In this post, I'm going to share a short script which can be used to create you own TFTP server. You need to run the server.py on your server (it can be your local machine) and client.py on your client machine (i.e. your local machine). While both scripts are running, you can look at your server's files and download them.CODE: CLIENT.PY
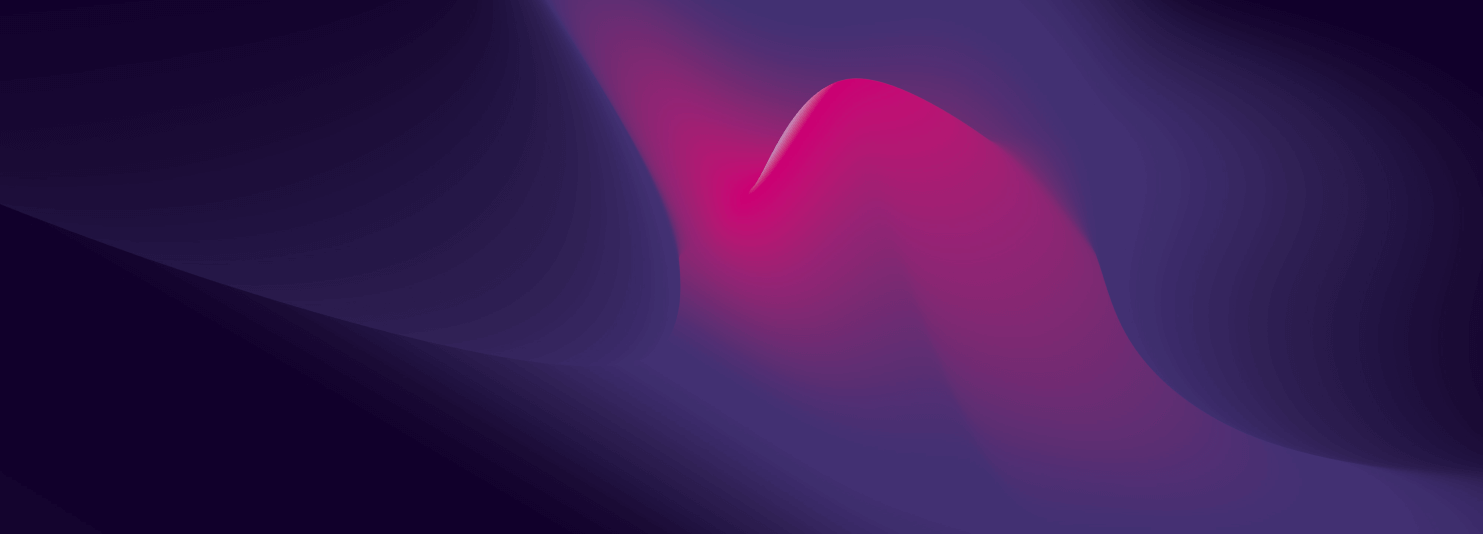
import socketdef main():sock=socket.socket(socket.AFINET,socket.SOCKDGRAM)print 'Enter the client IP address i.e. 192.168.1.102',print 'If server is your Local computer then you can enter 127.0.0.1'while True:ip=rawinput("Server's IP address: ")try:sock.connect((ip,5555))breakexcept:print '...Enter a valid IP address...'help="Enter:nt'ls' for listnt'cwd' for current working directorynt'cd <directory name>' to change directorynt'download <file name>' to download a filent'quit' to Exit"print helpwhile True:cmd=rawinput('Enter a command: ')if cmd=='quit':sock.send('quit')breakelif cmd=='ls' or cmd=='cwd' or (cmd[:2]=='cd' and cmd[2:3]==' ' and cmd[3:]!=''):sock.send(cmd)data=sock.recv(8096)print dataelif (cmd[:8]=='download' and cmd[8:9]==' ' and cmd[9:]!=''):sock.send(cmd)data=sock.recv(8096)if data=='fail':print '...file not found...'else:filename='downloaded'+cmd[9:]fo=open(filename,'ab')print '...downloading file Please wait...'while data!='done':fo.write(data)data=sock.recv(8096)fo.close()print '...file downloaded...'else:print helpmain()
SERVER.PY import socketimport subprocessimport osdef main():sock=socket.socket(socket.AFINET,socket.SOCKDGRAM)sock.bind(('',5555))while True:cmd,addr=sock.recvfrom(2048)if cmd=='quit':breakelif cmd=='cwd':sock.sendto(os.getcwd(),addr)elif cmd[:2]=='cd' and cmd[2:3]==' ' and cmd[3:]!='':try:dir=cmd[3:]os.chdir(os.path.join(os.getcwd(),dir))sock.sendto('directory changed',addr)except:sock.sendto('fail to change directory',addr)elif cmd=='ls':process=subprocess.Popen('dir',shell=True,stdout=subprocess.PIPE)result=process.stdout.read()+' 'sock.sendto(result,addr)elif cmd[:8]=='download' and cmd[8:9]==' ' and cmd[9:]!='':filename=cmd[9:]try:size=8096fo=open(file_name,'rb')data=fo.read(size)while data!='':sock.sendto(data,addr)data=fo.read(size)fo.close()sock.sendto('done',addr)except:sock.sendto('fail',addr)else:passmain()

Building a Security Team
June 27, 2023
Digital Forensics and Incident Response: What It Is, When You Need It, and How to Implement It
A quick guide to digital forensics and incident response (DFIR): what it is, when it’s needed, how to implement a cutting-edge program, and how to develop DFIR skills on your team.