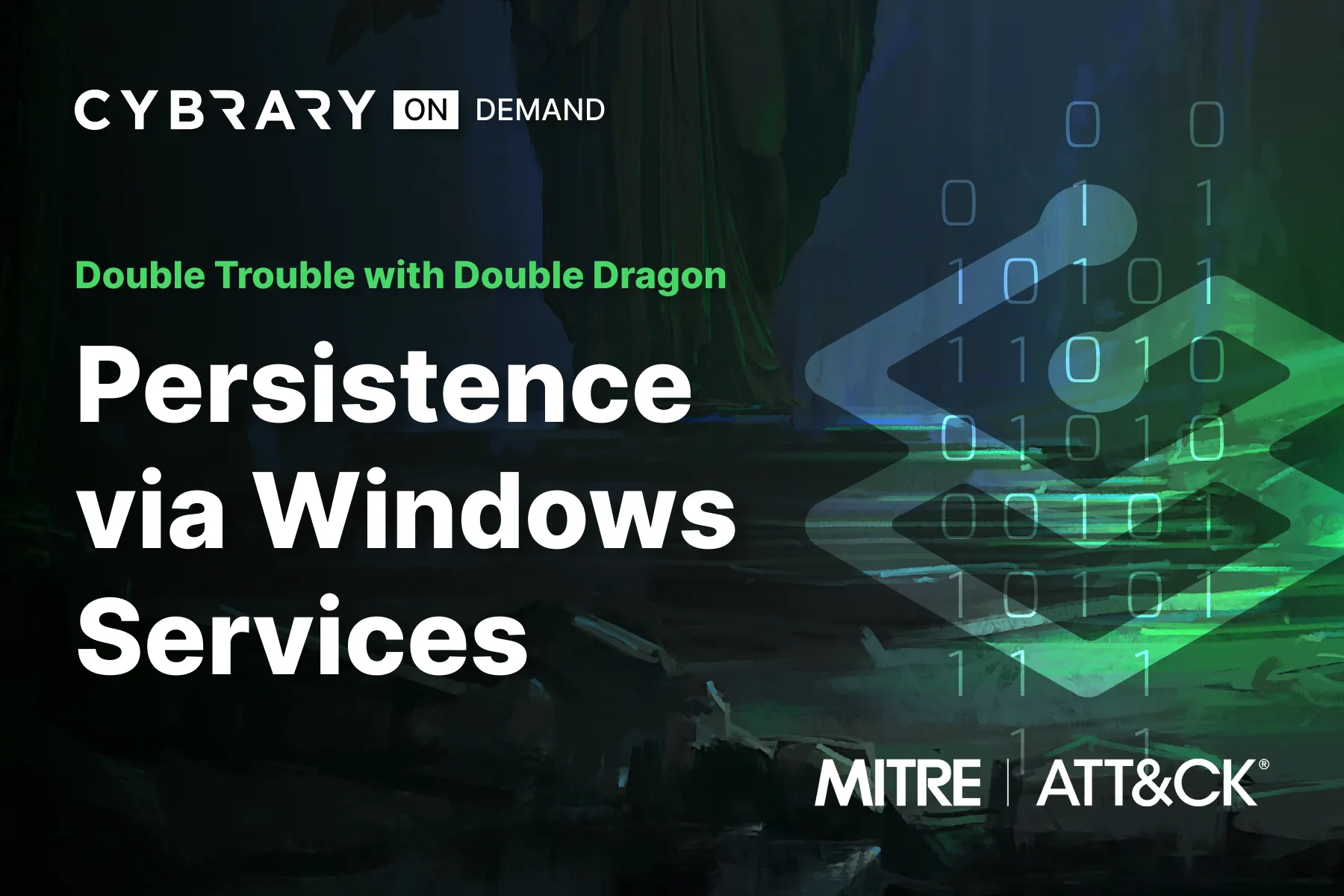
Persistence via Windows Services

System Binary Proxy Execution and a Spearphish Payload

Server Software Component: Web Shell

Scheduled Task
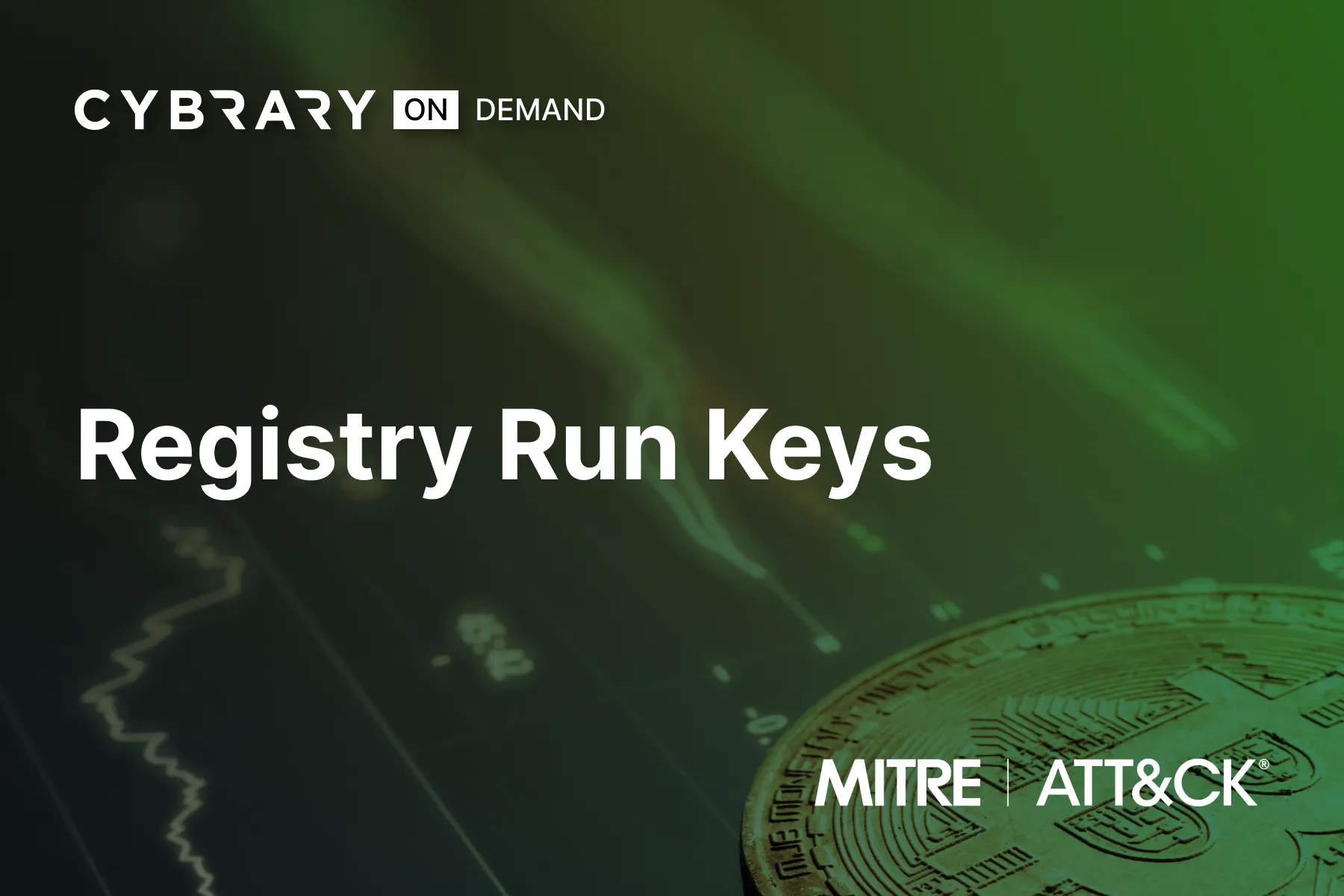
Registry Run Keys

Certified Cloud Security Professional (CCSP) Practice Test
This practice test from CyberVista will help you prepare for the Certified Cloud Security Professional (CCSP) exam by demonstrating your knowledge and hands-on experience with cloud security architecture, design, operations and service orchestration.

Using the OWASP Zed Attack Proxy Web Application Scanner
In this challenge you will gain understanding of OWASP Zed Attack Proxy by performing basic scans against a vulnerable web server. OWASP ZAP is an open-source web application security scanner, and is used by those new to application security as well as seasoned professional penetration testers.

Perform Automatic Remediation by Using an AWS Config Rule
Gain hands-on experience configuring an AWS Config rule to ensure that public access to Amazon S3 buckets is automatically disabled. Lab activities include: creating an Amazon Identity and Access Management role for automation, creating an S3 bucket that is noncompliant, and creating an AWS Config rule to remediate any noncompliant S3 buckets.

Enable Security for Azure Data Lake
Gain hands-on experience enabling security for Azure Data Lake. Lab activities include: deploying a storage account that uses Azure Data Lake Storage, creating a container for blob data, designing access control list (ACL) and role-based access control (RBAC) security for the data lake, and enabling ACL and RBAC security for the data lake.

Configure Security Recommendations for Virtual Machines
This "Configure Security Recommendations for Virtual Machines" IT Pro Challenges virtual lab teaches learners how to configure security recommendations for a virtual machine by enabling security recommendations and Azure Disk Encryption. Skills learned in this lab are crucial for the job roles of System Administrators and Azure Administrators.

Configure Azure Disk Encryption
This IT Pro Challenge virtual lab teaches how to encrypt a virtual machine’s disk using Azure’s Cloud Shell commands. Hands-on experience — creating and deploying a virtual machine and a data disk, and using a command-line interface — advances System Administrators, Cybersecurity Specialists, and Microsoft Azure Cloud Engineers career paths.

Create and Configure Application Security Groups
The Azure platform provides cloud-based tools for businesses to deploy their web services. This IT Pro Challenge virtual lab will give you an Azure application security groups' overview. Application security groups allow system administrators to control access to Azure virtual machines. In this lab, you will deploy an application security group.

Configure Virtual Network Connectivity using Peering
In this lab, you assume the role of an Azure® administrator. First, you will create an Azure virtual network by using the Azure portal. Next, you will create an Azure virtual network by using Azure Cloud Shell. Finally, you will configure virtual network peering connections for secure bidirectional communication.

CCSP: Domain 3 - Cloud Platform and Infrastructure Security
In this CCSP: Domain 3 - Cloud Platform and Infrastructure Security course you will learn about how to design a secure data center, about identification, authentication, and authorization in the cloud, as well as business continuity and disaster recovery planning and testing.

Certified Information Systems Auditor (CISA)
Showcase your audit experience and demonstrate that you are skilled at assessing vulnerabilities, reporting on compliance, and instituting controls within the enterprise by obtaining your ISACA CISA certification. Completion of the practice test means you understand the five domains within the formal ISACA exam.

CompTIA Security+ SY0-701 Practice Test
Prepare yourself to enter the cybersecurity field or validate your knowledge by earning the Security+ certification. This practice test for CompTIA Security+ (SY0-701) will help you identify domain areas to study more so you know when you're exam ready. Be sure to set yourself up for exam success. Start this practice test today.

