TL;DR
Have you ever thought of writing a program of your own or customizing a tool that you make use of regularly? To some, writing a program/tool is one of the scariest things they can imagine, especially with tons of code and the potential for errors. However, most people working in the IT industry may love to write a tool of their own. There are many scenarios where a simple program can save a lot of time. Think of an IT staff-member who would check connectivity for a list of hosts regularly throughout a day or filter log files of a system for errors and generate a report. These things would be much harder, time-consuming, and troublesome as a manual process, but if the responsible one spends a little time writing a tool that can handle all of these things, they would be automated, time-saving, and precise. This article discusses a command language interpreter, Bash, which can be used to create scripts, along with two other handy tools.
What is Bash?
Bash is the shell, or command language interpreter, for GNU/Linux operating systems. Bash is an acronym for __B__ourne-__A__gain __SH__ell. Executing Bash commands requires a terminal where the Bash commands are entered. Here are some sample Bash commands.
lstext in bold - prints the contents(files and folder) of a directory mkdirtext in bold New_Folder- creates the New_Folder pingtext in bold IP_Address - checks the connection of a host with the IP_Address rmtext in bold File - removes File cptext in bold File Destination_Directory - copies the File to Destination_Directory mvtext in bold File Destination_Directory - moves the File to Destination_Directory Touchtext in bold File_Name - create an empty file named File_Name
What is a Bash script?
A file containing Bash commands is called a Bash script. Bash scripts can be extremely simple or extremely complex. They are usually saved with the extension ".sh." Below is an example where a file is created using the nano text editor. The text in the file simply states "ping google.com," and it is saved as ping__text in bold___google.sh.

Now, by running the script, it executes the command, and the system establishes a connection with (pings) Google.
Learn More With The "Linux Fundamentals for Security Practitioners" Course >>
Why Bash scripts?!
Although it is all command-line, which is not user-friendly, it is much more powerful than GUI (Graphical User Interface) based options. There are many reasons to use Bash scripts, such as:
- It is easy to learn and easy to write programs with.
- It can execute any command easily just as they would execute in a terminal, such as ping, nano, ls, tcpdump, traceroute, convert,text in bold and much more. Other programming languages, however, require specific modules.
- It is a scripting language, and it has all the features, such as variables, flow control (conditional statements like if), iterative structures (for and while loops), and much more.
- It can be used to make very sophisticated tools.
- It can be used to run a series of commands as a single command.
- Once written, It can save a lot of time because it allows for the automation of repetitive, mundane, or complex tasks.
- It is portable (i.e., can be executed in any Unix-like operating systems without any modifications) or can simply be stored with other scripts on a USB drive and run on any Unix-based operating systems.
Despite the above advantages, sometimes, Bash scripts are very complicated to write. It is also limited to GNU/Linux operating systems, which means they can only be used on Linux-based systems. Compared to any programming language, slow execution speed is another downside for bash scripts, as a new process is launched for almost every shell command executed. These are the major limitations of Bash scripts.
What to learn for writing a Bash script?
Learning any scripting language would take a bit of time, and Bash is not an exception. For learning Bash and writing scripts using it, the following points should prove helpful:
- Learn (some) Bash commands and tools used in Linux-based machines. For book lovers, Kali Linux Revealed would be a great place to start. Cybrary also has a course called Kali Linux Fundamentals, which is also a great place to start.
- Learn the syntax for Bash, such as defining variables, taking input from the user, if-statements, loops, and so on. This helps create sophisticated tools. The following websites are helpful as well:
https://ryanstutorials.net/bash-scripting-tutorial/ https://linuxconfig.org/bash-scripting-tutorial-for-beginners
- Create while learning. Practicing while learning is extremely helpful. It can inspire ideas to use the capability better. Try to create sample scripts while learning this powerful scripting language.
Creating sample tools using Bash!
For creating tools using any programming/scripting language, one should have an idea or imagination of what he/she wants to do as there is an Einstein's quotation that says, "Imagination is more important than knowledge."
