Overview
This IT Pro Challenges virtual lab teaches learners how to access an Azure storage account used by the web app with private access. Learners will learn using Azure Storage, Blob container, SAS token to integrate Blob storage. Skills learned in this lab are valuable in multiple roles, such as SQL Developer, Database administrator, etc.
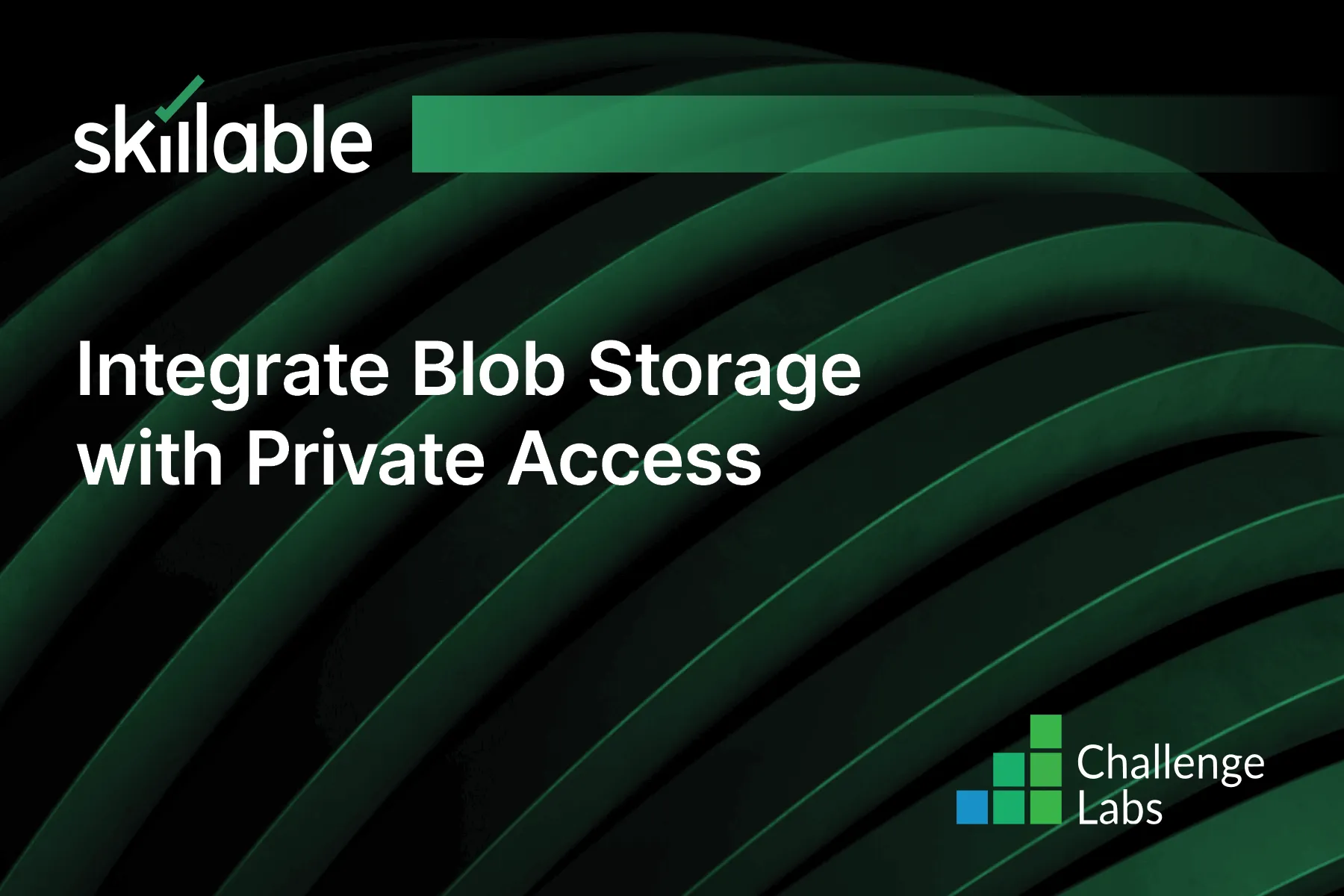
Learning Partner
Skillable
Ready-built content across a variety of topics and technologies