Cybrary Challenge: Motor Mayhem

Challenge: Memory Mysteries
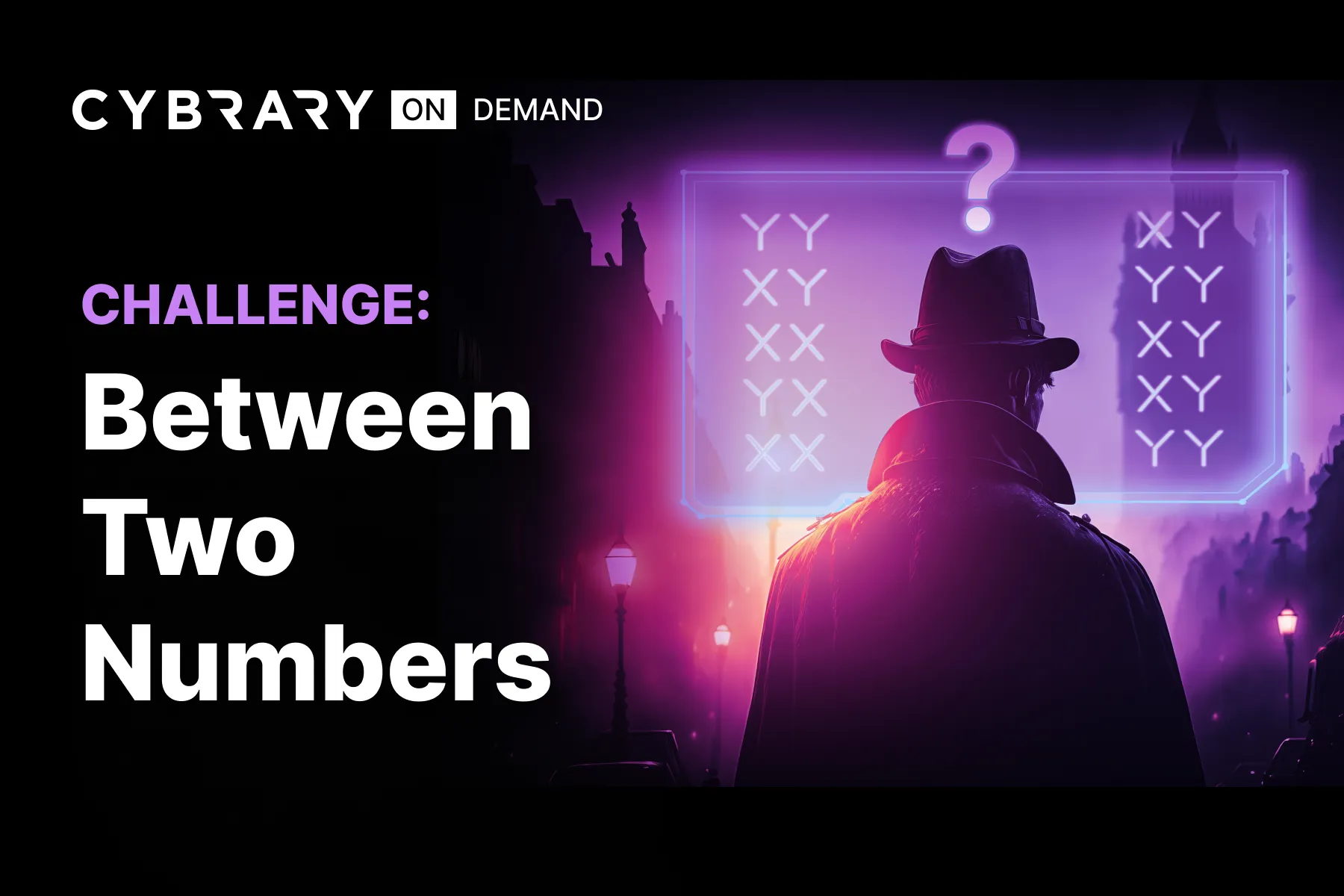
Challenge: Between Two Numbers

Challenge: Saving A Fellow Spy

Challenge: Space Mission
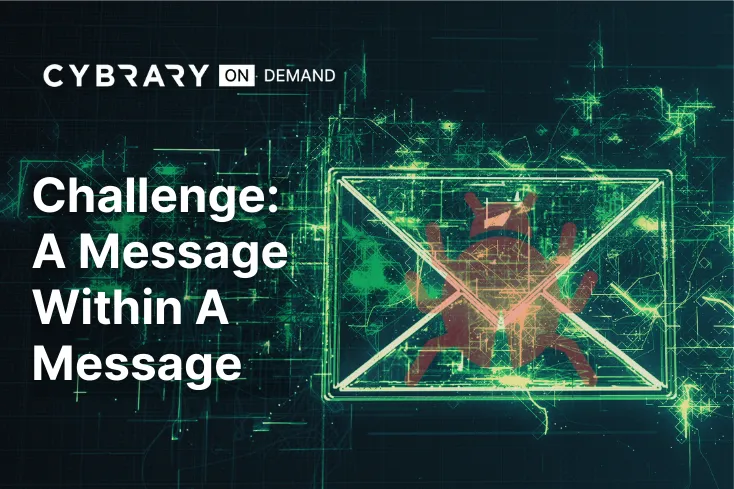
Challenge: A Message Within A Message
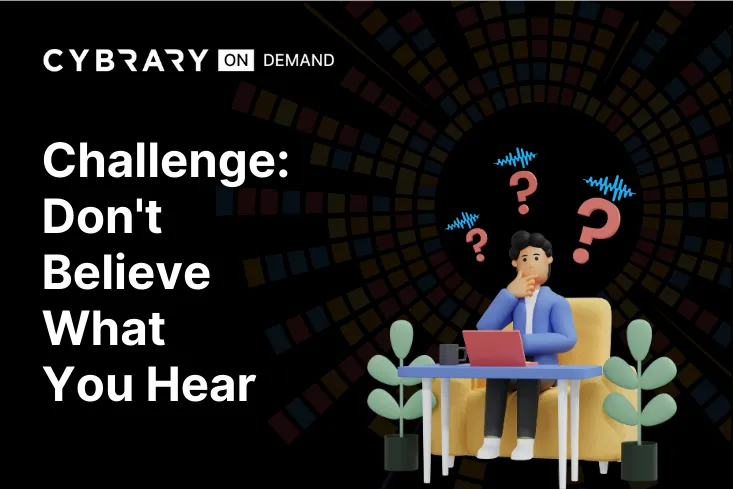
Challenge: Don't Believe What You Hear
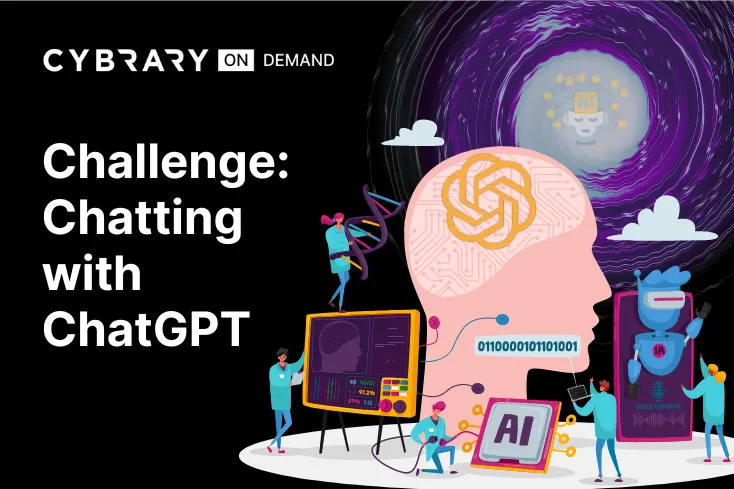
Challenge: Chatting with ChatGPT
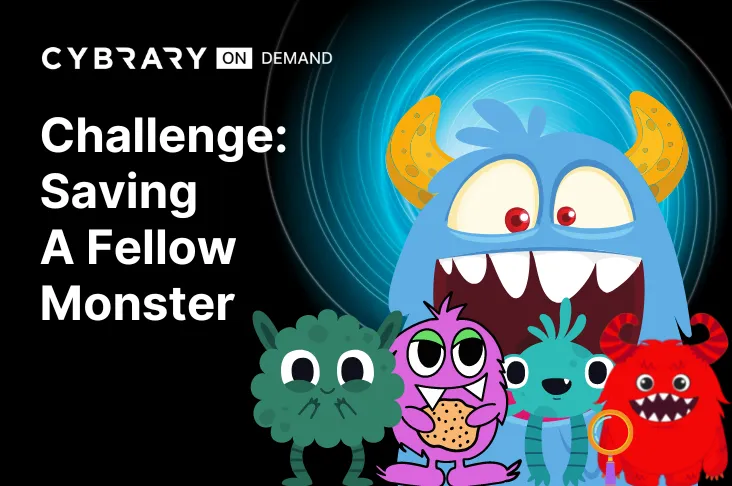
Challenge: Saving a Fellow Monster
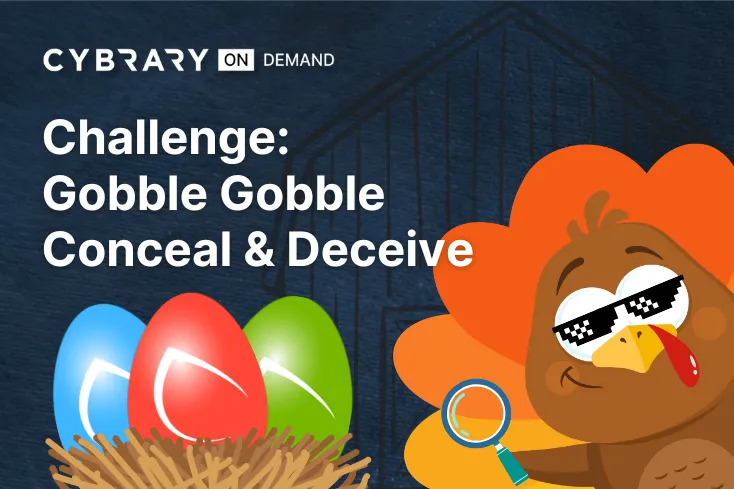
Challenge: Gobble Gobble Conceal & Deceive
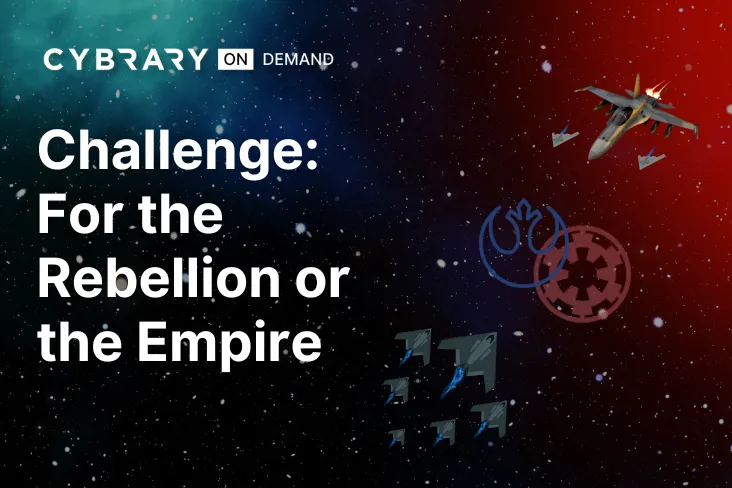
Challenge: For the Rebellion or the Empire