File classifier
Scenario: There is a folder called test which has 812 files in it. They are all videos, documents, pictures, and audio files. According to their extension, the mission is to create four folders in this directory named Documents, Pictures, Music, and Videos, and move the files to specific directories. At the end of the process, all documents will be in the Documents folder, images will be in the Pictures folder, audio files will be in the Music folder, and videos will be in the Videos folder.
The above scenario is simply an example of how Bash scripts can be used for sorting files. However, sorting files may be needed in various situations. Say there is a program that generates five different files each time it runs, and the user has to transfer all of them to their specific directories. This and other frequent sorting tasks are easily done using Bash, and once it is automated, there is no need to do them manually.
Solution:
- Open the working folder. In this case, it is called test.
- Right-click on it, and click on "Open in Terminal." A terminal will open in the current directory.

- Hereafter, everything is done through the terminal. Create a script file using "nanotext in bold classify_files.sh". Name the file anything, but here, it's named classify_files.sh.

- First of all, create the four folders mentioned earlier. For creating folders, use "mkdirtext in bold Documents Videos Pictures Music."
- Now, this is the time to move all images to the Pictures folder using the following commands.
mvtext in bold *jpeg Pictures mvtext in bold *gif Pictures mvtext in bold *jpg Pictures mvtext in bold *webp Pictures
- The mv command syntax is "mvtext in bold file destination_folder," which will move the file to destination_folder. The asterisk (*) is a wildcard and matches any character(s), so the commands above will move any file ending with jpeg, jpg, gif, and webp to the Pictures directory. This folder contains only these picture file types, but the commands can change for other picture file formats.
- Next, move all documents to the Documents directory using the following commands.
mvtext in bold *doc Documents mvtext in bold *docx Documents mvtext in bold *odt Documents mvtext in bold *pdf Documents mvtext in bold *rtf Documents mvtext in bold *txt Documents
- The above commands move all doc, docx, odt, rtf, pdf, and txt file formats to the Documents folder.
- After that, move all audio files to the Music directory using the following commands.
mvtext in bold *mp3 Music mvtext in bold *aiff Music mvtext in bold *aac Music mvtext in bold *wav Music
- This time mvtext in bold is used to move all files ending with mp3, aiff, aac, and wav to the Music folder.
- Then move all video files to the Videos directory using the following commands.
mvtext in bold *mp4 Videos mvtext in bold *avi Videos mvtext in bold *mpeg Videos mvtext in bold *wmv Videos
- Next, print a verification message using the command below.
echotext in bold "All files moved to their respective directories."
The command echotext in bold will print whatever is provided after that on the terminal's screen, known as stdout (standard output). So, it will print the message inside the double quotation mark. The final version of the script file looks like the following picture.

Finally, press "ctrl+xtext in bold" to close the editor, press "ytext in bold," and hit the Entertext in bold key right after that to save all the things written so far.
Let's check the process right now. The picture below is a screenshot of the directory where 812 files are located to be categorized. The respective screenshot only shows 198 files out of 812. Imagine how difficult and time-consuming it would be to find all video files among 812 files and move them to a separate directory.

Let's return to the terminal and run the script using the command "bashtext in bold calssify_files.sh."
After pressing the Entertext in bold key, it won't take more than a 1000th of a second, and the last line in our script, which was the verification message, will be printed out on the terminal.

Let's go back to the test directory and recheck it. The effect of the script can be seen in the directory.
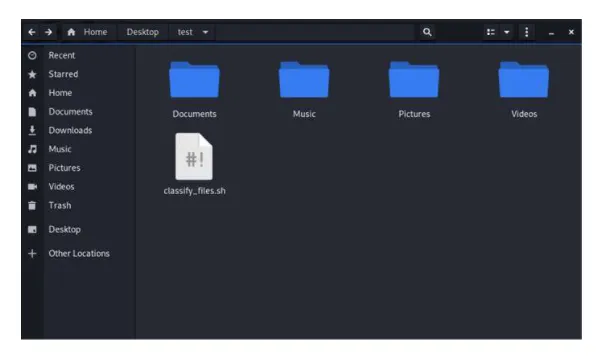
- And MISSION ACCOMPLISHED! As it is clear, four folders have been created, and the whole files have been transferred to their corresponding directories. The only file that remained outside is the script called classify_files.sh. Creating another directory called Scripts and moving all script files into that directory is a great idea. But this is an exercise for the reader! Give it a try!
