Overview
This IT Pro Challenge virtual lab shows learners how to use Microsoft Visual Studio to modify an ADO.NET file with code that accesses a database for a MySQL server, creates a MySQL table, and populates and then retrieves data from the table. Learners will test their work using a web application.
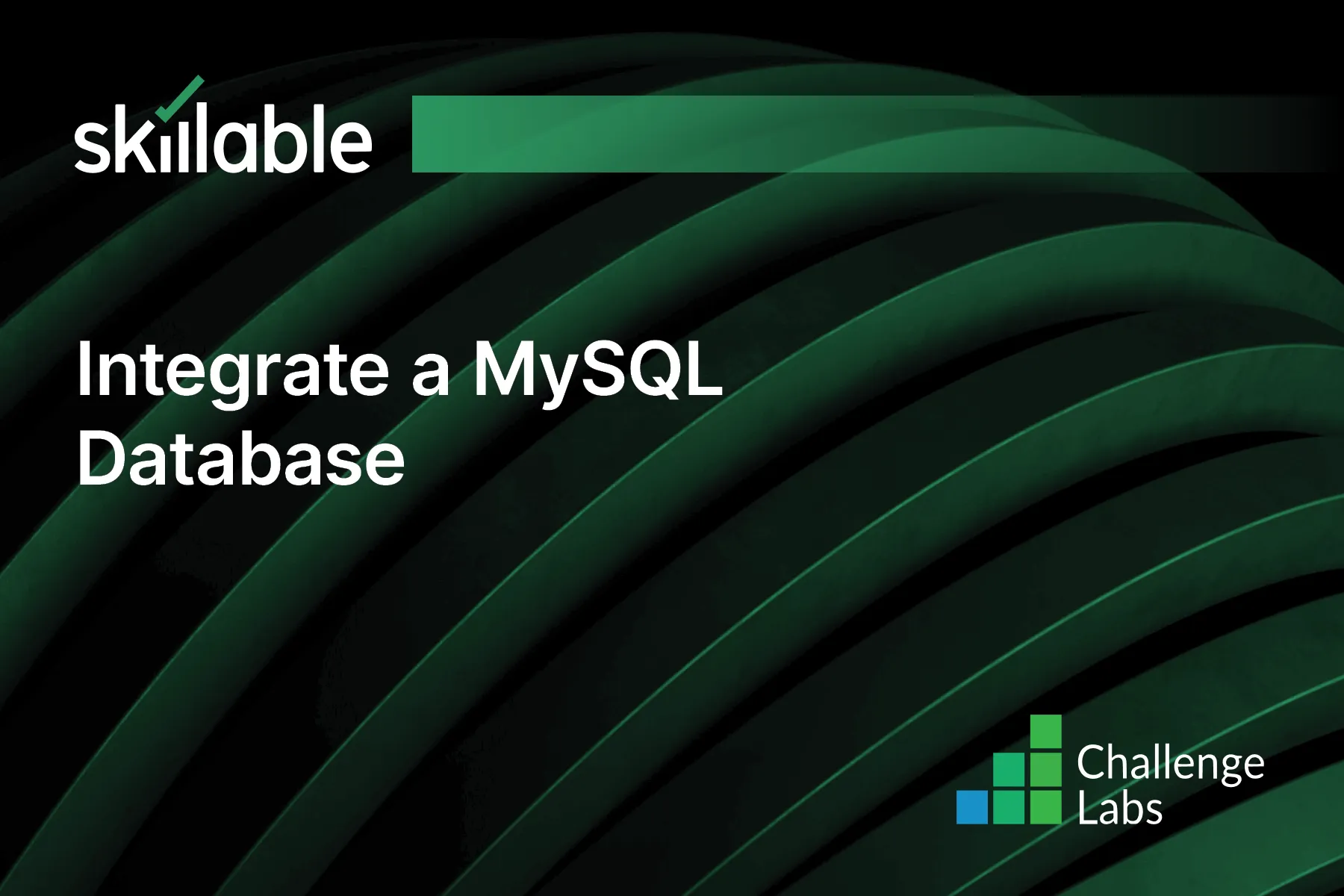
Learning Partner
Skillable
Ready-built content across a variety of topics and technologies