Challenge: Update B4 It's 2Late
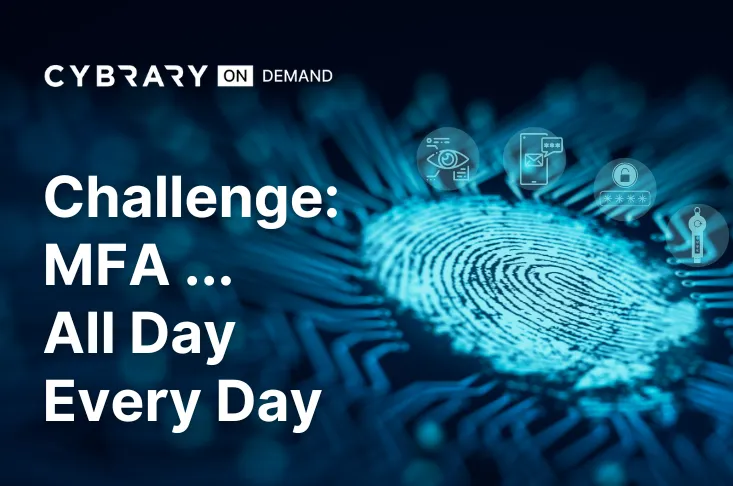
Challenge: MFA ... All Day Every Day
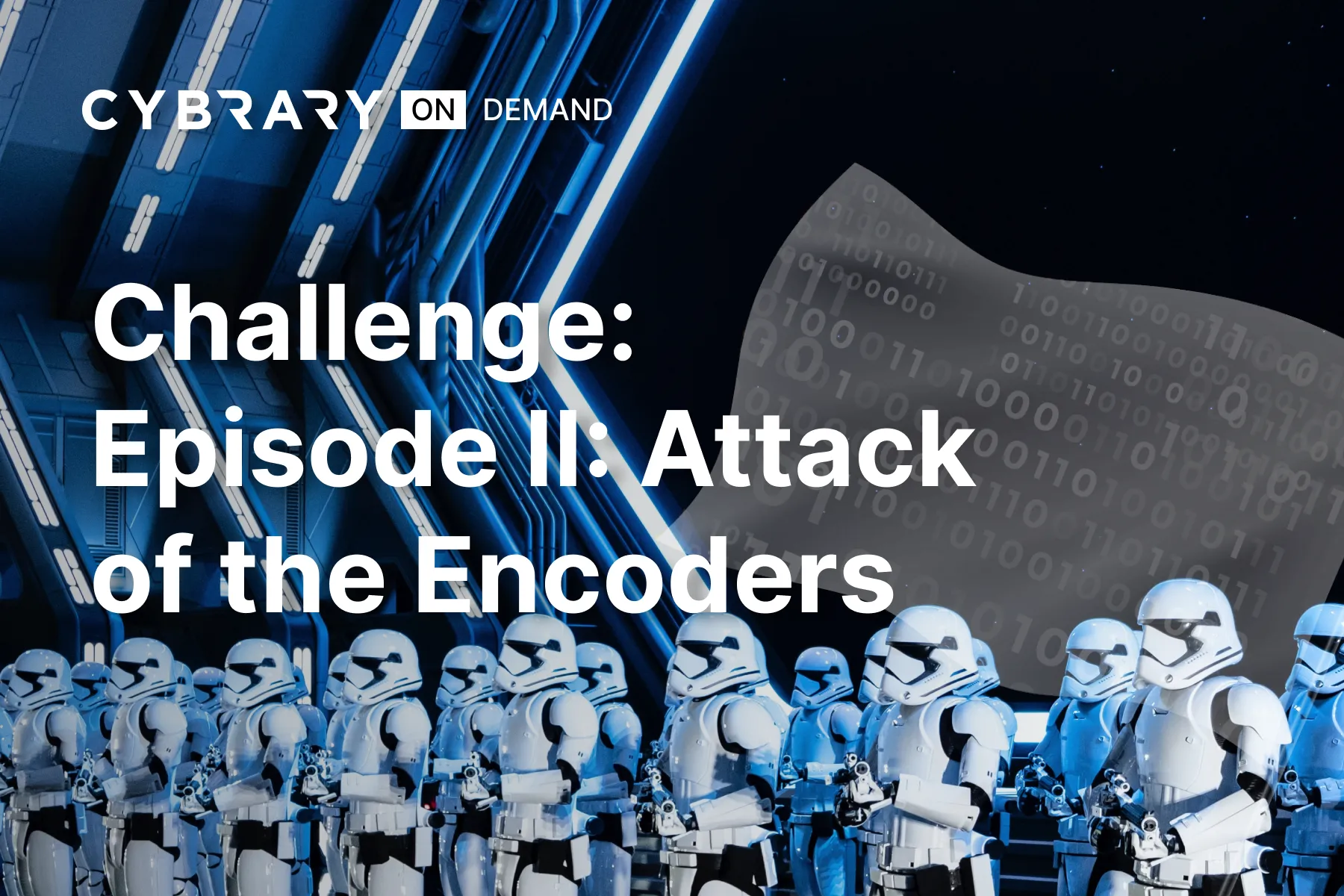
Challenge: Episode II - Attack of the Encoders

Challenge: The Base(64)ics
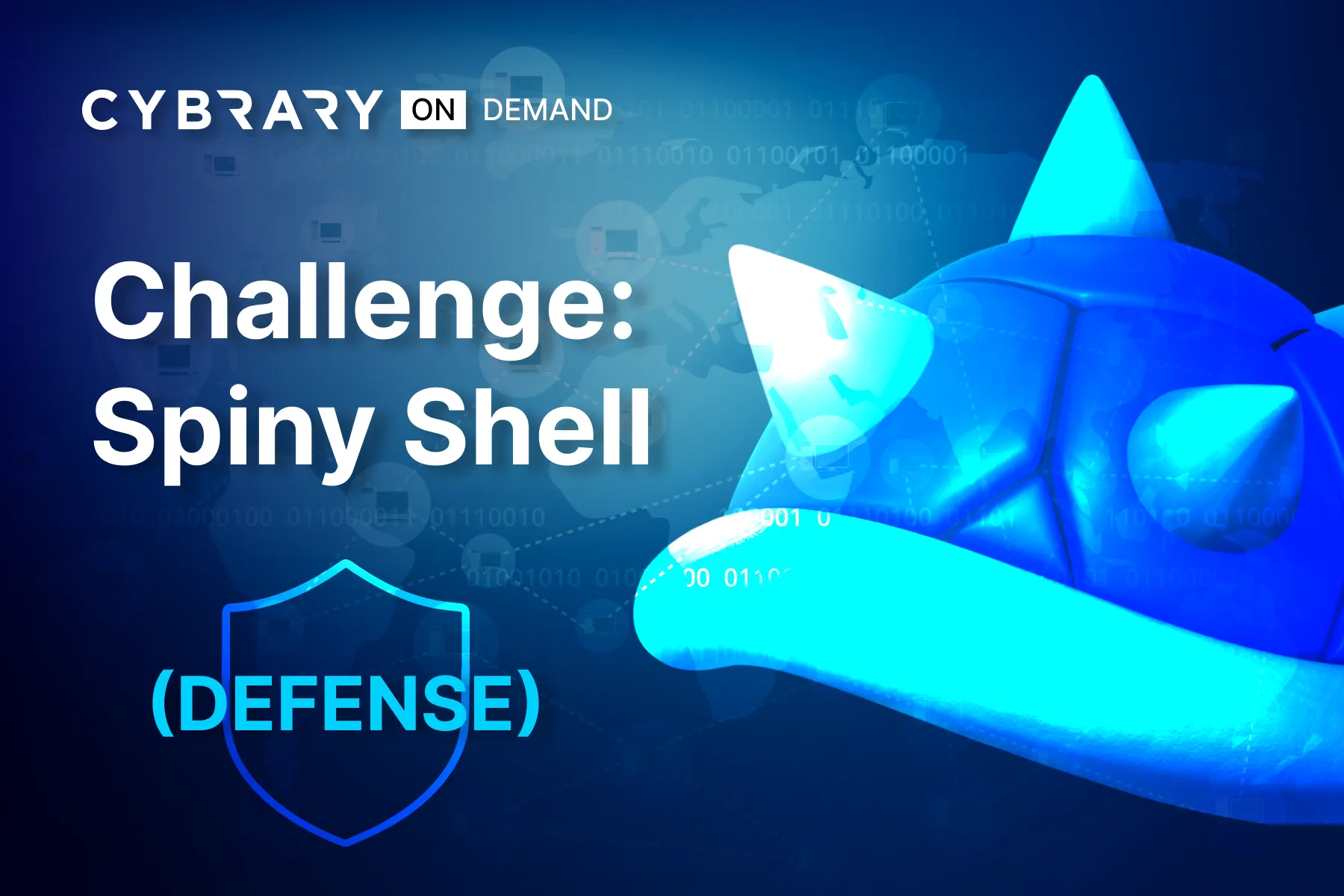
Challenge: Spiny Shell
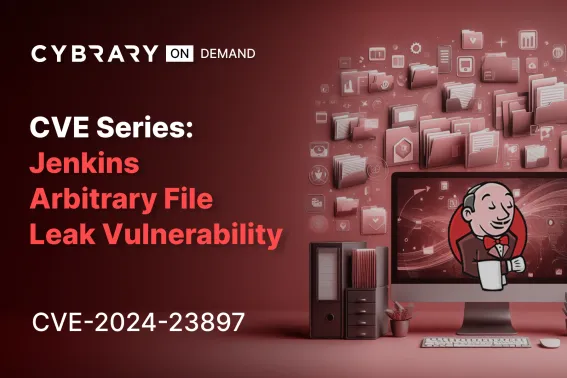
CVE Series: Jenkins Arbitrary File Leak Vulnerability (CVE-2024-23897)
CVE-2024-23897 is a critical security flaw affecting Jenkins, a Java-based open-source automation server widely used for application building, testing, and deployment. It allows unauthorized access to files through the Jenkins integrated command line interface (CLI), potentially leading to remote code execution (RCE).
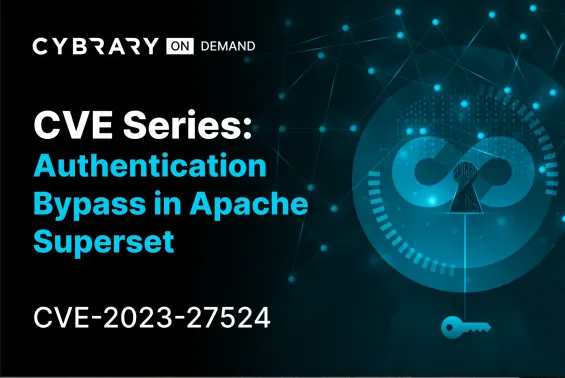
CVE Series: Authentication Bypass in Apache Superset (CVE-2023-27524)
CVE-2023-27524 is a critical vulnerability in Apache Superset, affecting versions up to 2.0.1. It enables attackers to bypass authentication by exploiting weak or default SECRET_KEY values. Attackers can forge session cookies to gain admin access, leading to potential remote code execution and unauthorized data access.
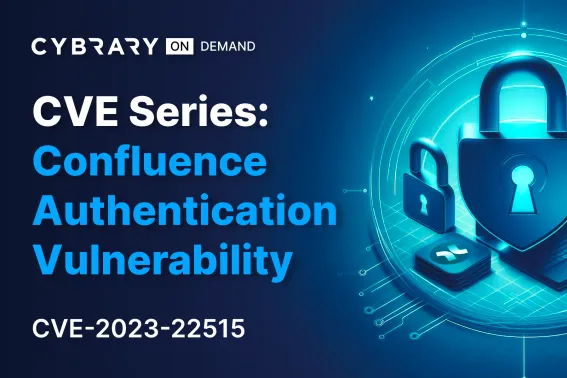
CVE Series: Confluence Authentication Vulnerability (CVE-2023-22515)
Confluence suffers from a Broken Access Control vulnerability that affects Data Center and Server versions 8.0.0 to 8.3.2, 8.4.0 to 8.4.2, and 8.5.0 to 8.5.1. Threat actors exploit this vulnerability to obtain administrator access to Confluence servers. Put on your Red Team hat to create your own malicious admin account leveraging this CVE!