A secondary interface to the tcpdump
For creating tools like this, the only thing that is needed is an idea. After that, the knowledge for applying it would be easily gathered using the internet. Recently, I was using a network-data packet analyzer called tcpdumptext in bold, which runs on the command-line interface. I was struggling with learning the flags for this command. After executing a command or two, I thought of creating an interface for this command, making using it easier. It would also be good for those who are learning it new or just want to use it without knowing more details about it.
A recently completed version of this script is available on GitHub, which can be accessed through the following link. https://github.com/karimbakhshamiry/tcpdump-interface/
Here is how this script works. As it runs it, it gives the user some choices to select from, as shown in the picture below.
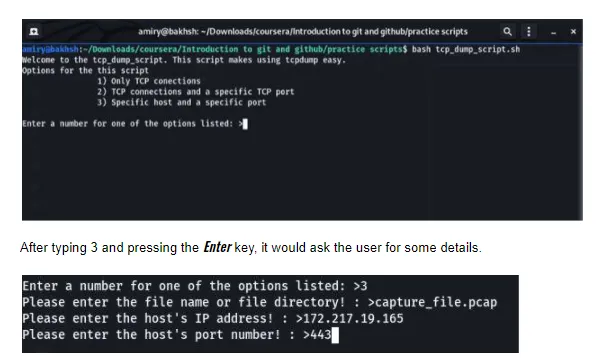
Once all the required details are given to the script, it creates the tcpdump command accordingly and executes it. Here is the output which filters all connections to 172.217.19.165 on port number 443 from the capture_file.pcap.

All in all, In the world of IT, there are many situations where scripting and automation can lighten a huge load of manual tasks. SysAdmins are mostly struggling with these types of situations, so spending a little time writing a program that can automatically do all those things can save a lot of time, and the tasks will no longer need to be done manually scripts will do them. Bash scripting can open the doors to a whole new world, so start learning this fascinating stuff today!
The Open Worldwide Application Security Project (OWASP) is a community-led organization and has been around for over 20 years and is largely known for its Top 10 web application security risks (check out our course on it). As the use of generative AI and large language models (LLMs) has exploded recently, so too has the risk to privacy and security by these technologies. OWASP, leading the charge for security, has come out with its Top 10 for LLMs and Generative AI Apps this year. In this blog post we’ll explore the Top 10 risks and explore examples of each as well as how to prevent these risks.
LLM01: Prompt Injection
Those familiar with the OWASP Top 10 for web applications have seen the injection category before at the top of the list for many years. This is no exception with LLMs and ranks as number one. Prompt Injection can be a critical vulnerability in LLMs where an attacker manipulates the model through crafted inputs, leading it to execute unintended actions. This can result in unauthorized access, data exfiltration, or social engineering. There are two types: Direct Prompt Injection, which involves "jailbreaking" the system by altering or revealing underlying system prompts, giving an attacker access to backend systems or sensitive data, and Indirect Prompt Injection, where external inputs (like files or web content) are used to manipulate the LLM's behavior.
As an example, an attacker might upload a resume containing an indirect prompt injection, instructing an LLM-based hiring tool to favorably evaluate the resume. When an internal user runs the document through the LLM for summarization, the embedded prompt makes the LLM respond positively about the candidate’s suitability, regardless of the actual content.
How to prevent prompt injection:
- Limit LLM Access: Apply the principle of least privilege by restricting the LLM's access to sensitive backend systems and enforcing API token controls for extended functionalities like plugins.
- Human Approval for Critical Actions: For high-risk operations, require human validation before executing, ensuring that the LLM's suggestions are not followed blindly.
- Separate External and User Content: Use frameworks like ChatML for OpenAI API calls to clearly differentiate between user prompts and untrusted external content, reducing the chance of unintentional action from mixed inputs.
- Monitor and Flag Untrusted Outputs: Regularly review LLM outputs and mark suspicious content, helping users to recognize potentially unreliable information.