CVE Series: WinRar Vulnerability (CVE-2023-38831)

CVE Series: Openfire (CVE-2023-32315)
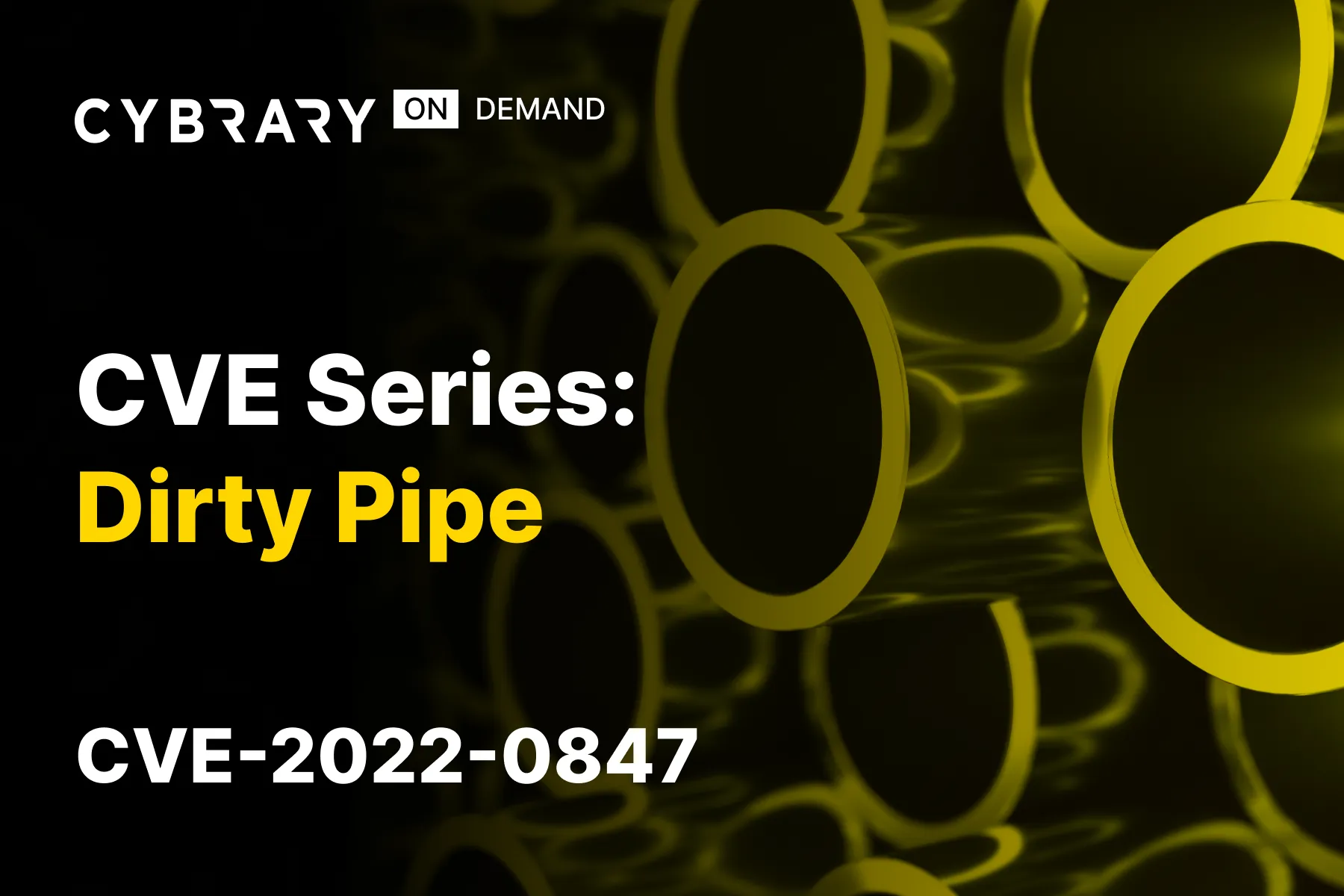
CVE Series: Dirty Pipe (CVE-2022-0847)
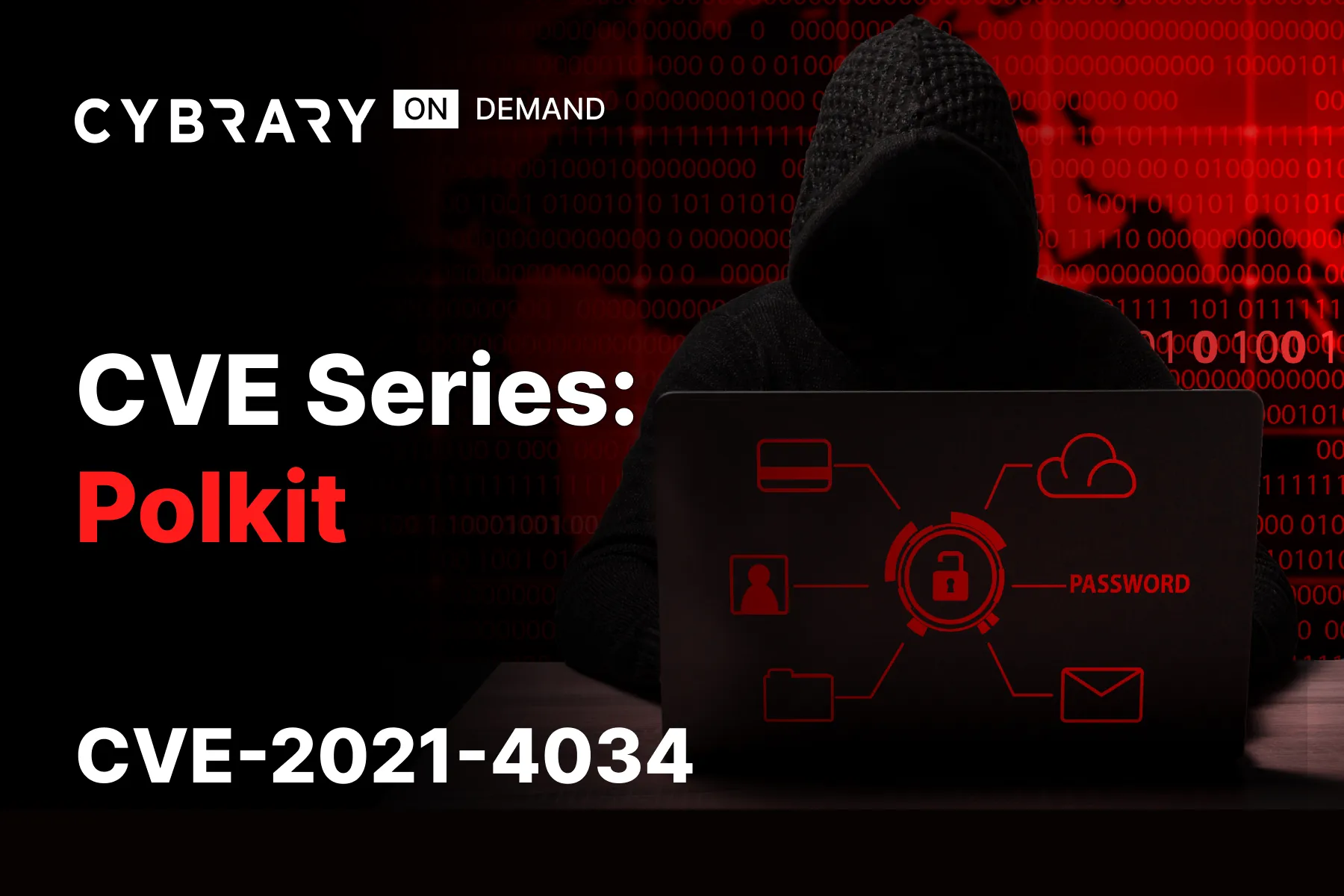
CVE Series: Polkit (CVE-2021-4034)
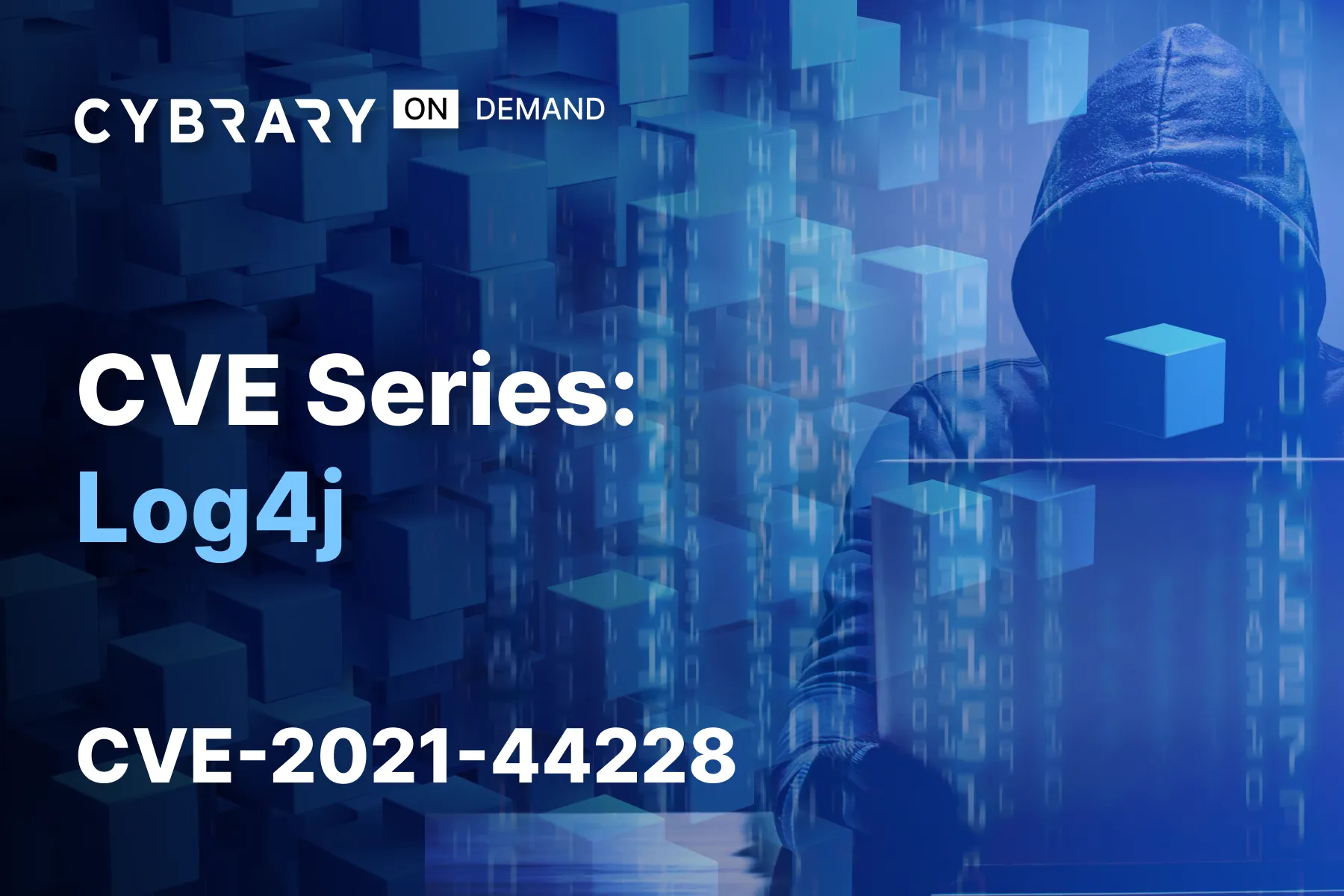
CVE Series: Log4J (CVE-2021-44228)
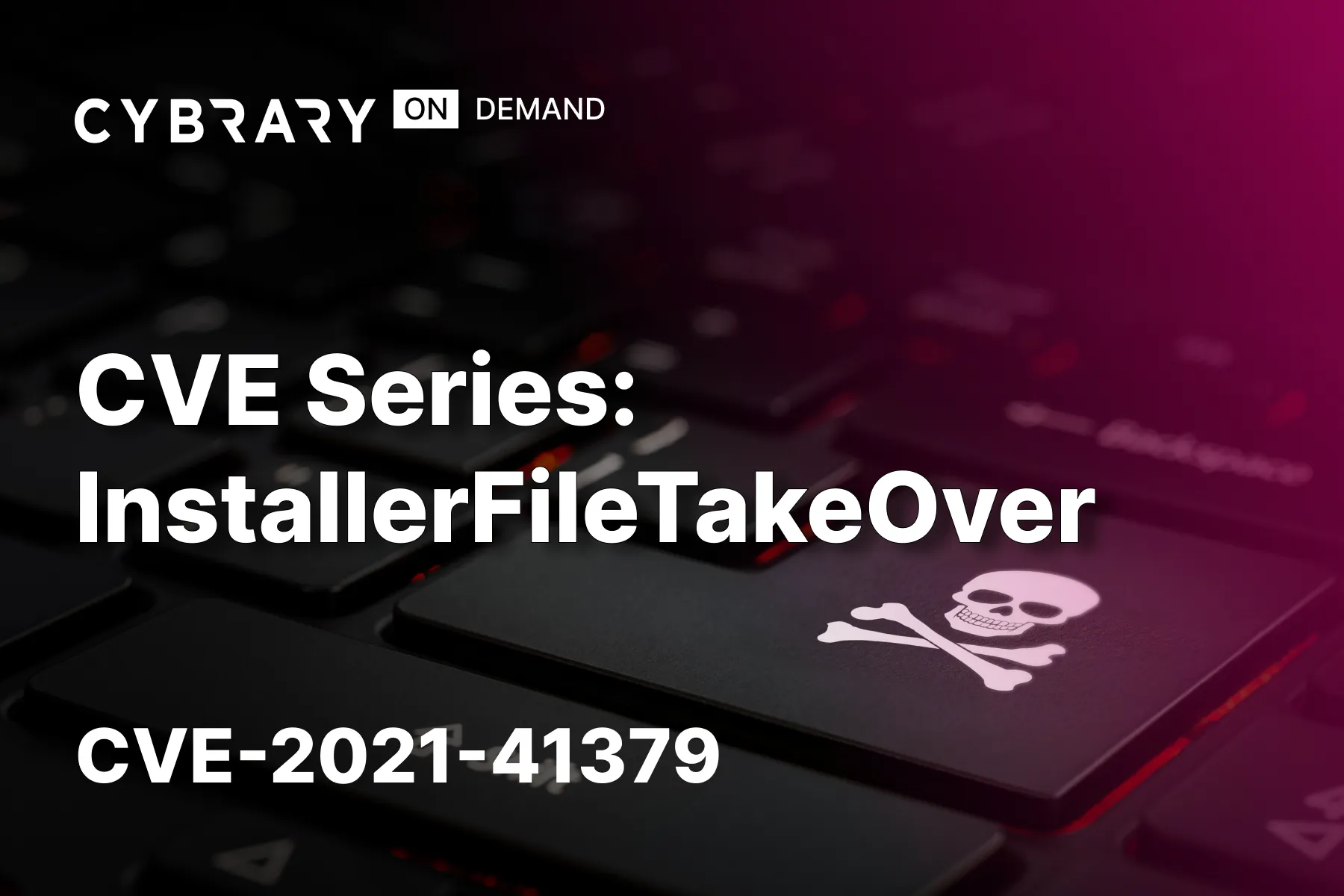
CVE Series: InstallerFileTakeOver (CVE-2021-41379)
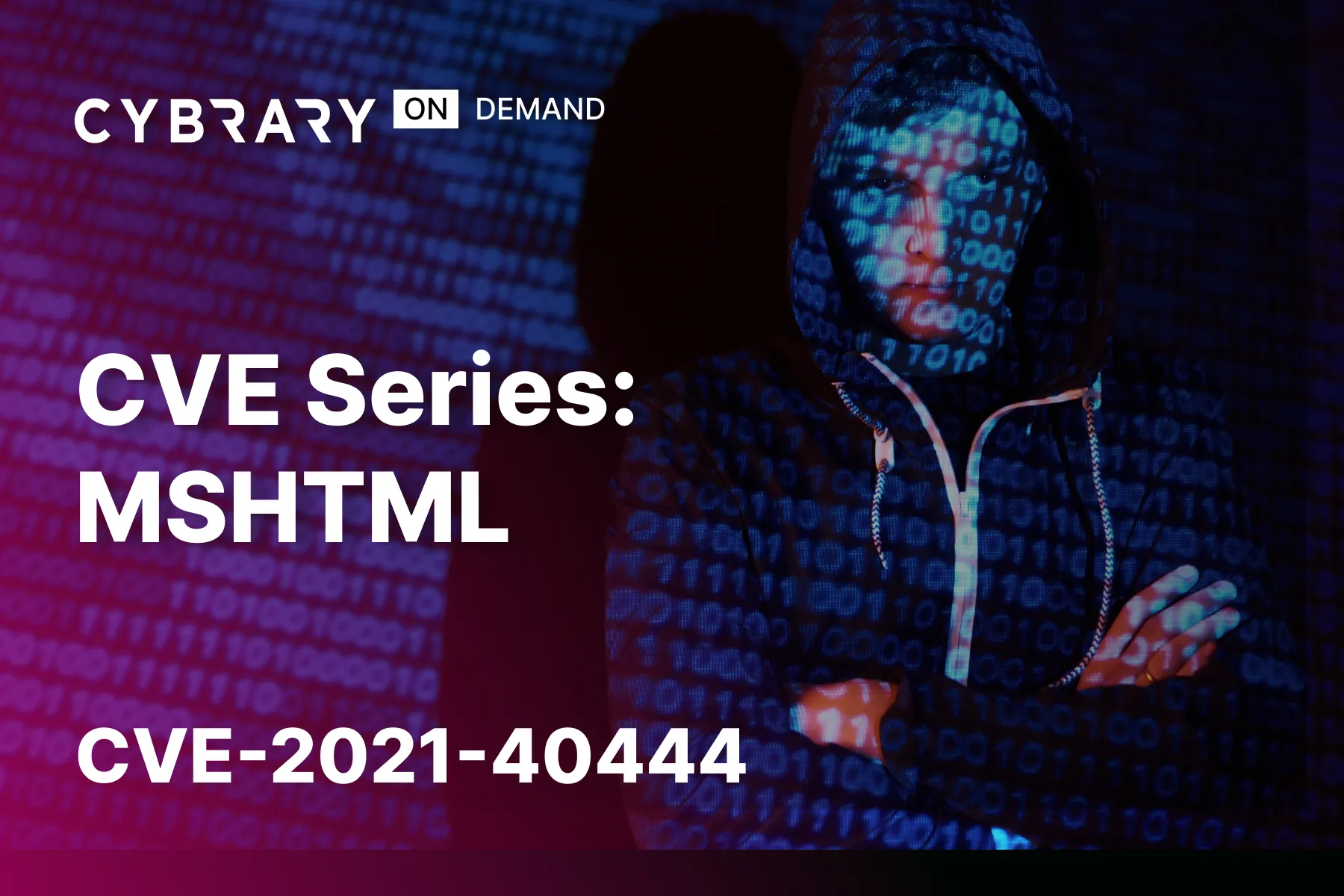
CVE Series: MSHTML Vulnerability (CVE-2021-40444)

CVE Series: HiveNightmare (CVE-2021-36934)

CVE Series: PrintNightmare (CVE-2021-1675 and CVE-2021-34527)

CVE Series: Ghostcat (CVE-2020-1938)
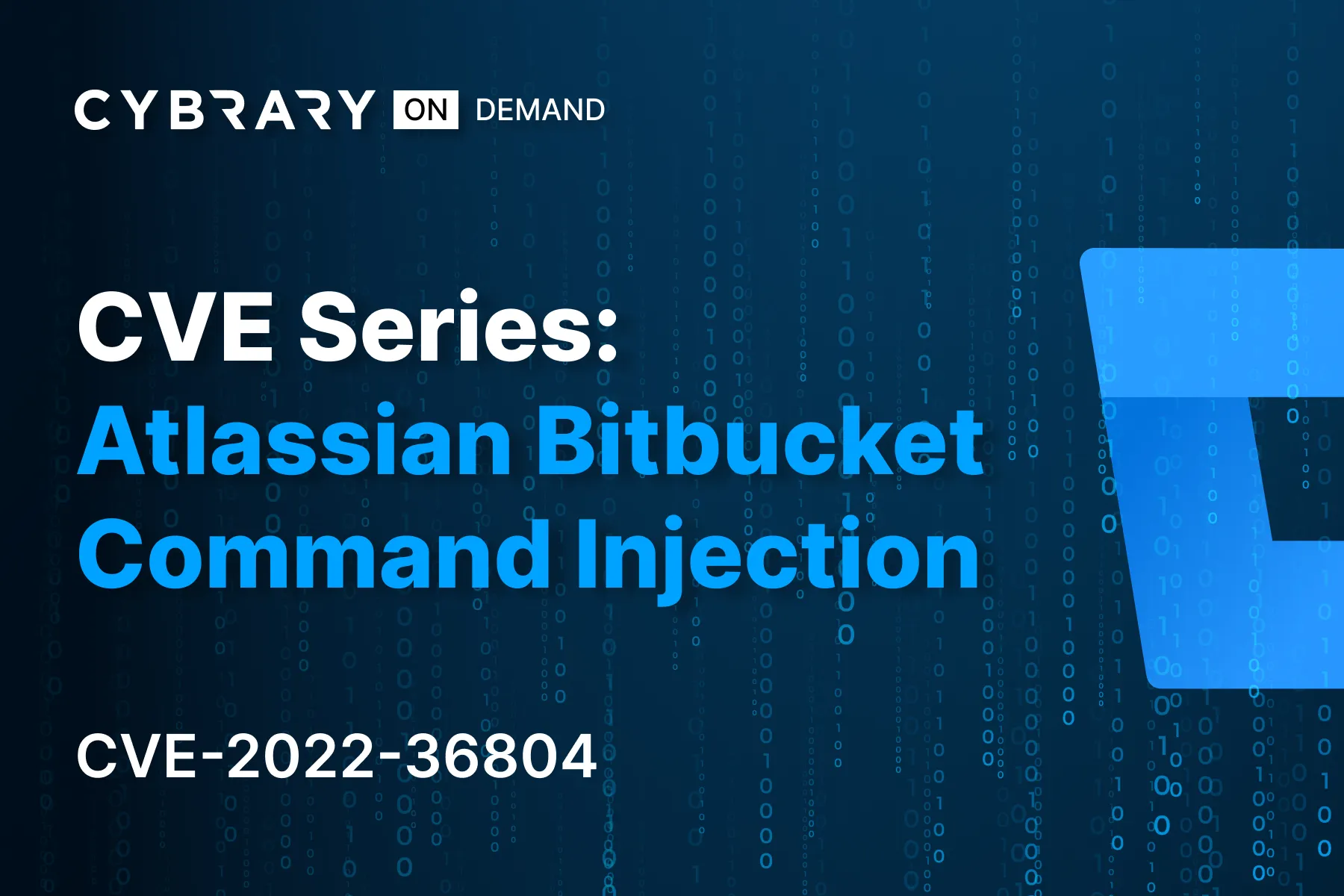
CVE Series: Atlassian Bitbucket Command Injection (CVE-2022-36804)
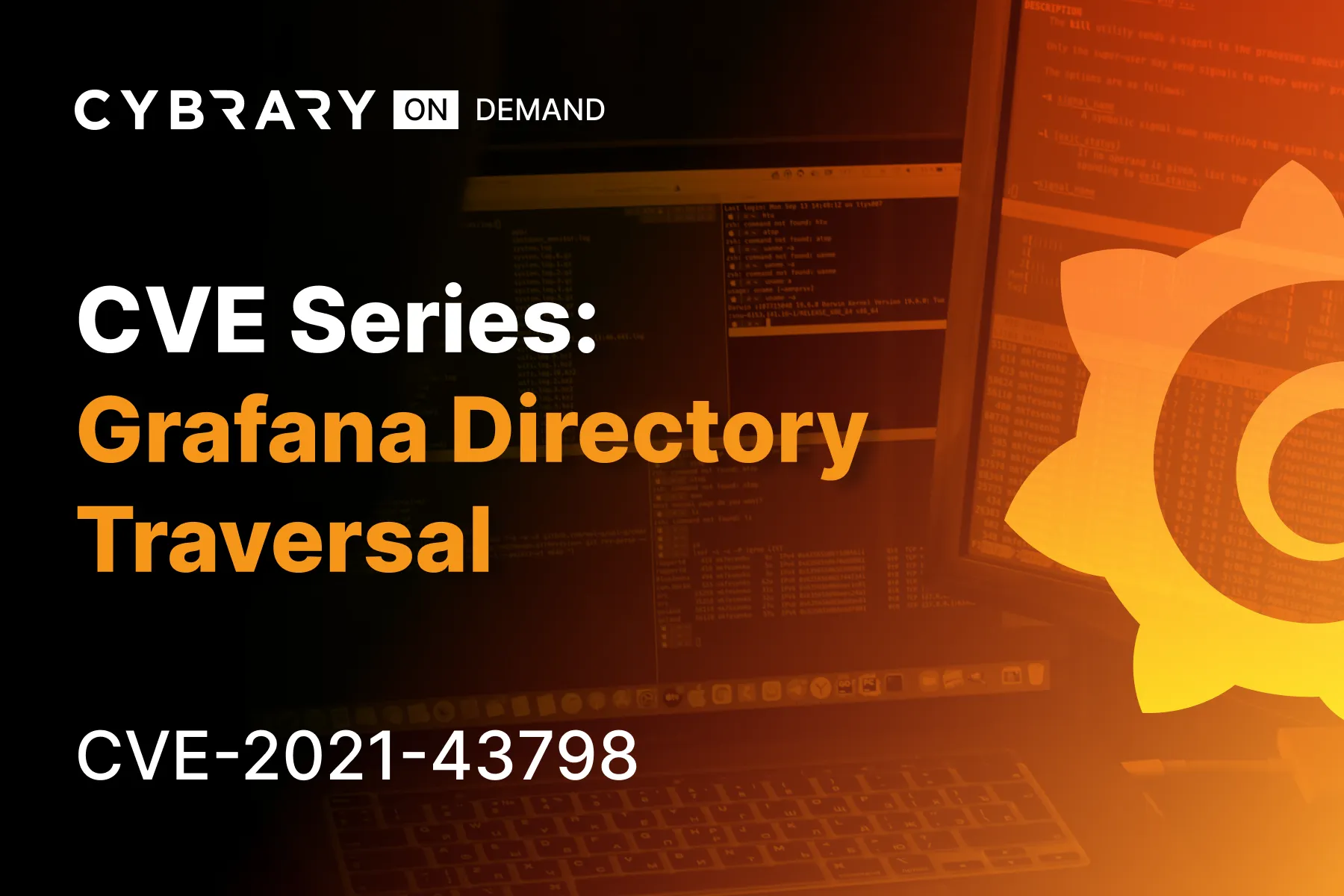
CVE Series: Grafana Directory Traversal (CVE-2021-43798)