LLM02: Insecure Output Handling
Insecure Output Handling occurs when the outputs generated by a LLM are not properly validated or sanitized before being used by other components in a system. Since LLMs can generate various types of content based on input prompts, failing to handle these outputs securely can introduce risks like cross-site scripting (XSS), server-side request forgery (SSRF), or even remote code execution (RCE). Unlike Overreliance (LLM09), which focuses on the accuracy of LLM outputs, Insecure Output Handling specifically addresses vulnerabilities in how these outputs are processed downstream.
As an example, there could be a web application that uses an LLM to summarize user-provided content and renders it back in a webpage. An attacker submits a prompt containing malicious JavaScript code. If the LLM’s output is displayed on the webpage without proper sanitization, the JavaScript will execute in the user’s browser, leading to XSS. Alternatively, if the LLM’s output is sent to a backend database or shell command, it could allow SQL injection or remote code execution if not properly validated.
How to prevent Insecure Output Handling:
- Zero-Trust Approach: Treat the LLM as an untrusted source, applying strict allow list validation and sanitization to all outputs it generates, especially before passing them to downstream systems or functions.
- Output Encoding: Encode LLM outputs before displaying them to end users, particularly when dealing with web content where XSS risks are prevalent.
- Adhere to Security Standards: Follow the OWASP Application Security Verification Standard (ASVS) guidelines, which provide strategies for input validation and sanitization to protect against code injection risks.
LLM03: Training Data Poisoning
Training Data Poisoning refers to the manipulation of the data used to train LLMs, introducing biases, backdoors, or vulnerabilities. This tampered data can degrade the model's effectiveness, introduce harmful biases, or create security flaws that malicious actors can exploit. Poisoned data could lead to inaccurate or inappropriate outputs, compromising user trust, harming brand reputation, and increasing security risks like downstream exploitation.
As an example, there could be a scenario where an LLM is trained on a dataset that has been tampered with by a malicious actor. The poisoned dataset includes subtly manipulated content, such as biased news articles or fabricated facts. When the model is deployed, it may output biased information or incorrect details based on the poisoned data. This not only degrades the model’s performance but can also mislead users, potentially harming the model’s credibility and the organization’s reputation.
How to prevent Training Data Poisoning:
- Data Validation and Vetting: Verify the sources of training data, especially when sourcing from third-party datasets. Conduct thorough checks on data integrity, and where possible, use trusted data sources.
- Machine Learning Bill of Materials (ML-BOM): Maintain an ML-BOM to track the provenance of training data and ensure that each source is legitimate and suitable for the model’s purpose.
- Sandboxing and Network Controls: Restrict access to external data sources and use network controls to prevent unintended data scraping during training. This helps ensure that only vetted data is used for training.
- Adversarial Robustness Techniques: Implement strategies like federated learning and statistical outlier detection to reduce the impact of poisoned data. Periodic testing and monitoring can identify unusual model behaviors that may indicate a poisoning attempt.
- Human Review and Auditing: Regularly audit model outputs and use a human-in-the-loop approach to validate outputs, especially for sensitive applications. This added layer of scrutiny can catch potential issues early.
LLM04: Model Denial of Service
Model Denial of Service (DoS) is a vulnerability in which an attacker deliberately consumes an excessive amount of computational resources by interacting with a LLM. This can result in degraded service quality, increased costs, or even system crashes. One emerging concern is manipulating the context window of the LLM, which refers to the maximum amount of text the model can process at once. This makes it possible to overwhelm the LLM by exceeding or exploiting this limit, leading to resource exhaustion.
As an example, an attacker may continuously flood the LLM with sequential inputs that each reach the upper limit of the model’s context window. This high-volume, resource-intensive traffic overloads the system, resulting in slower response times and even denial of service. As another example, if an LLM-based chatbot is inundated with a flood of recursive or exceptionally long prompts, it can strain computational resources, causing system crashes or significant delays for other users.
How to prevent Model Denial of Service:
- Rate Limiting: Implement rate limits to restrict the number of requests from a single user or IP address within a specific timeframe. This reduces the chance of overwhelming the system with excessive traffic.