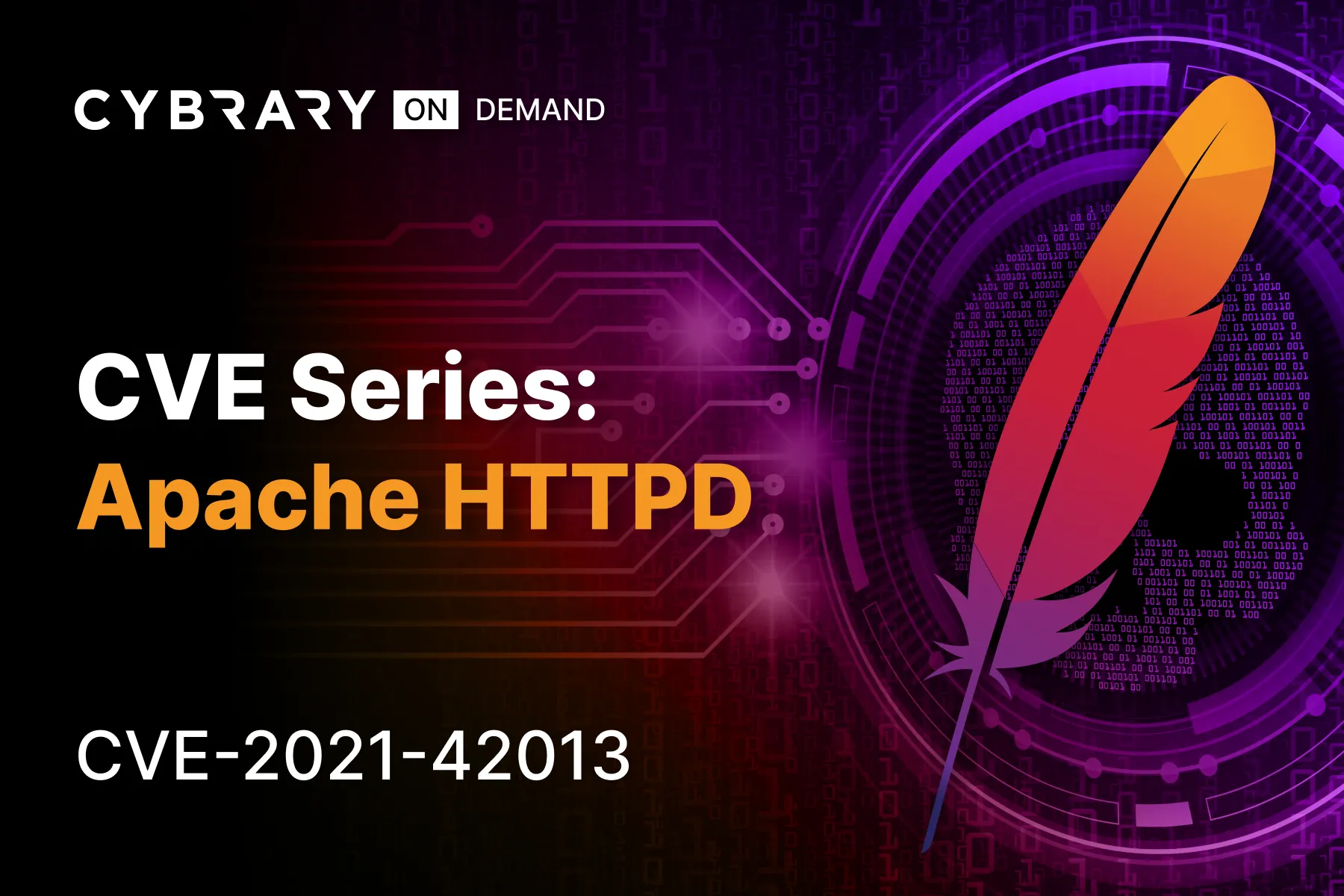
CVE Series: Apache HTTPD (CVE-2021-42013)
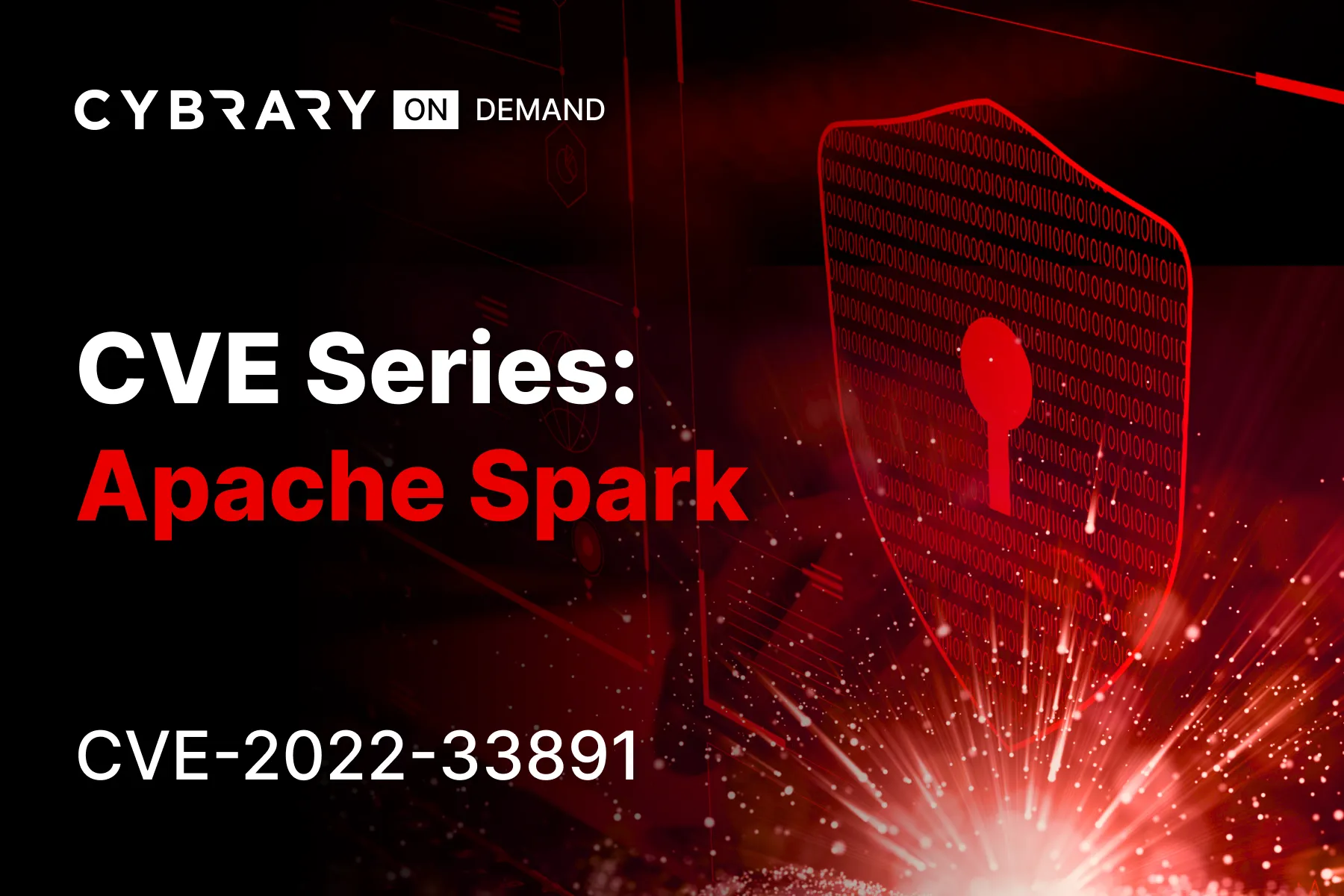
CVE Series: Apache Spark (CVE-2022-33891)
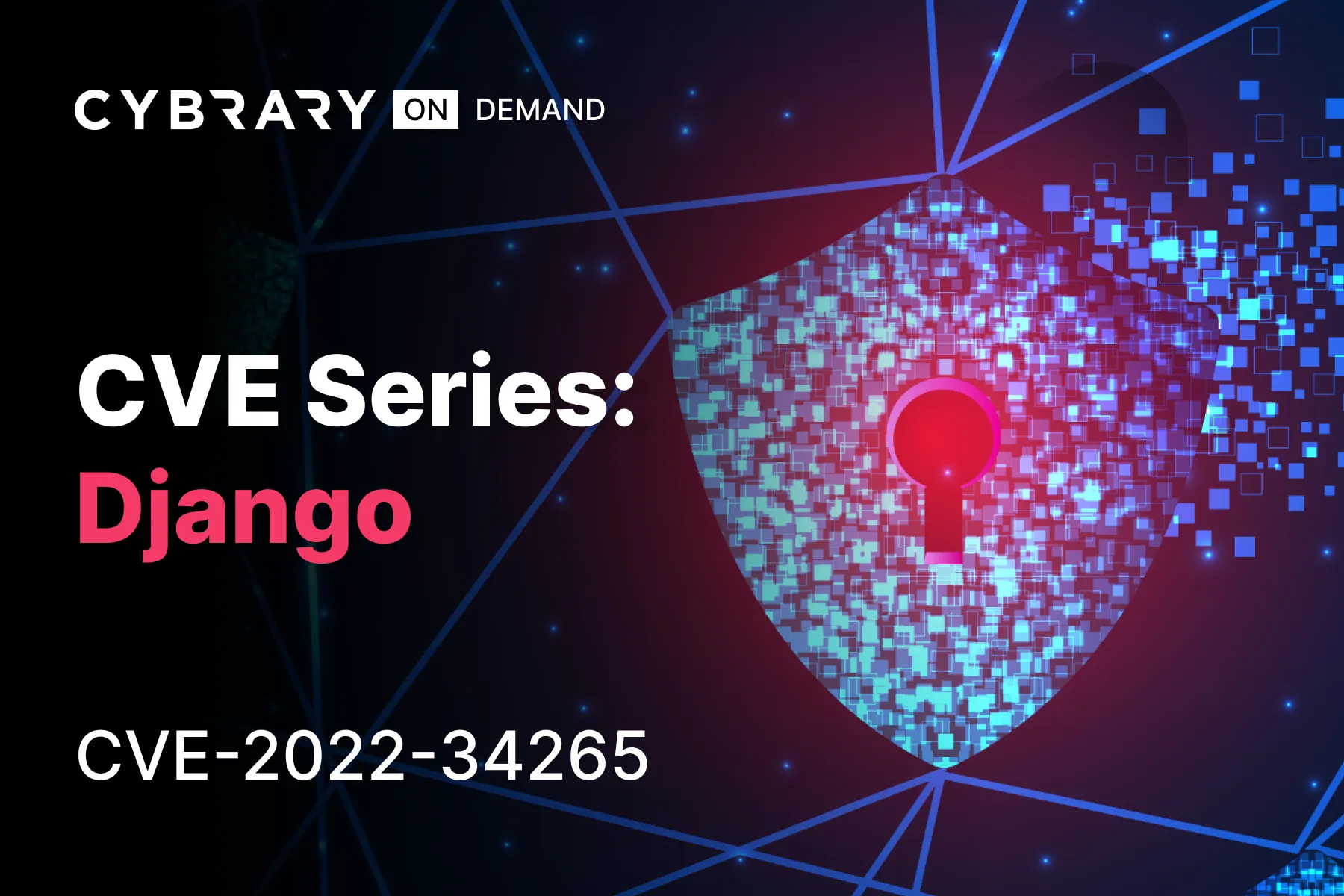
CVE Series: Django (CVE-2022-34265)
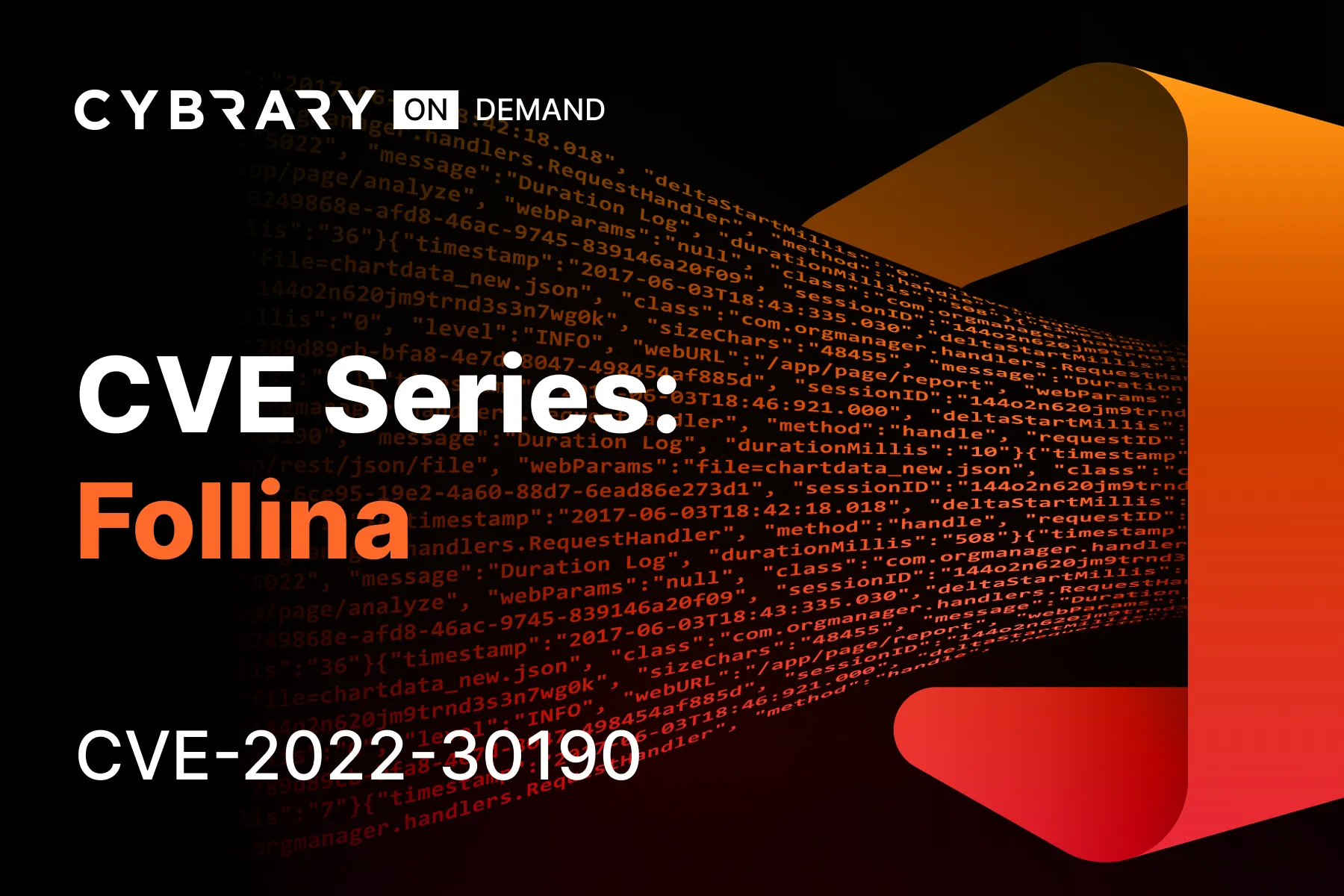
CVE Series: Follina (CVE-2022-30190)