- Resource Allocation Caps: Set caps on resource usage per request to ensure that complex or high-resource requests do not consume excessive CPU or memory. This helps prevent resource exhaustion.
- Input Size Restrictions: Limit input size according to the LLM's context window capacity to prevent excessive context expansion. For example, inputs exceeding a predefined character limit can be truncated or rejected.
- Monitoring and Alerts: Continuously monitor resource utilization and establish alerts for unusual spikes, which may indicate a DoS attempt. This allows for proactive threat detection and response.
- Developer Awareness and Training: Educate developers about DoS vulnerabilities in LLMs and establish guidelines for secure model deployment. Understanding these risks enables teams to implement preventative measures more effectively.
LLM05: Supply Chain Vulnerabilities
Supply Chain attacks are incredibly common and this is no different with LLMs, which, in this case refers to risks associated with the third-party components, training data, pre-trained models, and deployment platforms used within LLMs. These vulnerabilities can arise from outdated libraries, tampered models, and even compromised data sources, impacting the security and reliability of the entire application. Unlike traditional software supply chain risks, LLM supply chain vulnerabilities extend to the models and datasets themselves, which may be manipulated to include biases, backdoors, or malware that compromises system integrity.
As an example, an organization uses a third-party pre-trained model to conduct economic analysis. If this model is poisoned with incorrect or biased data, it could generate inaccurate results that mislead decision-making. Additionally, if the organization uses an outdated plugin or compromised library, an attacker could exploit this vulnerability to gain unauthorized access or tamper with sensitive information. Such vulnerabilities can result in significant security breaches, financial loss, or reputational damage.
How to prevent Supply Chain Vulnerabilities:
- Vet Third-Party Components: Carefully review the terms, privacy policies, and security measures of all third-party model providers, data sources, and plugins. Use only trusted suppliers and ensure they have robust security protocols in place.
- Maintain a Software Bill of Materials (SBOM): An SBOM provides a complete inventory of all components, allowing for quick detection of vulnerabilities and unauthorized changes. Ensure that all components are up-to-date and apply patches as needed.
- Use Model and Code Signing: For models and external code, employ digital signatures to verify their integrity and authenticity before use. This helps ensure that no tampering has occurred.
- Anomaly Detection and Robustness Testing: Conduct adversarial robustness tests and anomaly detection on models and data to catch signs of tampering or data poisoning. Integrating these checks into your MLOps pipeline can enhance overall security.
- Implement Monitoring and Patching Policies: Regularly monitor component usage, scan for vulnerabilities, and patch outdated components. For sensitive applications, continuously audit your suppliers’ security posture and update components as new threats emerge.
LLM06: Sensitive Information Disclosure
Sensitive Information Disclosure in LLMs occurs when the model inadvertently reveals private, proprietary, or confidential information through its output. This can happen due to the model being trained on sensitive data or because it memorizes and later reproduces private information. Such disclosures can result in significant security breaches, including unauthorized access to personal data, intellectual property leaks, and violations of privacy laws.
As an example, there could be an LLM-based chatbot trained on a dataset containing personal information such as users’ full names, addresses, or proprietary business data. If the model memorizes this data, it could accidentally reveal this sensitive information to other users. For instance, a user might ask the chatbot for a recommendation, and the model could inadvertently respond with personal information it learned during training, violating privacy rules.
How to prevent Sensitive Information Disclosure:
- Data Sanitization: Before training, scrub datasets of personal or sensitive information. Use techniques like anonymization and redaction to ensure no sensitive data remains in the training data.
- Input and Output Filtering: Implement robust input validation and sanitization to prevent sensitive data from entering the model’s training data or being echoed back in outputs.
- Limit Training Data Exposure: Apply the principle of least privilege by restricting sensitive data from being part of the training dataset. Fine-tune the model with only the data necessary for its task, and ensure high-privilege data is not accessible to lower-privilege users.
- User Awareness: Make users aware of how their data is processed by providing clear Terms of Use and offering opt-out options for having their data used in model training.