CVE Series: Confluence RCE (CVE-2022-26134)
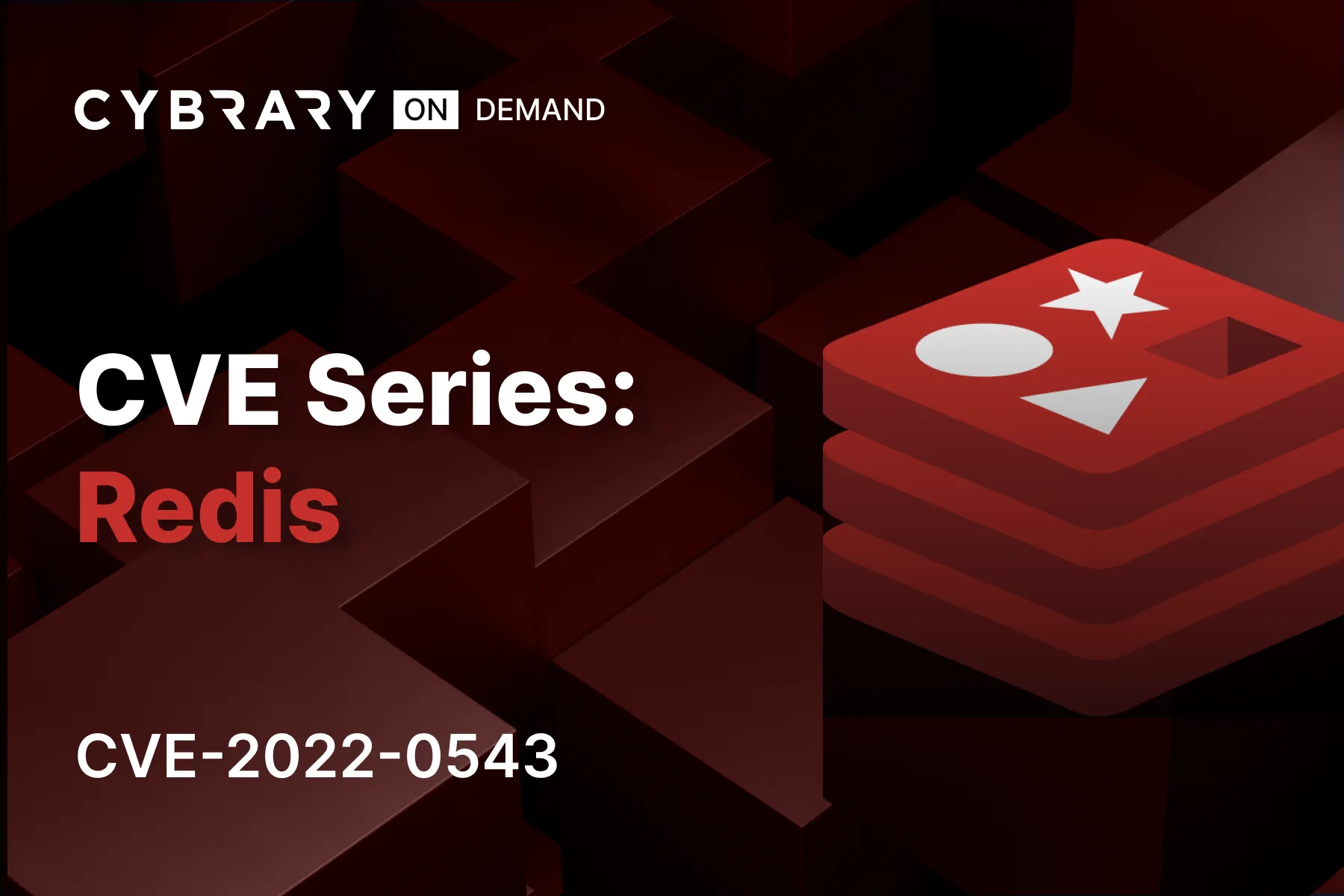
CVE Series: Redis (CVE-2022-0543)

CVE Series: Spring4Shell (CVE-2022-22965)