- Access Controls: Apply strict access control to external data sources used by the LLM, ensuring that sensitive information is handled securely throughout the system
LLM07: Insecure Plugin Design
Insecure Plugin Design vulnerabilities arise when LLM plugins, which extend the model’s capabilities, are not adequately secured. These plugins often allow free-text inputs and may lack proper input validation and access controls. When enabled, plugins can execute various tasks based on the LLM’s outputs without further checks, which can expose the system to risks like data exfiltration, remote code execution, and privilege escalation. This vulnerability is particularly dangerous because plugins can operate with elevated permissions while assuming that user inputs are trustworthy.
As an example, there could be a weather plugin that allows users to input a base URL and query. An attacker could craft a malicious input that directs the LLM to a domain they control, allowing them to inject harmful content into the system. Similarly, a plugin that accepts SQL “WHERE” clauses without validation could enable an attacker to execute SQL injection attacks, gaining unauthorized access to data in a database.
How to prevent Insecure Plugin Design:
- Enforce Parameterized Input: Plugins should restrict inputs to specific parameters and avoid free-form text wherever possible. This can prevent injection attacks and other exploits.
- Input Validation and Sanitization: Plugins should include robust validation on all inputs. Using Static Application Security Testing (SAST) and Dynamic Application Security Testing (DAST) can help identify vulnerabilities during development.
- Access Control: Follow the principle of least privilege, limiting each plugin's permissions to only what is necessary. Implement OAuth2 or API keys to control access and ensure only authorized users or components can trigger sensitive actions.
- Manual Authorization for Sensitive Actions: For actions that could impact user security, such as transferring files or accessing private repositories, require explicit user confirmation.
- Adhere to OWASP API Security Guidelines: Since plugins often function as REST APIs, apply best practices from the OWASP API Security Top 10. This includes securing endpoints and applying rate limiting to mitigate potential abuse.
LLM08: Excessive Agency
Excessive Agency in LLM-based applications arises when models are granted too much autonomy or functionality, allowing them to perform actions beyond their intended scope. This vulnerability occurs when an LLM agent has access to functions that are unnecessary for its purpose or operates with excessive permissions, such as being able to modify or delete records instead of only reading them. Unlike Insecure Output Handling, which deals with the lack of validation on the model’s outputs, Excessive Agency pertains to the risks involved when an LLM takes actions without proper authorization, potentially leading to confidentiality, integrity, and availability issues.
As an example, there could be an LLM-based assistant that is given access to a user's email account to summarize incoming messages. If the plugin that is used to read emails also has permissions to send messages, a malicious prompt injection could trick the LLM into sending unauthorized emails (or spam) from the user's account.
How to prevent Excessive Agency:
- Restrict Plugin Functionality: Ensure plugins and tools only provide necessary functions. For example, if a plugin is used to read emails, it should not include capabilities to delete or send emails.
- Limit Permissions: Follow the principle of least privilege by restricting plugins’ access to external systems. For instance, a plugin for database access should be read-only if writing or modifying data is not required.
- Avoid Open-Ended Functions: Avoid functions like “run shell command” or “fetch URL” that provide broad system access. Instead, use plugins that perform specific, controlled tasks.
- User Authorization and Scope Tracking: Require plugins to execute actions within the context of a specific user's permissions. For example, using OAuth with limited scopes helps ensure actions align with the user’s access level.
- Human-in-the-Loop Control: Require user confirmation for high-impact actions. For instance, a plugin that posts to social media should require the user to review and approve the content before it is published.
- Authorization in Downstream Systems: Implement authorization checks in downstream systems that validate each request against security policies. This prevents the LLM from making unauthorized changes directly.
LLM09: Overreliance
Overreliance occurs when users or systems trust the outputs of a LLM without proper oversight or verification. While LLMs can generate creative and informative content, they are prone to “hallucinations” (producing false or misleading information) or providing authoritative-sounding but incorrect outputs. Overreliance on these models can result in security risks, misinformation, miscommunication, and even legal issues, especially if LLM-generated content is used without validation. This vulnerability becomes especially dangerous in cases where LLMs suggest insecure coding practices or flawed recommendations.
As an example, there could be a development team using an LLM to expedite the coding process. The LLM suggests an insecure code library, and the team, trusting the LLM, incorporates it into their software without review. This introduces a serious vulnerability. As another example, a news organization might use an LLM to generate articles, but if they don’t validate the information, it could lead to the spread of disinformation.