Royal Ransomware Group
Royal is a spin-off group of Conti, which first emerged in January of 2022. The group consists of veterans of the ransomware industry and brings more advanced capabilities and TTPs against their victims. Begin this campaign to learn how to detect and protect against this newer APT group!
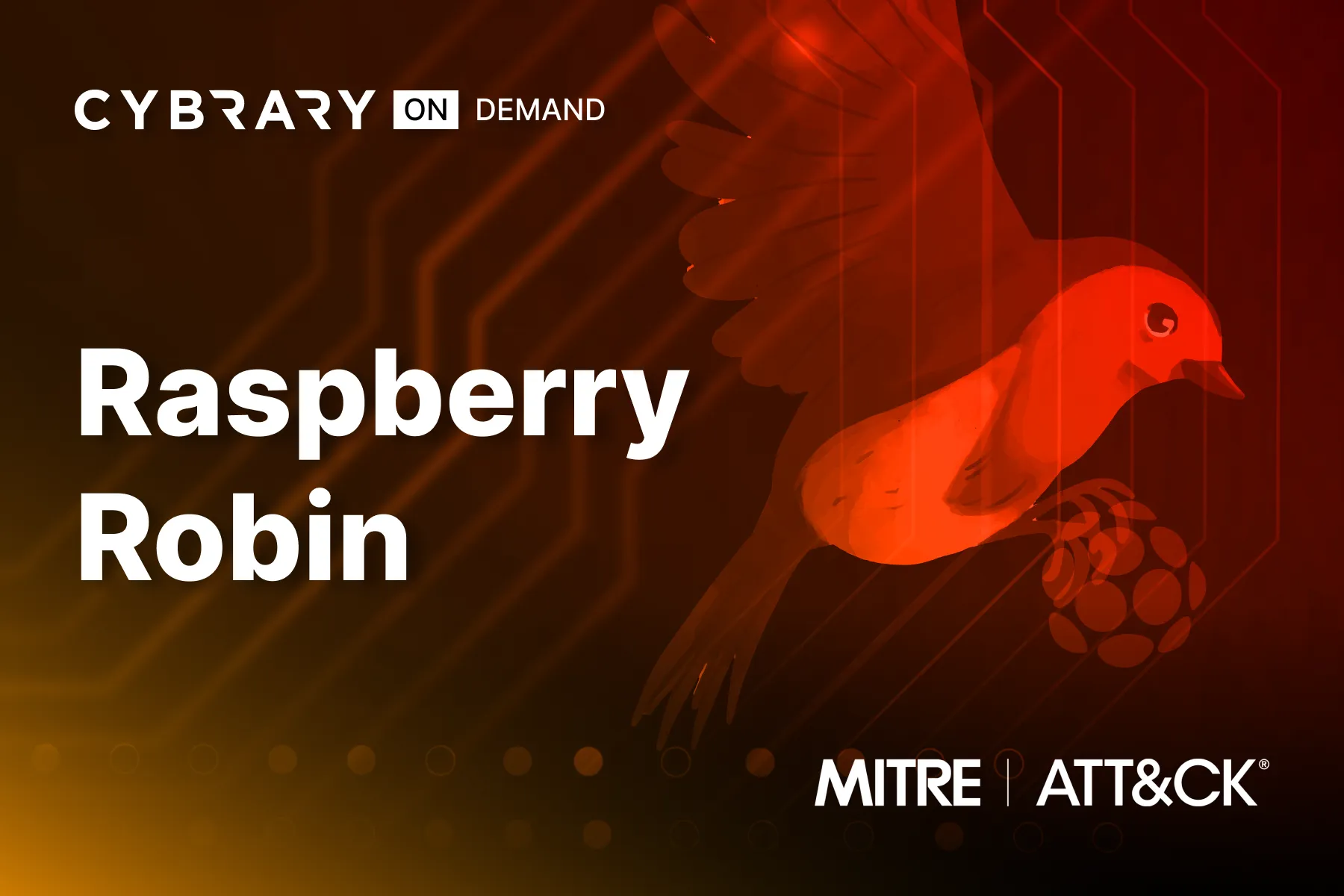
Raspberry Robin
Raspberry Robin is a malware family that continues to be manipulated by several different threat groups for their purposes. These threat actors (Clop, LockBit, and Evil Corp) specialize in establishing persistence on a compromised host and creating remote connections to use later. Once established, these C2 connections can be used for multiple purposes, including data exfiltration, espionage, and even further exploitation.

Double Trouble with Double Dragon

Weak Link in the Supply Chain
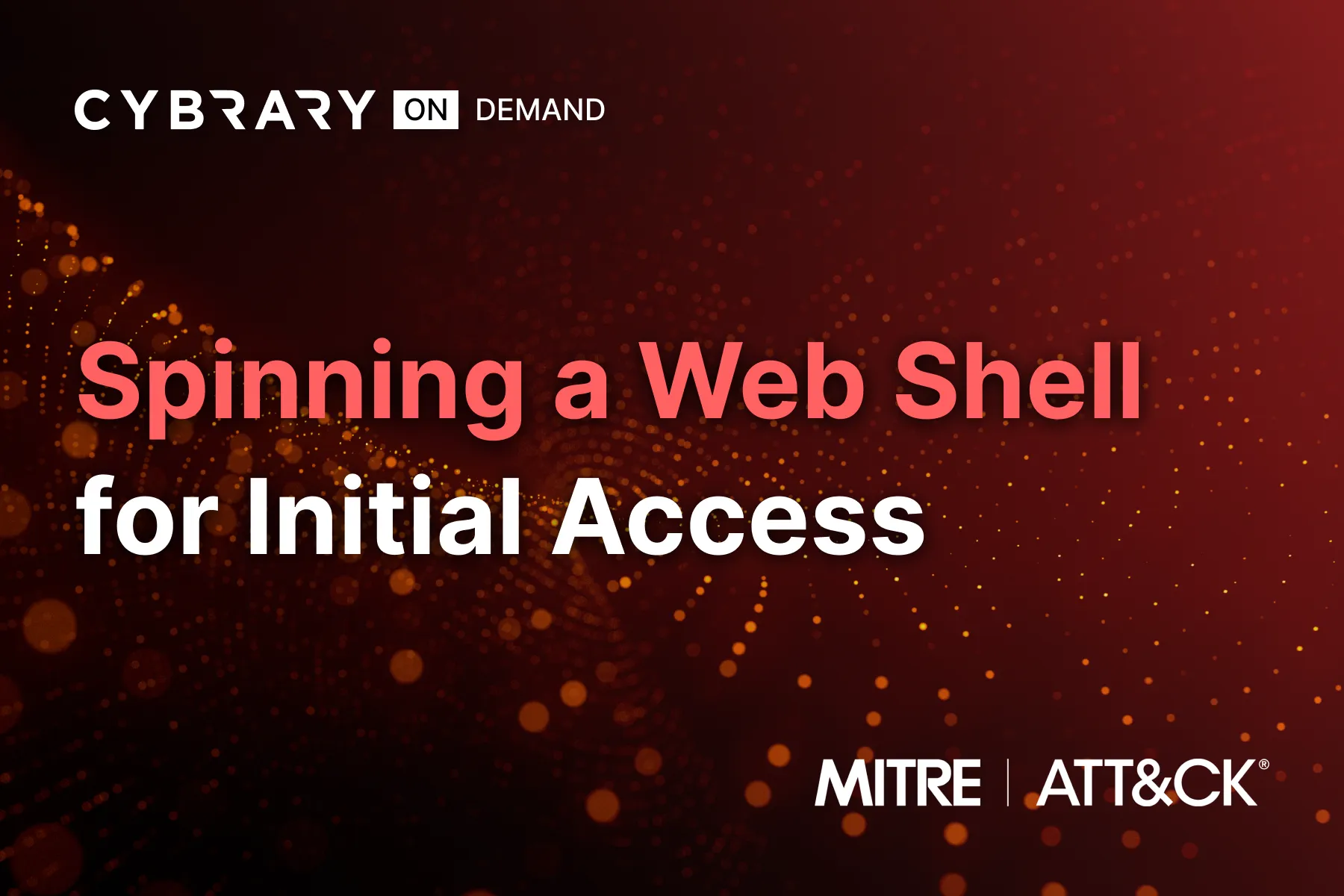
Spinning a Web Shell for Initial Access

Exfiltration and Extortion
Threat actors will use stolen data exfiltrated from victim systems to extort organizations. Once they gain a foothold, they delete critical system files and threaten to release the data or disrupt operations if the victims do not pay up. Understanding these techniques is vital to defending your organization from such attacks.
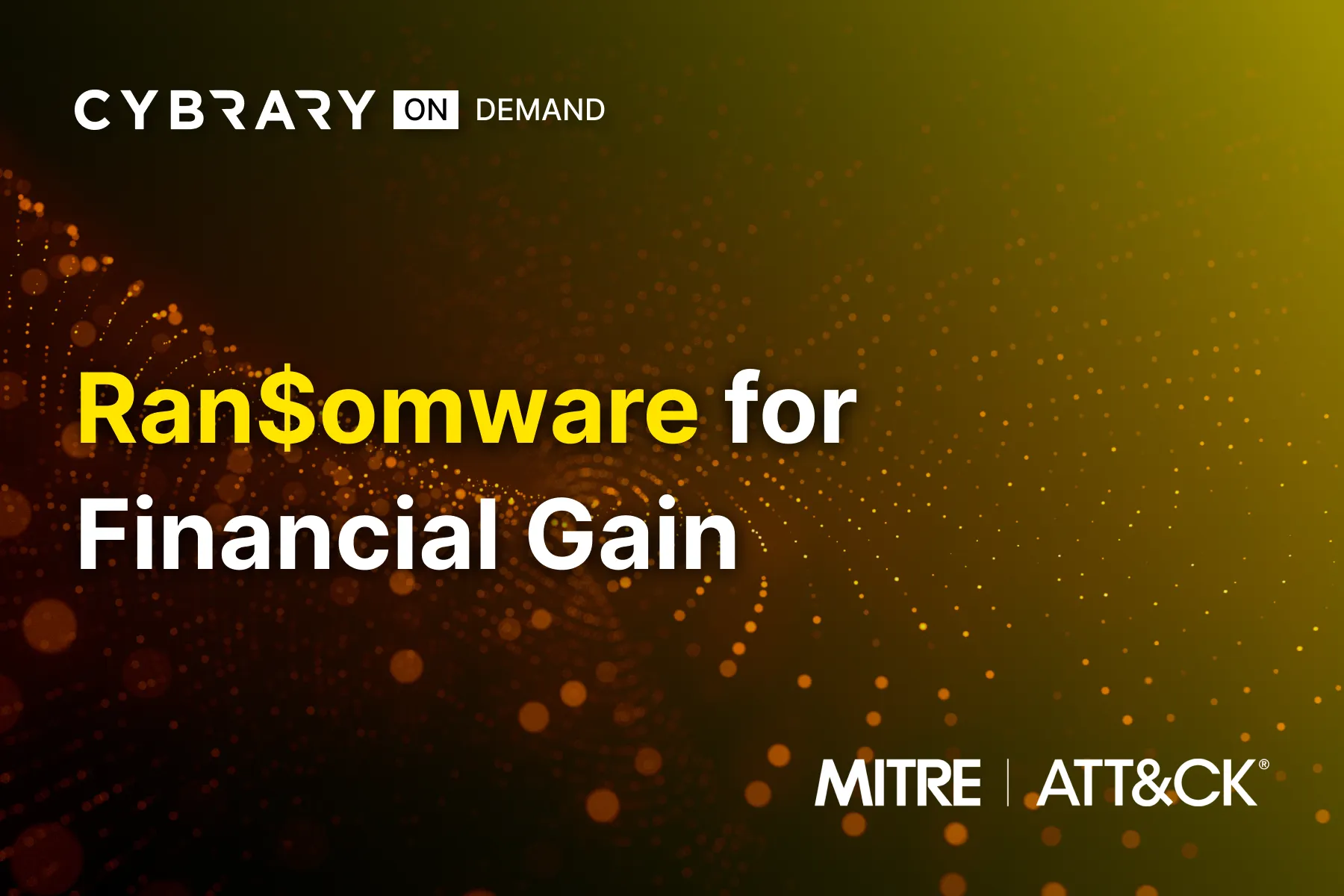
Ransomware for Financial Gain
Threat actors continue to leverage ransomware to extort victim organizations. What was once a simple scheme to encrypt target data has expanded to include data disclosure and targeting a victim’s clients or suppliers. Understanding the techniques threat actors use in these attacks is vital to having an effective detection and mitigation strategy.
Our Instructors
Industry seasoned. Cybrary trained.
Our instructors are current cybersecurity professionals trained by Cybrary to deliver engaging, consistent, quality content.