How to prevent Overreliance:
- Regular Monitoring and Review: Implement processes to review LLM outputs regularly. Use techniques like self-consistency checks or voting mechanisms to compare multiple model responses and filter out inconsistencies.
- Cross-Verification: Compare the LLM’s output with reliable, trusted sources to ensure the information’s accuracy. This step is crucial, especially in fields where factual accuracy is imperative.
- Fine-Tuning and Prompt Engineering: Fine-tune models for specific tasks or domains to reduce hallucinations. Techniques like parameter-efficient tuning (PET) and chain-of-thought prompting can help improve the quality of LLM outputs.
- Automated Validation: Use automated validation tools to cross-check generated outputs against known facts or data, adding an extra layer of security.
- Risk Communication: Clearly communicate the limitations of LLMs to users, highlighting the potential for errors. Transparent disclaimers can help manage user expectations and encourage cautious use of LLM outputs.
- Secure Coding Practices: For development environments, establish guidelines to prevent the integration of potentially insecure code. Avoid relying solely on LLM-generated code without thorough review.
LLM10: Model Theft
Model Theft refers to the unauthorized access, extraction, or replication of proprietary LLMs by malicious actors. These models, containing valuable intellectual property, are at risk of exfiltration, which can lead to significant economic and reputational loss, erosion of competitive advantage, and unauthorized access to sensitive information encoded within the model. Attackers may steal models directly from company infrastructure or replicate them by querying APIs to build shadow models that mimic the original. As LLMs become more prevalent, safeguarding their confidentiality and integrity is crucial.
As an example, an attacker could exploit a misconfiguration in a company’s network security settings, gaining access to their LLM model repository. Once inside, the attacker could exfiltrate the proprietary model and use it to build a competing service. Alternatively, an insider may leak model artifacts, allowing adversaries to launch gray box adversarial attacks or fine-tune their own models with stolen data.
How to prevent Model Theft:
- Access Controls and Authentication: Use Role-Based Access Control (RBAC) and enforce strong authentication mechanisms to limit unauthorized access to LLM repositories and training environments. Adhere to the principle of least privilege for all user accounts.
- Supplier and Dependency Management: Monitor and verify the security of suppliers and dependencies to reduce the risk of supply chain attacks, ensuring that third-party components are secure.
- Centralized Model Inventory: Maintain a central ML Model Registry with access controls, logging, and authentication for all production models. This can aid in governance, compliance, and prompt detection of unauthorized activities.
- Network Restrictions: Limit LLM access to internal services, APIs, and network resources. This reduces the attack surface for side-channel attacks or unauthorized model access.
- Continuous Monitoring and Logging: Regularly monitor access logs for unusual activity and promptly address any unauthorized access. Automated governance workflows can also help streamline access and deployment controls.
- Adversarial Robustness: Implement adversarial robustness training to help detect extraction queries and defend against side-channel attacks. Rate-limit API calls to further protect against data exfiltration.
- Watermarking Techniques: Embed unique watermarks within the model to track unauthorized copies or detect theft during the model’s lifecycle.
Wrapping it all up
As LLMs continue to grow in capability and integration across industries, their security risks must be managed with the same vigilance as any other critical system. From Prompt Injection to Model Theft, the vulnerabilities outlined in the OWASP Top 10 for LLMs highlight the unique challenges posed by these models, particularly when they are granted excessive agency or have access to sensitive data. Addressing these risks requires a multifaceted approach involving strict access controls, robust validation processes, continuous monitoring, and proactive governance.
For technical leadership, this means ensuring that development and operational teams implement best practices across the LLM lifecycle starting from securing training data to ensuring safe interaction between LLMs and external systems through plugins and APIs. Prioritizing security frameworks such as the OWASP ASVS, adopting MLOps best practices, and maintaining vigilance over supply chains and insider threats are key steps to safeguarding LLM deployments. Ultimately, strong leadership that emphasizes security-first practices will protect both intellectual property and organizational integrity, while fostering trust in the use of AI technologies.